R Continue for Loop
-
Use the
next
Statement to Continue afor
Loop in R -
Use
next
With Multiple Conditions to Continue afor
Loop in R -
Use the
if
Statement to Skip to the Next Iteration in R -
Use the
break
Statement to Exit a Loop in R -
Use Vectorized Operations to Continue a
for
Loop in R - Conclusion
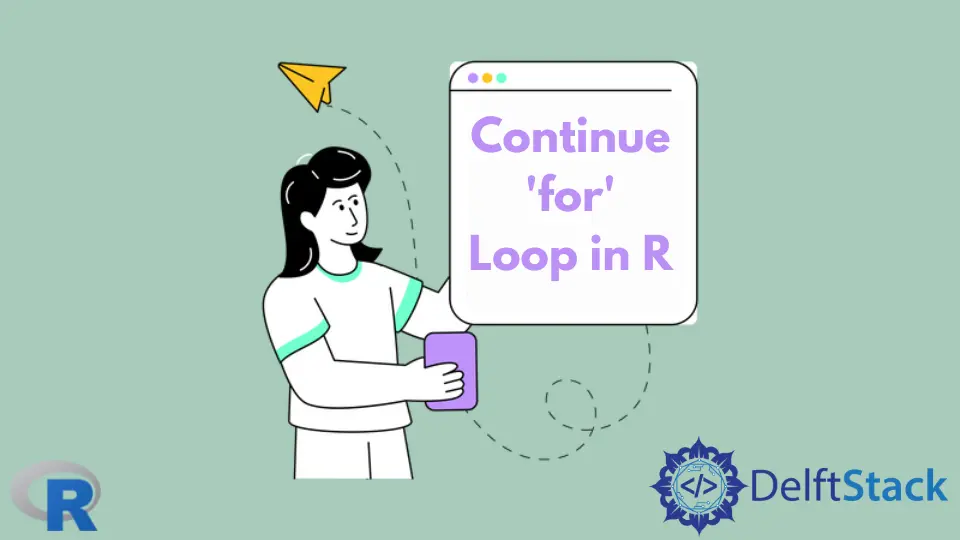
In R, the for
loop is a fundamental construct for iterating over a sequence of elements. Occasionally, you might encounter situations where you want to skip the current iteration and move to the next one prematurely.
In this article, you will learn how to skip lines of code and jump to the next iteration in a for
loop in R when some condition is met.
Use the next
Statement to Continue a for
Loop in R
When you have many lines of code inside a loop, and you want R to continue for the next iteration when some condition is met, you can write an if
clause that evaluates the condition, and if it is true, skip everything in the loop and continue for the next iteration. That skipping is done with the next
instruction.
The next
statement allows you to skip the rest of the code in the loop for the current iteration and move on to the next one.
A typical case is when you use a loop to go through a series of data elements and do some calculations on each one, leaving aside those that meet a particular condition. In the following example, we go through a vector of numbers, multiplying all of them except for those that are multiples of 5.
result <- 1
base <- 5
x <- c(7, 5, 3, 10, 8, 4, 11, 15, 6, 13)
for (num in x) {
if (num%%base == 0) {
next
}
result <- result * num
}
paste("Result:", result)
Output:
[1] "Result: 576576"
This code initializes variables, result
and base
, processes a sequence of numbers (x
), and calculates a cumulative result. The loop iterates through each number in x
, skipping those divisible by the base
.
The final result is obtained by multiplying the non-divisible numbers together. The output displays the calculated result after processing the entire sequence.
Note that the result is the multiplication of all the vector numbers except for 5, 10, and 15.
Use next
With Multiple Conditions to Continue a for
Loop in R
You can use next
with multiple conditions to skip iterations based on complex logic. The following example illustrates the use of next
with two conditions:
for (i in 1:5) {
if (i%%2 == 0 || i == 3) {
next # Skip even numbers and 3
}
print(i)
}
Output:
[1] 1
[1] 5
This code demonstrates the use of a for
loop to iterate over values from 1 to 5. Within the loop, an if
statement is employed to skip specific values during iteration.
The conditions for skipping include even numbers (i %% 2 == 0
) and the number 3 (i == 3
). The next
statement is utilized to skip the remaining code within the loop for iterations that meet these conditions.
Consequently, the code prints only the values 1 and 5 to the console, as they are not subject to the skipping conditions.
Use the if
Statement to Skip to the Next Iteration in R
You can use a simple if
statement to skip to the next iteration of the loop, controlling the flow of the loop based on a condition. This method combines the condition and the action in a single if
statement.
for (i in 1:5) {
if (i == 3) {
next # Skip iteration when i is 3
} else {
print(i)
}
}
Output:
[1] 1
[1] 2
[1] 4
[1] 5
The if
statement provides a flexible way to customize the behavior of your for
loop based on specific conditions. Incorporating if
statements empowers you to control the flow of your code dynamically, whether you want to skip certain iterations or exit the loop prematurely.
Use the break
Statement to Exit a Loop in R
While break
is traditionally used to exit a loop, combining it with conditional checks allows you to achieve a form of loop continuation. By strategically placing the break
statement, you can skip the remaining code and move to the next iteration based on specific conditions.
for (i in 1:5) {
if (i == 3) {
break # Exit the loop when i is 3
}
print(i)
}
Output:
[1] 1
[1] 2
The break
statement is a useful tool when you want to exit a loop based on a specific condition. It allows you to control the flow of the loop and terminate it before it completes all iterations.
In this example, the loop exits when i
is equal to 3, demonstrating how the break
statement can be used for early termination.
Use Vectorized Operations to Continue a for
Loop in R
Leveraging vectorized operations and logical indexing is a powerful way to skip specific elements within a loop, avoiding the need for explicit next
or break
statements. In R, it’s often more efficient to use vectorized operations instead of explicit loops.
For instance, you can create a vector that contains specific elements to skip, like the following code:
# Using vectorized operations to skip specific values
to_skip <- c(2, 4, 6)
for (i in 1:6) {
if (i %in% to_skip) {
next # Skip values specified in 'to_skip'
}
print(i)
}
Output:
[1] 1
[1] 3
[1] 5
Here, the to_skip
vector contains values that should be skipped in the loop. The if (i %in% to_skip)
statement checks whether the current value of i
is in the vector.
If true
, the next
statement is executed, skipping the remaining code for that iteration.
Conclusion
This article delves into different methods for controlling the flow of for
loops in R using the next
statement. It explores techniques such as skipping iterations based on conditions, handling multiple conditions with next
, prematurely exiting loops with break
, and employing vectorized approaches for efficiency.
The examples provided demonstrate how these methods can be applied to enhance code flexibility and readability. Overall, understanding and incorporating these techniques empower R programmers to optimize loop execution based on specific requirements.