在 React 中只輸入數字
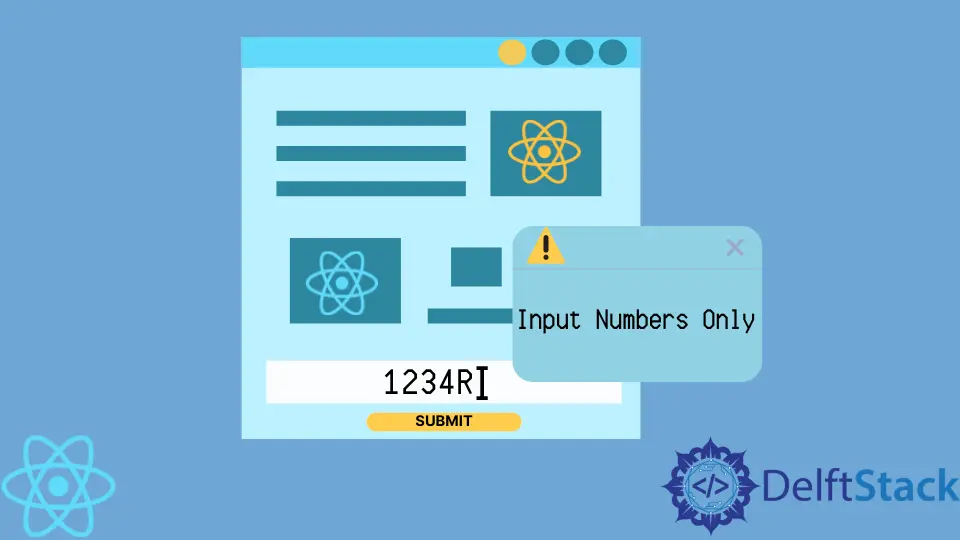
對於希望使用者輸入金額的電子商務網站,這是確保使用者只允許輸入數字的理想方法。這也適用於填表網站、現金應用程式、銀行應用程式等。
有許多方法可以將此功能應用於 Web 應用程式。讓我們看看其中的一些。
在 React 中使用數字模式方法
這種方法採用了一種完全相反的方法。它的輸入型別是文字,通常應該是字母,但神奇的是把模式設定為只有數字。
在我們的專案資料夾中,我們導航到 index.js
檔案並輸入以下程式碼:
程式碼片段 - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Example extends React.Component {
constructor(props) {
super(props);
this.state = {
financialGoal: ''
}
}
handleChange(evt) {
const financialGoal = (evt.target.validity.valid) ? evt.target.value : this.state.financialGoal;
this.setState({ financialGoal });
}
render() {
return (
<input type="text" pattern="[0-9]*" onInput={this.handleChange.bind(this)} value={this.state.financialGoal} />
)
}
}
ReactDOM.render(<Example />, document.body);
首先,將元件的初始狀態設定為' '
,因此輸入欄為空,然後我們將元件繫結到 handleChange
事件偵聽器以驗證輸入是否僅為數字。因此,當我們在輸入欄中輸入字元時,事件偵聽器會啟動以檢查輸入的內容是否符合要求。
輸出:
在 React 中使用輸入 type='tel'
方法
當使用者需要在必填欄位中輸入他們的電話號碼時,通常採用'tel'
型別的輸入法。但這種方法也適用於我們希望客戶僅輸入數字並且需要新增具有某些引數的模式的情況。
程式碼片段 - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Input extends React.Component {
state = {message: ''};
updateNumber = (e) => {
const val = e.target.value;
if (e.target.validity.valid) this.setState({message: e.target.value});
else if (val === '' || val === '-') this.setState({message: val});
}
render() {
return (
<input
type='tel'
value={this.state.message}
onChange={this.updateNumber}
pattern="^-?[0-9]\d*\.?\d*$"
/>
);
}
}
ReactDOM.render(<Input />, document.getElementById('main'));
我們將 updateNumber
元件分配給 onChange
事件偵聽器,以便在輸入欄位中輸入字元時。事件偵聽器被觸發,然後執行目標有效性函式以確保在輸入欄位中鍵入的內容僅為數字。
輸出:
在 React 中使用遞增數字方法
此示例僅允許使用者通過使用輸入欄末端的向上或向下箭頭來增加或減少數字來選擇數字。此方法非常適用於使用者想要選擇日期或線上投票情況的 Web 表單。
程式碼片段 - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const {Component} = React;
class Input extends Component {
onKeyPress(event) {
const keyCode = event.keyCode || event.which;
const keyValue = String.fromCharCode(keyCode);
if (/\+|-/.test(keyValue))
event.preventDefault();
}
render() {
return (
<input style={{width: '150px'}} type="number" onKeyPress={this.onKeyPress.bind(this)} />
)
}
ReactDOM.render(<Input /> , document.body);
onKeyPress
事件處理程式與 keyCode
一起使用以檢查使用者正在按下的鍵。
輸出:
まとめ
製作輸入欄位編號的想法對客戶非常有幫助。它消除了他們是否可以在輸入欄位中輸入其他字元的混淆。
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn