How to Create Input With Only Numbers in React
- Use Number Pattern Method in React
-
Use Input
type='tel'
Method in React - Use Incremental Number Method in React
- Conclusion
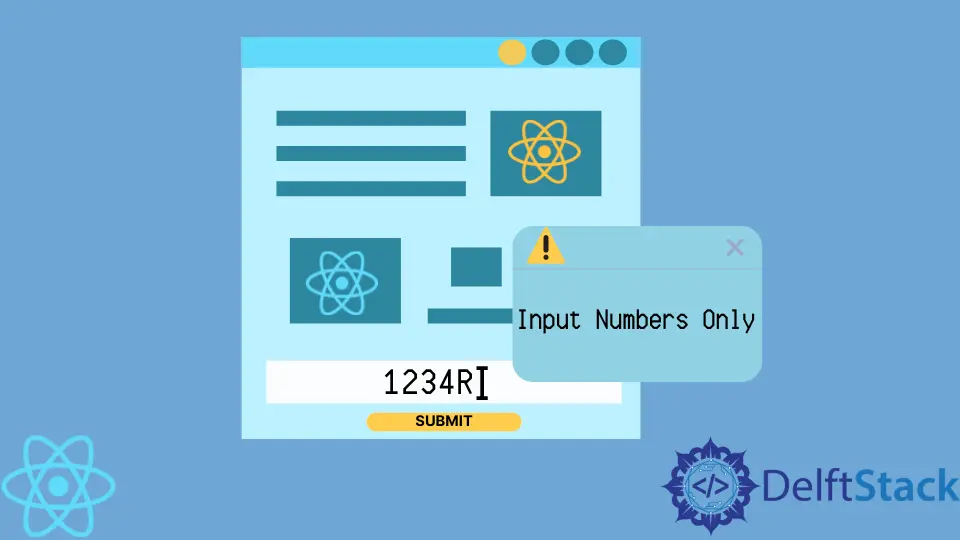
For e-commerce websites, where users are expected to type in amounts, it is an ideal approach to ensure that users are allowed to type in numbers only. This is also true for form-filling websites, cash applications, banking applications, etc.
There are numerous approaches to applying this feature to a web application. Lets us look at just a number of them.
Use Number Pattern Method in React
This method takes quite a reversed approach. It takes input type to be text, which should normally take letters, but the magic is setting the pattern to only digits.
In our project folder, we navigate to the index.js
file and put the following code:
Code Snippet - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Example extends React.Component {
constructor(props) {
super(props);
this.state = {
financialGoal: ''
}
}
handleChange(evt) {
const financialGoal = (evt.target.validity.valid) ? evt.target.value : this.state.financialGoal;
this.setState({ financialGoal });
}
render() {
return (
<input type="text" pattern="[0-9]*" onInput={this.handleChange.bind(this)} value={this.state.financialGoal} />
)
}
}
ReactDOM.render(<Example />, document.body);
First, set the initial state of the component to ' '
, so the input bar is empty, and then we bind the component to the handleChange
event listener to validate that the input is a number only. So when we input characters into the input bar, the event listener kicks in to check that what is inputted is as required.
Output:
Use Input type='tel'
Method in React
The 'tel'
type input method is usually adopted when users are required to input their telephone number in the required field. But this approach is also applicable when we want customers to input mere numbers and we need to add a pattern with certain parameters.
Code Snippet - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Input extends React.Component {
state = {message: ''};
updateNumber = (e) => {
const val = e.target.value;
if (e.target.validity.valid) this.setState({message: e.target.value});
else if (val === '' || val === '-') this.setState({message: val});
}
render() {
return (
<input
type='tel'
value={this.state.message}
onChange={this.updateNumber}
pattern="^-?[0-9]\d*\.?\d*$"
/>
);
}
}
ReactDOM.render(<Input />, document.getElementById('main'));
We assign the updateNumber
component to the onChange
event listener, such that when characters are typed into the input field. The event listener gets triggered and then runs the target validity function to make sure that what is typed in the input field are numbers only.
Output:
Use Incremental Number Method in React
This example allows users to only pick numbers by using the up or down arrows at the extreme of the input bar to increase or decrease the numbers. This method works great for web forms where the user wants to pick a date or for online polling situations.
Code Snippet - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const {Component} = React;
class Input extends Component {
onKeyPress(event) {
const keyCode = event.keyCode || event.which;
const keyValue = String.fromCharCode(keyCode);
if (/\+|-/.test(keyValue))
event.preventDefault();
}
render() {
return (
<input style={{width: '150px'}} type="number" onKeyPress={this.onKeyPress.bind(this)} />
)
}
ReactDOM.render(<Input /> , document.body);
The onKeyPress
event handler is used alongside keyCode
to check the keys the user is pressing.
Output:
Conclusion
The idea of making input field numbers is really helpful for customers. It eliminates the confusion of whether they could input other characters in the input field.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn