How to Set Focus on an Input Field After Rendering Using React
-
Use the
componentDidMount()
Method in React -
Use the
autoFocus
Prop Method in React - Use Inline Ref Property Method in React
- Conclusion
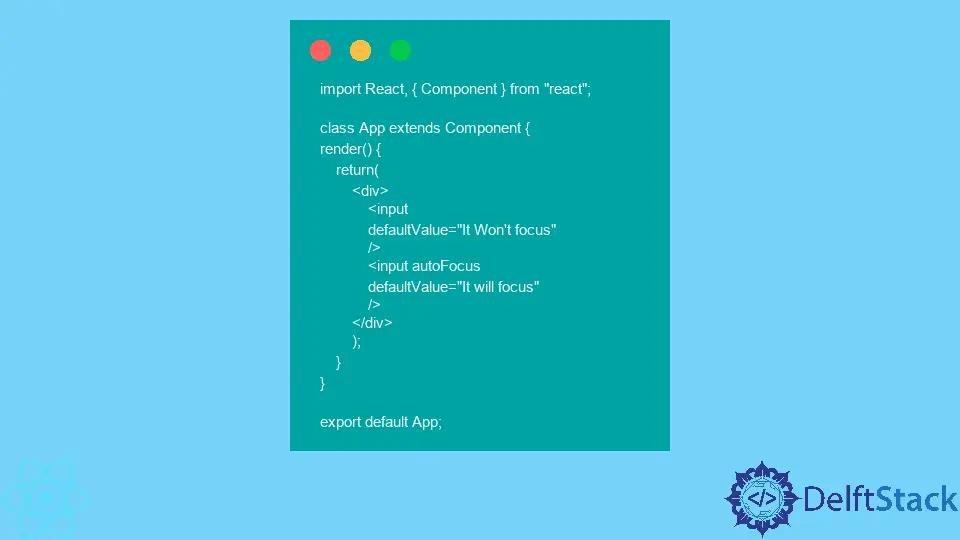
Input fields are those wide bars that we input information to be submitted to the website’s server. A popular example is google.com’s search bar.
This article will demonstrate different methods to focus on an input field after the react components have been rendered on the web page.
Use the componentDidMount()
Method in React
To begin our react project, create a new project using npx create-react-app exampleone
from the terminal, after which we navigate to the project name.
Applying the componentDidMount()
method enables the input field to focus after displaying the page. This function is good for making pages load faster as the function doesn’t load data until after the first-page rendering and is called once.
Code Snippet - App.js
:
import React, { Component } from "react";
class App extends Component {
componentDidMount() {
this.nameInput.focus();
}
render() {
return (
<div>
<input
defaultValue="It Won't focus"
/>
<input
ref={(input) => { this.nameInput = input; }}
defaultValue="It will focus"
/>
</div>
);
}
}
export default App;
Use the autoFocus
Prop Method in React
After the first time the page is rendered, the input field is automatically highlighted for the user. This method is ideal for a website with lots of content that wants its users to easily locate the input field once the page loads.
The developer needs to be mindful of this autoFocus
feature because the first one will get focused if these features are applied to multiple elements.
Code Snippet - App.js
:
import React, { Component } from "react";
class App extends Component {
render() {
return(
<div>
<input
defaultValue="It Won't focus"
/>
<input autoFocus
defaultValue="It will focus"
/>
</div>
);
}
}
export default App;
Use Inline Ref Property Method in React
We have to write the focus function inside the render component using this method. This method is the most convenient as it is easy to code and easy to understand in situations where we are sharing our codes with another developer.
Code Snippet - App.js
:
import React, { Component } from "react";
class App extends Component {
render() {
return(
<div>
<input
defaultValue="It Won't focus"
/>
<input
ref={(input) => {input && input.focus() }}
defaultValue="It will focus"
/>
</div>
);
}
}
export default App;
When we are done with each method, we will run the application using npm start
.
Output:
Conclusion
The focus effect in React is a way to help users achieve a smooth and less cumbersome browsing experience, as it makes the input more obvious to the eye while adding some beauty to the webpage.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn