How to Identify R Object Properties
- Identify R Object Properties
-
str()
Function -
typeof()
Function -
class()
Function -
is.___()
Functions -
attributes()
Function - Other Functions
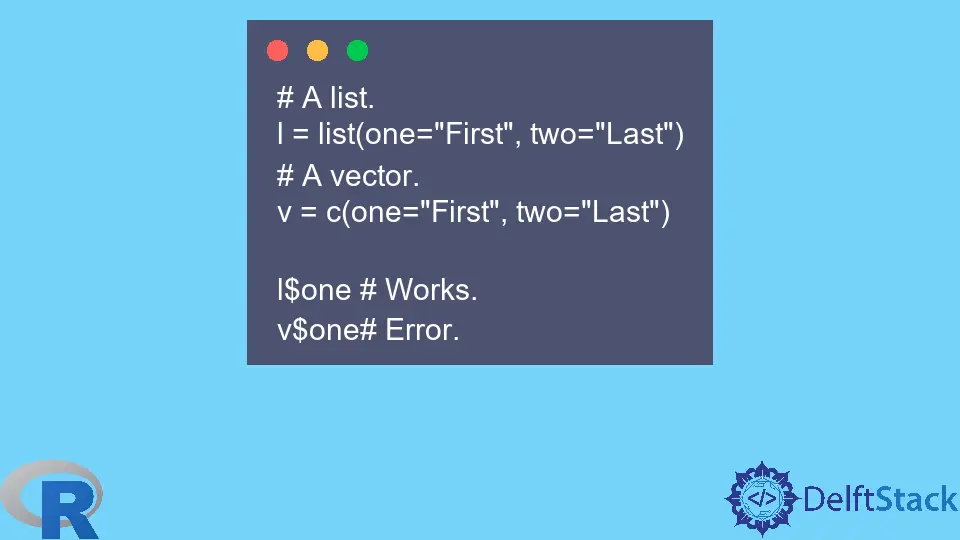
In our data analysis, we may encounter a situation where a function or operator that we use works on some objects but not on others. This happens because the function/operator only works on objects of a certain type.
For example, the $
operator works on a list but not an atomic vector.
Example Code:
# A list.
l = list(one="First", two="Last")
# A vector.
v = c(one="First", two="Last")
l$one # Works.
v$one# Error.
Output:
> l$one # Works.
[1] "First"
> v$one# Error.
Error in v$one : $ operator is invalid for atomic vectors
Identify R Object Properties
This article will look at functions that help us identify various properties of the objects we work with.
This article will only give a brief description and an example for each function. R’s documentation for these functions gives more details and examples.
First, we will create some R objects.
Example Code:
# Vector of numbers.
vn = c(4, 5, 6, 7)
# Vector of Booleans. (A vector of True False values)
vb <- vn%%2 == 0
# Data frame.
dfr = data.frame(Col1=vn, Col2=vb)
str()
Function
The str()
function displays the object’s internal structure. It works on any R object.
Example Code:
str(vn)
str(vb)
str(dfr)
Output:
> str(vn)
num [1:4] 4 5 6 7
> str(vb)
logi [1:4] TRUE FALSE TRUE FALSE
> str(dfr)
'data.frame': 4 obs. of 2 variables:
$ Col1: num 4 5 6 7
$ Col2: logi TRUE FALSE TRUE FALSE
typeof()
Function
The typeof()
function displays any R object’s type or storage mode.
Example Code:
typeof(vn)
typeof(vb)
typeof(dfr)
Output:
> typeof(vn)
[1] "double"
> typeof(vb)
[1] "logical"
> typeof(dfr)
[1] "list"
class()
Function
The class()
function displays the class
attribute of the R object. It lists the names of the classes from which this object inherits.
Even objects that do not have a class attribute have an implicit class.
Example Code:
class(vb)
class(dfr)
# Create a matrix and check its class.
m = matrix(c(1:6), nrow=3)
class(m)
Output:
> class(vb)
[1] "logical"
> class(dfr)
[1] "data.frame"
> class(m)
[1] "matrix" "array"
is.___()
Functions
R has many inbuilt is.___()
functions. These functions return Boolean values indicating whether the object is of that type.
For example, we can check whether a given object is a vector, a list, a matrix, an array, a data frame, etc. In the following code, we will see the result of checking a particular object with various is.___()
functions.
Example Code:
# We will check whether the object vb we created is of any of the following types of object.
is.list(vb)
is.vector(vb)
is.atomic(vb)
is.numeric(vb)
is.matrix(vb)
is.data.frame(vb)
is.array(vb)
Output:
> is.list(vb)
[1] FALSE
> is.vector(vb)
[1] TRUE
> is.atomic(vb)
[1] TRUE
> is.numeric(vb)
[1] FALSE
> is.matrix(vb)
[1] FALSE
> is.data.frame(vb)
[1] FALSE
> is.array(vb)
[1] FALSE
attributes()
Function
The attributes()
function displays a list of an object’s attributes.
Example Code:
# For the list object.
attributes(l)
# For the data frame object.
attributes(dfr)
# For the matrix object.
attributes(m)
Output:
> # For the list object.
> attributes(l)
$names
[1] "a" "z"
> # For the data frame object.
> attributes(dfr)
$names
[1] "Col1" "Col2"
$class
[1] "data.frame"
$row.names
[1] 1 2 3 4
> # For the matrix object.
> attributes(m)
$dim
[1] 3 2
Other Functions
Besides the functions described above, R has other functions which give us more information about an object. A few of them are specific to objects of certain types.
Some of the more commonly used functions are:
dim()
: displays the dimensions of an object.names()
: displays the names of the components of an object.summary()
: for statistical models, displays a summary of the model.colnames()
,rownames()
androw.names()
: display the column/row names for matrix-like objects. For a data frame,rownames()
callsrow.names()
andcolnames()
callsnames()
.