How to Count Number of Rows in R
-
Use the
data.frame(table())
Function to Count the Number of Rows in R -
Use the
count()
Function to Count the Number of Rows in R -
Use the
ddply()
Function to Count the Number of Rows in R -
Use the
nrow()
Function to Count the Number of Rows in R -
Use the
dim()
Function to Count the Number of Rows in R -
Use the
length()
Function on Data Frames to Count the Number of Rows in R -
Use the
sum()
Function With Logical Indexing to Count the Number of Rows in R -
Use the
dplyr
Package to Count the Number of Rows in R -
Use the
data.table
Package to Count the Number of Rows in R - Conclusion
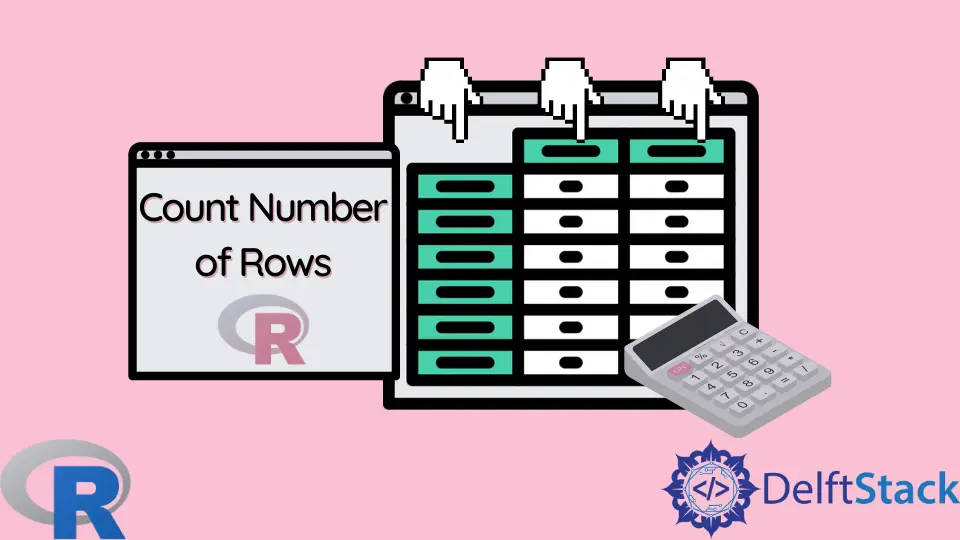
Counting the number of rows is a critical task in data analysis, enabling us to gain insights into the distribution and characteristics of our data. In R, this operation is seamless through various powerful techniques and packages.
This article will explore different methods to count the number of rows within specific groups in a dataset. This knowledge is invaluable for summarizing data, generating reports, and performing advanced analytics.
Use the data.frame(table())
Function to Count the Number of Rows in R
The combination of data.frame()
and table()
in R provides a powerful method for counting the occurrences of unique values in a dataset.
By converting the output of table()
into a data frame, you obtain a structured summary that includes the unique values and their respective frequencies. This approach is particularly useful for categorical data and for gaining insights into the distribution of values within a dataset.
Syntax:
result <- data.frame(table(column_name))
table(column_name)
: Generates a frequency table for the unique values in the specified column (column_name
).data.frame()
: Converts the result oftable()
into a data frame.result
: A variable that holds the resulting data frame.
For example, if you want to count the frequency of unique values in the column named Month
in a data frame df
, you would use:
result <- data.frame(table(df$Month))
This will create a data frame containing two columns: one for the unique values and another for their respective frequencies.
The code below demonstrates how to count the frequency of unique values in a specific column of a data frame using the table()
function and then convert the result into a data frame for easy interpretation and further analysis.
df <- data.frame(Name = c("Jack","Jay","Mark","Sam"),
Month = c("Jan","Jan","May","July"),
Age = c(12,10,15,13))
data.frame(table(df$Month))
This code snippet creates a data frame df
with columns: Name
, Month
, and Age
. It then counts the frequency of each unique month using the table()
function applied to df$Month
.
