How to Extract Rows From a Data Frame in R
-
Using Square Brackets (
[]
) to Extract Rows From a Data Frame in R -
Using the
subset()
Function to Extract Rows in R -
Using the
which()
Function to Extract Rows in R - Conclusion
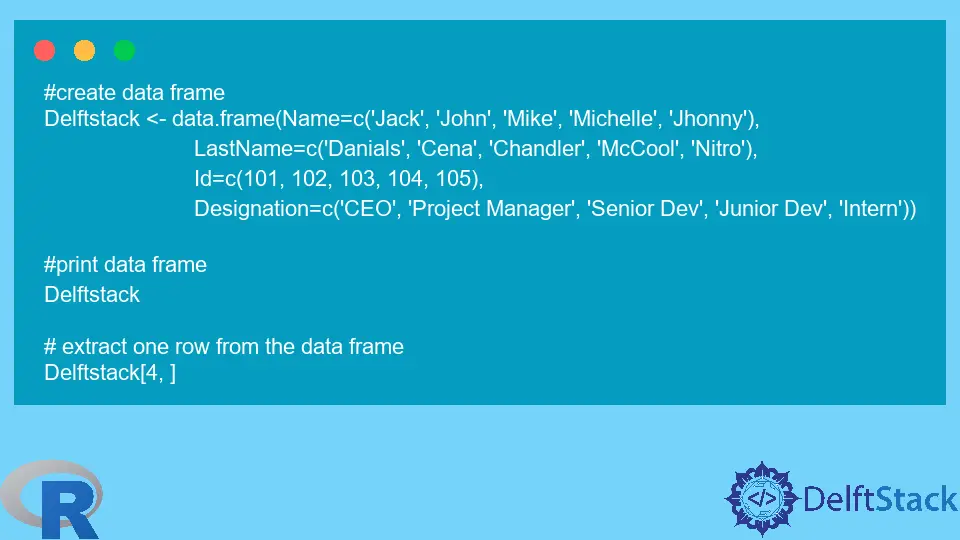
Manipulating and extracting specific rows from a data frame is a common task in data analysis. We can extract rows from a data frame based on our requirements, and either it can be a single row or multiple rows.
This tutorial demonstrates the ways how to extract rows from a data frame in R.
Using Square Brackets ([]
) to Extract Rows From a Data Frame in R
Base R has the functionality to extract rows from a data frame. Extracting a row from a data frame involves using square brackets ([]
) and specifying the row index.
Extract Single Row From a Data Frame
To extract a single row from a data frame in R, we use the square brackets ([]
) and specify the row index.
Basic Syntax:
data_frame[row_index, ]
In the syntax, the data_frame
is the name of your data frame. The row_index
is the index of the row you want to extract.
Code:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
Delftstack[4, ]
In this code, we have a data frame named Delftstack
with columns for 'Name'
, 'LastName'
, 'Id'
, and 'Designation'
. Using square brackets ([]
), we extract the fourth row of the data frame.
Output:
Name LastName Id Designation
4 Michelle McCool 104 Junior Dev
In the output, we can see the extracted row displaying information for Michelle
, McCool
, Id 104
, and Junior Dev
from the data frame.
Extract Multiple Rows From a Data Frame
To extract multiple rows from a data frame in R, we use the square brackets ([]
) and specify a vector of row indices.
Basic Syntax:
data_frame[c(row_index1, row_index2, ..., row_indexN),
]
In the syntax, the data_frame
is the name of your data frame. The row_index1, row_index2, ..., row_indexN
are the indices of the rows you want to extract.
For example, if you want to extract rows 2, 5, and 7 from a data frame named my_data
, the syntax would be:
my_data[c(2, 5, 7),
]
Code:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
Delftstack[c(1, 4),
]
In this code, we have a data frame named Delftstack
. Using square brackets ([]
), we extract rows 1 and 4 from the data frame.
Output:
Name LastName Id Designation
1 Jack Danials 101 CEO
4 Michelle McCool 104 Junior Dev
In the output, it displays the extracted row, displaying information for Jack
and Michelle
from the data frame.
Using the subset()
Function to Extract Rows in R
The subset()
function in R is a convenient way to extract rows from a data frame based on specific conditions.
Basic Syntax:
subset(data_frame, condition)
In the syntax, the data_frame
is the name of the data frame from which you want to extract rows. The condition
determines which rows to extract.
Code:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
subset_data <- subset(Delftstack, Id > 102)
subset_data
In this code, we’re working with the Delftstack
data frame containing individual records. Using the subset()
function, we extract rows where the 'Id'
is greater than 102.
Output:
Name LastName Id Designation
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
In the output, it shows we’ve successfully created a subset of the data frame, including individuals with 'Id'
greater than 102, displaying their 'Name'
, 'LastName'
, 'Id'
, and 'Designation'
.
Using the which()
Function to Extract Rows in R
The which()
function in R is another approach to extracting rows from a data frame based on specific conditions. It is typically used in conjunction with indexing.
Basic Syntax:
data_frame[which(condition),
]
In the syntax, the data_frame
is the name of the data frame from which you want to extract rows. The condition
determines which rows to extract.
Code:
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, 103, 104, 105),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
index <- which(Delftstack$Designation == "CEO")
selected_rows <- Delftstack[index, ]
selected_rows
In this code, we’ve created a data frame named Delftstack
with information about individuals. Using the which()
function, we find the indices where the 'Designation'
is equal to "CEO"
.
We then extract the rows corresponding to these indices.
Output:
Name LastName Id Designation
1 Jack Danials 101 CEO
In the output, it displays the extracted rows where the 'Designation'
is "CEO"
, resulting in a subset named selected_rows
containing relevant information about individuals with that designation.
Conclusion
In conclusion, we’ve explored various methods for extracting specific rows from a data frame in R. Using square brackets ([]
) is the fundamental approach, allowing us to extract both single and multiple rows based on row indices.
The subset()
function provides a convenient way to extract rows based on specified conditions, enhancing flexibility in data manipulation. Additionally, the which()
function serves as an alternative method for row extraction, particularly useful when conditions involve logical expressions.
By mastering these techniques, we empower ourselves with versatile tools for effective data analysis and manipulation in R.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook