Nested for Loops in R
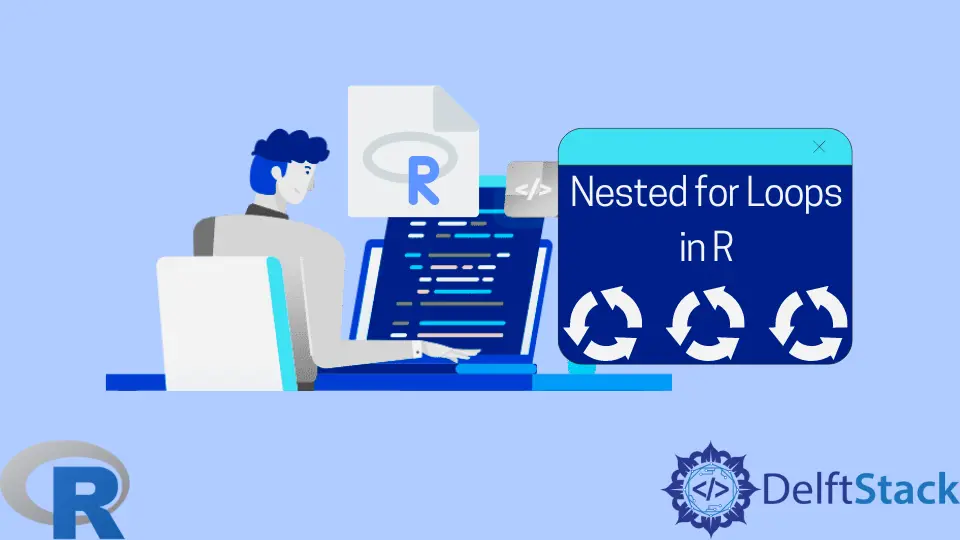
This article will introduce the nested for
loops in R.
for
Loop in R Language
The for
loop is available in R language with similar heuristics as in most programming languages. It repeats the given code block multiple times. The for
loop syntax is as follows.
for (item in set) {}
item
is an object that stores the iterated element from the set
. The for
loop doesn’t return output, so we need to call the print
function to output the word
value on each iteration.
vec1 <- c("ace", "spades", "king", "spades", "queen", "spades", "jack",
"spades", "ten", "spades")
for (word in vec1) {
print(word)
}
Output:
[1] "ace"
[1] "spades"
[1] "king"
[1] "spades"
[1] "queen"
[1] "spades"
[1] "jack"
[1] "spades"
[1] "ten"
[1] "spades"
We can also implement the for
loop, where the index is exposed as a variable. In this case, the length
function is utilized to calculate the size of the vec1
vector and iterate from the first element to the end. Note that 1:
notation is important, and it specifies the beginning of the range. The following example code creates a string vector copied to another vector of the same size using the for
loop.
vec1 <- c("ace", "spades", "king", "spades", "queen", "spades", "jack",
"spades", "ten", "spades")
vec2 <- vector(length = length(vec1))
for (i in 1:length(vec1)) {
vec2[i] <- vec1[i]
}
vec2
Output:
[1] "ace" "spades" "king" "spades" "queen" "spades" "jack" "spades" "ten"
[10] "spades"
Use Nested for
Loop to Iterate Over Matrix Elements in R
Nested loops can be implemented using the for
loop structure. This can be utilized to iterate over matrix elements and initialize them with random values. Note that the general notation is the same as the previous example, except that the end of the range is calculated with the nrow
and ncol
functions. nrow
and ncol
return the number of rows or columns of the array, respectively.
mat1 <- matrix(0, nrow = 5, ncol = 5)
for (i in 1:nrow(mat1)) {
for (j in 1:ncol(mat1)) {
mat1[i, j] <- sample(1:100, 1, replace=TRUE)
}
}
mat1
Output:
[,1] [,2] [,3] [,4] [,5]
[1,] 13 14 13 67 98
[2,] 28 50 23 55 9
[3,] 3 65 99 17 93
[4,] 18 6 20 50 46
[5,] 51 76 33 26 3
Although, the nested loop structure works fine in the previous example code. Matrix initialization is better done using the sample
function chained directly as the first argument of the matrix
function, as shown in the following snippet.
mat2 <- matrix(sample(1:100, 25, replace = TRUE), ncol = 5)
mat2
Output:
[,1] [,2] [,3] [,4] [,5]
[1,] 85 19 26 53 88
[2,] 44 50 66 96 56
[3,] 42 46 37 19 66
[4,] 43 23 13 32 67
[5,] 56 51 21 2 56
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook