How to Append Elements to a List in R
- Understanding Lists in R
-
Method 1: Using the
append()
Function - Method 2: Using List Indexing
- Method 3: Using a Loop to Append Multiple Elements
- Conclusion
- FAQ
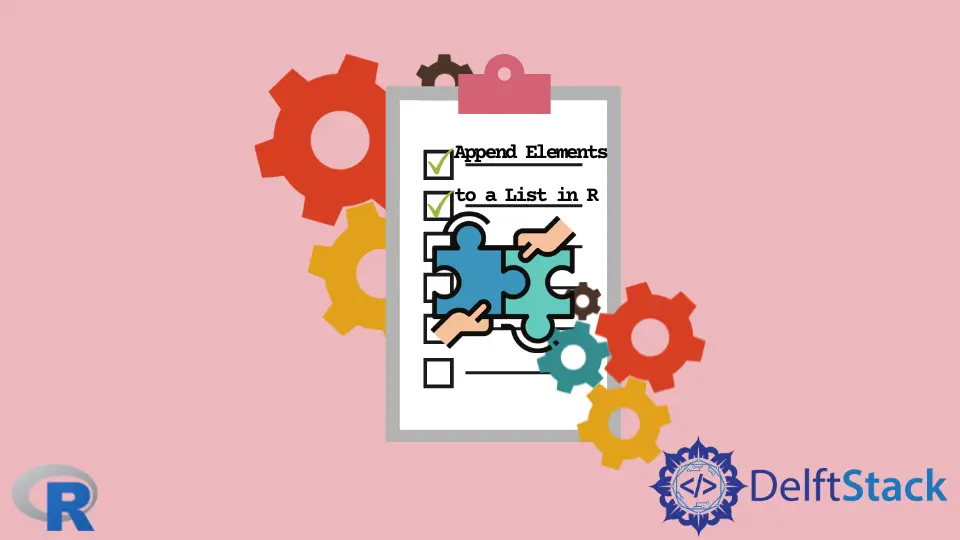
Appending elements to a list in R is a fundamental skill that can significantly enhance your data manipulation capabilities. Whether you’re working with statistical data, creating complex data structures, or simply organizing information, knowing how to efficiently add elements to a list is crucial.
In this tutorial, we will explore various methods to append to a list in R, focusing on using loops to iterate through elements. By the end, you will be equipped with the knowledge of the most efficient and convenient techniques for appending elements to lists in R.
Understanding Lists in R
Before diving into the methods of appending elements, it’s essential to understand what a list is in R. Lists are versatile data structures that can hold different types of elements, including vectors, matrices, and even other lists. They are particularly useful when you want to store heterogeneous data.
You can create a list in R using the list()
function. For example:
my_list <- list(name = "John", age = 30, scores = c(90, 85, 88))
This creates a list with three elements. Now, let’s explore how to append elements to this list effectively.
Method 1: Using the append()
Function
One of the simplest ways to append elements to a list in R is by using the built-in append()
function. This function allows you to add elements at a specified position or at the end of the list.
Here’s how you can use it:
my_list <- list(name = "John", age = 30, scores = c(90, 85, 88))
my_list <- append(my_list, list(city = "New York"))
print(my_list)
Output:
$name
[1] "John"
$age
[1] 30
$scores
[1] 90 85 88
$city
[1] "New York"
In this example, we first create a list called my_list
. We then use the append()
function to add a new element, city
, to the list. The append()
function returns a new list with the added element, which we assign back to my_list
. This method is straightforward and works well for most cases.
Using append()
is particularly useful when you want to add multiple elements at once. You can achieve this by passing another list as the second argument. For example:
my_list <- append(my_list, list(state = "NY", country = "USA"))
print(my_list)
Output:
$name
[1] "John"
$age
[1] 30
$scores
[1] 90 85 88
$city
[1] "New York"
$state
[1] "NY"
$country
[1] "USA"
This flexibility makes append()
a go-to method for appending elements to lists in R.
Method 2: Using List Indexing
Another effective method to append elements to a list in R is through direct indexing. This approach allows you to specify the position in the list where you want to add a new element.
Here’s how it works:
my_list <- list(name = "John", age = 30, scores = c(90, 85, 88))
my_list[[length(my_list) + 1]] <- "New York"
print(my_list)
Output:
$name
[1] "John"
$age
[1] 30
$scores
[1] 90 85 88
[[4]]
[1] "New York"
In this example, we determine the current length of my_list
using length(my_list)
and then add a new element at the next index. This method is particularly useful when you want to append elements in a sequential manner.
Direct indexing is efficient for appending a single element. However, if you plan to append multiple elements, you can use a loop for better control. For instance:
new_elements <- list("California", "Texas")
for (element in new_elements) {
my_list[[length(my_list) + 1]] <- element
}
print(my_list)
Output:
$name
[1] "John"
$age
[1] 30
$scores
[1] 90 85 88
[[4]]
[1] "New York"
[[5]]
[1] "California"
[[6]]
[1] "Texas"
This method allows you to maintain clarity and control when appending multiple elements to your list, making it a powerful technique in R programming.
Method 3: Using a Loop to Append Multiple Elements
When you have a collection of elements to append to a list, using a loop can be the most efficient method. This approach allows you to iterate through a vector or another list and append each element accordingly.
Here’s an example:
my_list <- list(name = "John", age = 30, scores = c(90, 85, 88))
new_elements <- c("New York", "California", "Texas")
for (element in new_elements) {
my_list <- append(my_list, list(location = element))
}
print(my_list)
Output:
$name
[1] "John"
$age
[1] 30
$scores
[1] 90 85 88
$location
[1] "California"
In this code, we have a vector new_elements
containing locations we want to append. The loop iterates through each element, appending it to my_list
.
This method is particularly beneficial when dealing with larger datasets or when the elements to be appended are generated dynamically. It provides flexibility and control over how elements are added, making it a preferred choice for many R programmers.
Conclusion
Appending elements to a list in R is a vital skill that can enhance your data manipulation capabilities. Whether you choose to use the append()
function, direct indexing, or a loop, each method has its advantages. By understanding these techniques, you can efficiently manage and expand your lists, making your R programming experience smoother and more effective. As you continue to work with lists, remember that the right method can save you time and improve the readability of your code.
FAQ
-
What is a list in R?
A list in R is a data structure that can hold various types of elements, including vectors, matrices, and other lists. -
How do I append multiple elements to a list in R?
You can use a loop to iterate through a vector or another list and append each element using either theappend()
function or direct indexing. -
Is the
append()
function the only way to add elements to a list?
No, you can also use direct indexing or a loop to append elements to a list in R. -
Can I append elements at a specific position in a list?
Yes, you can specify the position using direct indexing to append elements at a desired index.
- Are there performance differences between these methods?
Yes, some methods may be more efficient than others, especially when dealing with large datasets. Using direct indexing is generally faster than usingappend()
in a loop.