Finally, the result is converted into a new data frame and printed. The output provides a clear summary of the frequency of each month in the original data frame df
.
Var1 Freq
1 Jan 2
2 July 1
3 May 1
Use the count()
Function to Count the Number of Rows in R
The count()
function, part of the plyr
package, provides a concise way to count the number of rows in a dataset. It is particularly useful when working with data frames and performing group-wise operations, allowing you to obtain a summary of observations based on specific criteria.
Syntax:
count(data, ..., wt = NULL, sort = FALSE)
data
: The input data frame or tibble....
: Additional variables to group by. These can be column names or expressions.wt
: An optional weight variable for counting weighted observations.sort
: Logical value indicating whether the result should be sorted by frequency.
Before using the count()
function, it’s essential to ensure that the plyr
package is installed and loaded in your R environment. If not already installed, you can do so with the following code:
install.packages("plyr")
library(plyr)
One of the powerful features of count()
is its ability to perform group-wise operations (counting rows by groups). By specifying a grouping variable, you can obtain counts for each unique combination within the dataset.
Example 1:
# Load the plyr package
library(plyr)
# Create a sample data frame
data <- data.frame(Name = c("Jack","Jay","Mark","Sam"),
Month = c("Jan","Jan","May","July"),
Age = c(12,10,15,13))
# Count the number of rows by group
count_result <- count(data, vars = "Month")
print(count_result)
In this example, we first load the plyr
package using library(plyr)
. We then create a sample data frame data
with three columns: Name
, Month
, and Age
.
Next, we use the count()
function to count the number of rows by the "Month"
variable. The result will be a summary of unique months and their corresponding frequencies.
Finally, we print the count_result
.
Month freq
1 Jan 2
2 July 1
3 May 1
This indicates that there are 2 rows with the month Jan
, 1 row with May
, and 1 row with July
in the dataset.
The example below is the simplified version of the first example; either way, it displays the same result.
Example 2:
library(plyr)
data <- data.frame(Name = c("Jack","Jay","Mark","Sam"),
Month = c("Jan","Jan","May","July"),
Age = c(12,10,15,13))
count(data, "Month")
Output:
Month freq
1 Jan 2
2 July 1
3 May 1
The choice between using count(data, vars = "Month")
and count(data, "Month")
mainly comes down to coding style preference. They achieve the same outcome but with slightly different syntax.
Use the ddply()
Function to Count the Number of Rows in R
Another interesting function in the plyr
library is the ddply()
function. It splits the data into a subset, specifies some function to be applied to it, and combines the result.
The basic syntax of the ddply()
function involves specifying a data frame, a variable for grouping, and a function to apply.
ddply(.data, .variables, .fun, ...)
.data
: The data frame to be processed..variables
: A specification of the variables to use for grouping. This can be a single variable, a vector of variables, or a formula..fun
: The function to apply to each subset of the data. This can be a built-in function (e.g.,summarize
,transform
) or a custom function defined by the user....
: Additional arguments to be passed to the function specified by.fun
.
Before using ddply()
, you need to install and load the plyr
package. This can be done using the following commands:
install.packages("plyr")
library(plyr)
One of the powerful features of ddply()
is its ability to perform operations on grouped data. This is achieved by specifying one or more variables for grouping.
Example 1:
# Load the plyr package
library(plyr)
# Create a sample data frame
data <- data.frame(Name = c("Jack","Jay","Mark","Sam"),
Month = c("Jan","Jan","May","July"),
Age = c(12,10,15,13))
# Count the number of rows by group
result <- ddply(data, .(Month), summarize, Count = length(Month))
print(result)
In this example, a sample data frame named data
is created with columns for Name
, Month
, and Age
. The ddply()
is used to group the data by the Month
variable and then apply the summarize
function.
