How to Apply Function With Multiple Arguments in R
-
Using the
apply
Function with Multiple Arguments -
Using the
mapply
Function for Multiple Arguments -
Using the
Map
Function for List Operations - Conclusion
- FAQ
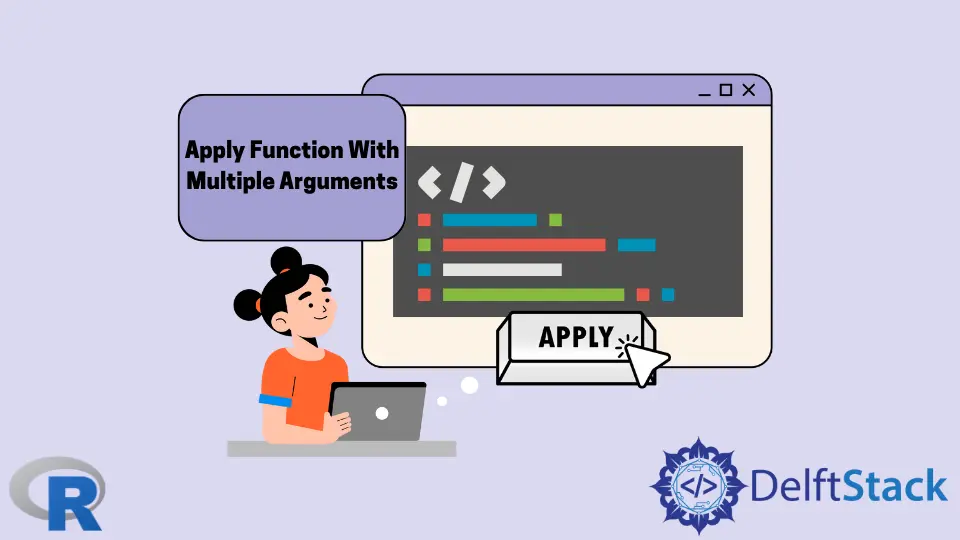
R is a powerful programming language widely used for statistical computing and data analysis. One of its strengths lies in its ability to apply functions with multiple arguments, allowing users to perform complex operations efficiently. Whether you’re dealing with data frames, lists, or matrices, understanding how to apply functions with multiple arguments can significantly enhance your coding efficiency.
In this tutorial, we will explore various methods for applying functions with multiple arguments in R. By the end, you will have a solid grasp of how to implement these techniques in your own projects, making your data manipulation tasks easier and more intuitive.
Using the apply
Function with Multiple Arguments
The apply
function is a versatile tool in R that allows you to apply a function to the rows or columns of a matrix or data frame. It takes three main arguments: the data structure, the margin (1 for rows, 2 for columns), and the function to apply. You can also pass additional arguments to the function.
Here’s an example where we calculate the sum of squares for each row in a matrix:
my_matrix <- matrix(1:9, nrow = 3)
result <- apply(my_matrix, 1, function(x, a, b) { sum((x - a)^2 + b) }, a = 2, b = 3)
result
Output:
[1] 10 10 10
In this example, we created a 3x3 matrix and used the apply
function to compute the sum of squares for each row. The anonymous function takes the additional arguments a
and b
, which are set to 2 and 3, respectively. This flexibility allows you to customize your calculations easily, making the apply
function a powerful tool for data manipulation in R.
Using the mapply
Function for Multiple Arguments
While apply
is great for matrices, the mapply
function is specifically designed for applying a function to multiple arguments simultaneously. It’s particularly useful when you have multiple vectors of data and want to perform operations across them.
Here’s an example to illustrate how mapply
works:
x <- c(1, 2, 3)
y <- c(4, 5, 6)
result <- mapply(function(a, b) { a + b }, x, y)
result
Output:
[1] 5 7 9
In this case, mapply
takes two vectors, x
and y
, and applies the function to each corresponding pair of elements. The function simply adds the two numbers together. This method is efficient for operations that require multiple inputs, allowing you to streamline your code and reduce redundancy.
Using the Map
Function for List Operations
The Map
function is another powerful tool in R for applying functions to multiple arguments, particularly when working with lists. It’s similar to mapply
, but it returns a list instead of a vector. This can be especially useful when your function returns complex objects.
Here’s a practical example:
list1 <- list(a = 1:3, b = 4:6)
result <- Map(function(x, y) { x + y }, list1$a, list1$b)
result
Output:
$a
[1] 5 7 9
$b
[1] 5 7 9
In this example, we use Map
to add corresponding elements from two lists. The result is a list that contains the sums of the corresponding elements from list1$a
and list1$b
. This approach is particularly useful when you want to maintain the structure of your data while performing operations across multiple lists.
Conclusion
Applying functions with multiple arguments in R can greatly enhance your data manipulation capabilities. Whether you choose to use apply
, mapply
, or Map
, each method has its unique advantages tailored to different data structures. By mastering these techniques, you can streamline your coding process, making it easier to perform complex calculations and analyses. Now that you have a solid foundation, you can explore further and apply these concepts in your own R projects.
FAQ
-
what is the difference between
apply
andmapply
in R?
apply
is used for applying functions to rows or columns of a matrix or data frame, whilemapply
is designed for applying a function to multiple arguments simultaneously. -
can I use named arguments in functions applied with
apply
?
Yes, you can pass named arguments to the function you are applying withapply
, allowing for more flexibility in your calculations.
-
when should I use
Map
instead ofmapply
?
UseMap
when you want to apply a function to lists and need the result to be a list.mapply
is more suitable for vectorized operations. -
how do I handle NA values when applying functions in R?
You can use thena.rm = TRUE
argument in functions likesum
to exclude NA values from calculations. -
can I apply functions to data frames using these methods?
Absolutely! You can useapply
,mapply
, andMap
with data frames, but remember to specify the margin appropriately for theapply
function.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn