How to Remove Object Names and Dimnames in R
-
Use the
unname()
Function to Remove Object Names and Dimnames in R - Use a Function Argument When Available to Remove Object Names and Dimnames in R
-
Use the
as.vector()
Function to Remove Object Names and Dimnames in R - Set Names and Dimnames to NULL to Remove Object Names and Dimnames in R
- Row Names in Data Frames in R
- Conclusion
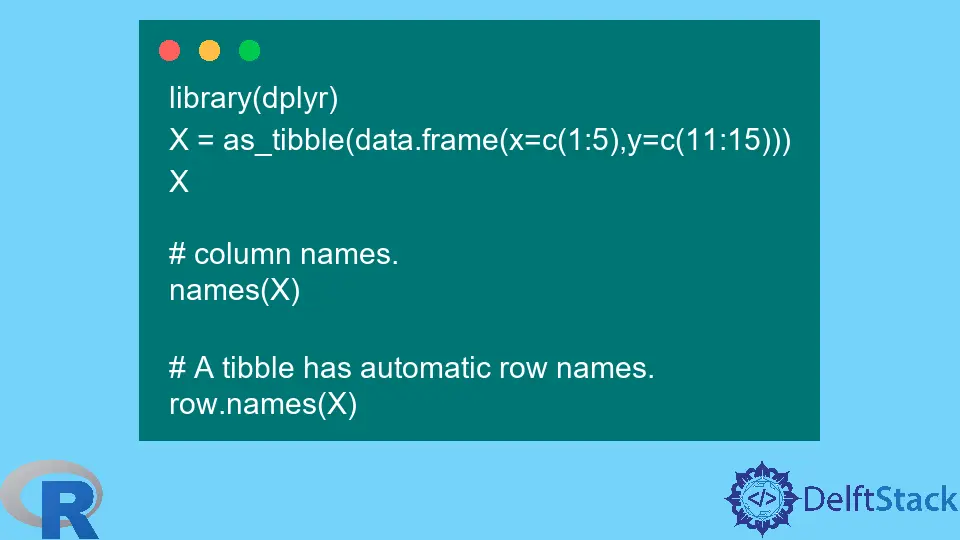
R gives us options to remove names and dimnames when we only want the values. We will demonstrate some of these options in this article.
Use the unname()
Function to Remove Object Names and Dimnames in R
The unname()
function removes names and dimnames from objects.
Example code:
# Calculate the 25th percentile.
a = quantile(c(2, 4, 6, 8), probs=0.25)
a # Displays the name.
names(a) # 25% is the name.
# We can remove the name by using the unname() function.
unname(a)
Output:
> # Calculate the 25th percentile.
> a = quantile(c(2, 4, 6, 8), probs=0.25)
> a # Displays the name.
25%
3.5
>
> names(a) # 25% is the name.
[1] "25%"
>
> # We can remove the name by using the unname() function.
> unname(a)
[1] 3.5
Use a Function Argument When Available to Remove Object Names and Dimnames in R
Some functions allow us to create objects without names. The function signature will tell us whether we can skip its creation.
Using such a function, we can directly create objects without names. Again, we will take quantile()
because it has the names
argument.
Example Code:
# Calculate the 60th percentile. Use names = FALSE.
b = quantile(c(2, 4, 6, 8), probs=0.6, names = FALSE)
b # There is no name
names(b)
Output:
> # Calculate the 60th percentile. Use names = FALSE.
> b = quantile(c(2, 4, 6, 8), probs=0.6, names = FALSE)
> b # There is no name
[1] 5.6
> names(b)
NULL
Use the as.vector()
Function to Remove Object Names and Dimnames in R
Section 5.9 of the manual An Introduction to R states that arrays can be coerced to a simple vector object using as.vector()
. This action removes the names.
Example Code:
# The summary has meaningful names.
c = summary(c(2, 4, 6, 8))
c
# This is just for illustration of the technique.
# We can remove the names using the as.vector() function.
as.vector(c)
Output:
> # The summary has meaningful names.
> c = summary(c(2, 4, 6, 8))
> c
Min. 1st Qu. Median Mean 3rd Qu. Max.
2.0 3.5 5.0 5.0 6.5 8.0
> # This is just for illustration of the technique.
> # We can remove the names using the as.vector() function.
> as.vector(c)
[1] 2.0 3.5 5.0 5.0 6.5 8.0
Set Names and Dimnames to NULL to Remove Object Names and Dimnames in R
Since names and dimnames can be assigned, we can set them to NULL.
Example Code:
# The summary has names.
d = summary(c(2, 4, 6, 8))
d
names(d)
# Set the names to NULL.
names(d) = NULL
# Now, there are no names.
d
names(d)
Output:
> # The summary has names.
> d = summary(c(2, 4, 6, 8))
> d
Min. 1st Qu. Median Mean 3rd Qu. Max.
2.0 3.5 5.0 5.0 6.5 8.0
> names(d)
[1] "Min." "1st Qu." "Median" "Mean" "3rd Qu." "Max."
>
> # Set the names to NULL.
> names(d) = NULL
>
> # Now there are no names.
> d
[1] 2.0 3.5 5.0 5.0 6.5 8.0
> names(d)
NULL
Row Names in Data Frames in R
We get two lists if we use dimnames()
on a data frame. One is names
, which gives the column names; the other is row.names
.
In R, data frames have row names. If we do not assign row names, R gives automatic row names.
Row names cannot be set to NULL. Doing so will make R give automatic row names.
The documentation states that the unname()
function does not work as expected when we use force = TRUE
. It throws an error, at least till R version 4.1.2.
The reason is that row names cannot be removed by setting them to NULL.
It does not work on dplyr’s tibbles either because they are data frames. Tibbles cannot be manually assigned row names, but they have them.
Example Code:
library(dplyr)
X = as_tibble(data.frame(x=c(1:5),y=c(11:15)))
X
# column names.
names(X)
# A tibble has automatic row names.
row.names(X)
Output:
> library(dplyr)
> X = as_tibble(data.frame(x=c(1:5),y=c(11:15)))
> X
# A tibble: 5 x 2
x y
<int> <int>
1 1 11
2 2 12
3 3 13
4 4 14
5 5 15
>
> # column names.
> names(X)
[1] "x" "y"
>
> # A tibble has automatic row names.
> row.names(X)
[1] "1" "2" "3" "4" "5"
Column names can be removed from data frames and tibbles using the unname()
function. However, we must remember that column names are helpful in many data analyses.
Conclusion
We saw a few different approaches to removing objects’ names and dimnames.
Depending on the objects we are working with, we can choose any convenient option to remove these attributes.