Inside summarize
, length(Month)
is used to count the number of rows in each month group. The result is stored in the variable result
.
Finally, the output displays a summary indicating the number of rows for each unique month.
Month Count
1 Jan 2
2 July 1
3 May 1
The example below is the simplified version of the first example; either way, it displays the same result.
Example 2:
library(plyr)
data <- data.frame(Name = c("Jack","Jay","Mark","Sam"),
Month = c("Jan","Jan","May","July"),
Age = c(12,10,15,13))
ddply(data, .(Month), nrow)
The ddply()
function is used directly on data
, grouping by the Month
variable. The nrow
function is applied to each group, which counts the number of rows in each month group.
Output:
Month V1
1 Jan 2
2 July 1
3 May 1
Both codes will produce similar results, which would be a summary of unique months and their respective row counts.
The choice between using ddply(data, .(Month), summarize, Count = length(Month))
and ddply(data, .(Month), nrow)
depends on coding style preference. They achieve the same outcome but with slightly different syntax and function usage.
Use the nrow()
Function to Count the Number of Rows in R
The nrow()
function is a simple and straightforward way to count the number of rows in a data frame or matrix.
Syntax:
nrow(x)
Here, x
represents the object (matrix or data frame) you want to analyze. The result is an integer representing the number of rows in x
.
The following code is an example of using nrow()
to count the number of rows in R.
# Create a sample data frame
data <- data.frame(
Name = c("John", "Jane", "Jim", "Jill"),
Age = c(25, 30, 22, 28),
Score = c(85, 92, 78, 88)
)
# Count the number of rows
num_rows <- nrow(data)
# Print the result
cat("The data frame has", num_rows, "rows.")
In this example, we create a data frame data
with columns for Name
, Age
, and Score
. We then use nrow()
to count the number of rows, which is 4
in this case.
Output:
The data frame has 4 rows.
Use the dim()
Function to Count the Number of Rows in R
The dim()
function returns a vector with the dimensions of an object, be it a matrix or a data frame. To get the number of rows, you can extract the first element of the result.
Syntax:
dim(x)
Here, x
represents the object under consideration. For matrices, dim()
returns a numeric vector with two elements: the number of rows followed by the number of columns.
For data frames, it provides the number of rows as the 1st element and the number of columns as the 2nd element.
Example:
# Create a sample matrix
matrix_data <- matrix(1:12, nrow = 4)
# Count the number of rows
row_count <- dim(matrix_data)[1]
print(row_count)
The code above creates a matrix named matrix_data
with 4 rows and 3 columns using the values 1 to 12.
The dim()
function is applied to matrix_data
to get its dimensions, and [1]
is used to extract the number of rows. This value is stored in the variable row_count
and prints it.
Output:
[1] 4
Use the length()
Function on Data Frames to Count the Number of Rows in R
In R, the length()
function is typically used to determine the number of elements in an object.
One of the most notable features of the length()
function is its versatility. Unlike nrow()
, which specifically counts rows, length()
can count both rows and columns within a data frame.
Syntax:
length(x)
Here, x
represents the object you want to analyze, which could be a vector, list, or data frame. The result is an integer representing the number of elements in x
.
You can use the length()
function on data frames to get the number of columns and use it on one of the columns to get the number of rows.
Example:
# Create a sample data frame
data <- data.frame(
Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 30, 22)
)
# Count the number of rows
row_count <- length(data$Name)
print(row_count)
The code above creates a sample data frame named data
with two columns: Name
and Age
. The length()
function is applied to the Name
column to count the number of rows in the data frame.
The resulting count is stored in a variable named row_count
, then the print()
method prints it.
Output:
[1] 3
Use the sum()
Function With Logical Indexing to Count the Number of Rows in R
The sum()
function in R is primarily used to calculate the total of numeric values in a vector, matrix, or data frame. However, when combined with logical indexing, sum()
takes on a new role: it can be used to count rows that meet specific conditions.
Syntax:
sum(logical_vector)
Here, logical_vector
is a vector of logical values (TRUE
/FALSE
), where TRUE
indicates rows that meet the specified condition.
The following code is an example of how you can use the sum()
function with logical indexing to count the number of rows that meet a specific condition.
# Create a sample data frame
data <- data.frame(
Name = c("Alice", "Bob", "Charlie"),
Age = c(25, 30, 22)
)
# Count the number of rows where Age is greater than 25
row_count <- sum(data$Age > 25)
print(row_count)
The code above generates a sample data frame named data
with two columns: Name
and Age
.
Then, it evaluates a condition to count the number of rows where the Age
is greater than 25
. It achieves this by summing up the TRUE
values from the comparison data$Age > 25
.
The resulting count is stored in a variable named row_count
, then the print()
method prints it.
Output:
[1] 1
Use the dplyr
Package to Count the Number of Rows in R
dplyr
is a highly regarded R package designed for fast and efficient data manipulation. It provides a set of intuitive functions that streamline common data-wrangling operations.
To get started, install and load the dplyr
package:
install.packages("dplyr")
library(dplyr)
The n()
function, part of the dplyr
package, allows you to count the number of rows in a data frame or data set.
Example:
library(dplyr)
# Create a sample data frame
data <- data.frame(
Name = c("John", "Jane", "Jim", "Jill"),
Age = c(25, 30, 22, 28),
Score = c(85, 92, 78, 88)
)
# Count the number of rows
num_rows <- data %>% summarize(num_rows = n())
# Print the result
cat("The data frame has", num_rows$num_rows, "rows.")
This code creates a sample dataset with columns Name
, Age
, and Score
. Then, it counts the rows using the summarize()
function along with n()
. The result is stored in num_rows
and printed, indicating there are 4 rows in the dataset.
Output:
The data frame has 4 rows.
Use the data.table
Package to Count the Number of Rows in R
The data.table
package extends R’s capabilities for handling and manipulating large datasets. Its syntax is designed for both simplicity and efficiency.
The core of the data.table
is the data.table
object, which is similar to a data frame but equipped with enhanced functionality and optimized performance.
To get started, install and load the data.table
package:
install.packages("data.table")
library(data.table)
At the heart of row counting with data.table
lies the .N
special symbol. It efficiently counts the number of rows within groups specified by a key.
Example:
# Create a sample data.table
dt <- data.table(
Group = c("A", "A", "B", "B", "B", "A", "C"),
Value = c(10, 15, 8, 12, 7, 9, 11)
)
# Count rows by 'Group'
row_counts <- dt[, .N, by = Group]
# Print the result
print(row_counts)
In this example, we first create a data.table
named dt
with two columns: Group
and Value
. Then, we use dt[, .N, by = Group]
to count the rows by the Group
column.
The result will be a data.table
with two columns: Group
and N
, where N
represents the count of rows within each group.
Output:
Group N
1: A 3
2: B 3
3: C 1
Conclusion
In this guide, we’ve covered several efficient methods for counting rows in R. We started with the combination of data.frame()
and table()
, which provides a structured summary of unique values.
The count()
function from the plyr
package offers a concise approach for group-wise row counting. Additionally, ddply()
from the same package excels in complex operations on grouped data.
The straightforward nrow()
function directly counts rows in a data frame or matrix. On the other hand, dim()
provides the dimensions (including row count) of an object. length()
can be used to count elements, especially in columns of data frames.
The sum()
function, with logical indexing, allows for conditional row counting. Meanwhile, dplyr
introduces n()
for easy row counting. Lastly, the data.table
package, known for handling large datasets efficiently, employs .N
for powerful group-wise row counting.
These methods cater to various preferences and needs, equipping analysts with versatile tools for effective data analysis in R.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn