Ways to Remove xa0 From a String in Python
-
Use the Unicodedata’s
Normalize()
Function to Remove\xa0
From a String in Python -
Use the String’s
replace()
Function to Remove\xa0
From a String in Python -
Use the
BeautifulSoup
Library’sget_text()
Function to Remove\xa0
From a String in Python -
Use the
translate()
Method to Remove\xa0
From a String in Python -
Use List Comprehension to Remove
\xa0
From a String in Python -
Use the
str.split()
andstr.join()
Methods to Remove\xa0
From a String in Python - Conclusion
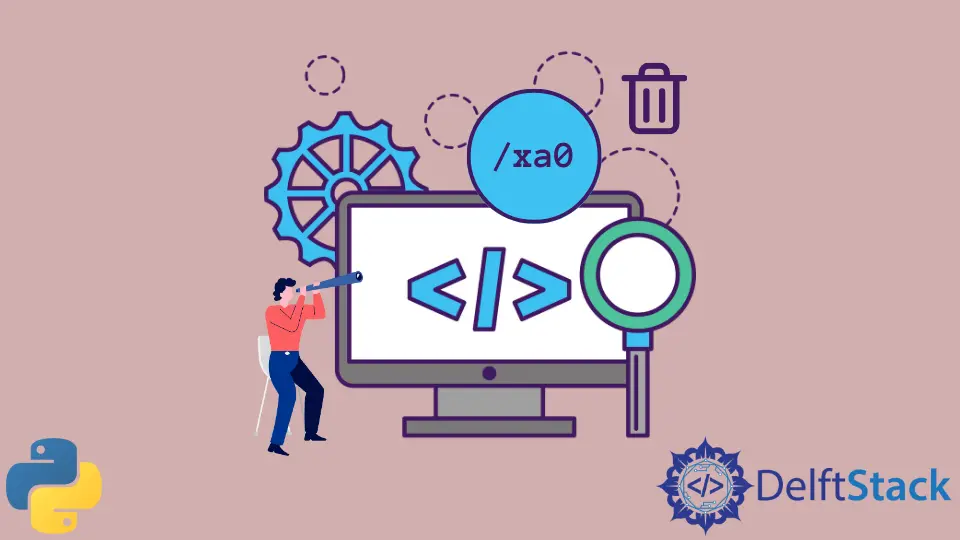
In Python, dealing with strings containing special characters, such as \xa0
(non-breaking space), often requires effective methods for cleaning and manipulation.
This article provides a comprehensive guide to removing \xa0
from a string using various techniques, showcasing the versatility of Python’s string manipulation capabilities.
Use the Unicodedata’s Normalize()
Function to Remove \xa0
From a String in Python
One powerful approach to remove special characters or non-breaking spaces, such as \xa0
, is to use the normalize()
function from the unicodedata
standard library. This allows us to transform and clean strings by converting them to a specific Unicode normalization form.
The normalize()
function takes two arguments: the normalization form and the input string. For removing \xa0
, we use the normalization form NFKD (Normal Form Compatibility Decomposition).
The syntax is as follows:
import unicodedata
normalized_string = unicodedata.normalize("NFKD", input_string)
Here, the NFKD
normalization form decomposes characters into their base and combines characters, effectively replacing compatibility characters like \xa0
with their equivalent characters.
Now, let’s see the complete working code example:
import unicodedata
str_with_hard_space = "17\xa0kg on 23rd\xa0June 2021"
if "\xa0" in str_with_hard_space:
print("xa0 is Found!")
else:
print("xa0 is not Found!")
normalized_str = unicodedata.normalize("NFKD", str_with_hard_space)
if "\xa0" in normalized_str:
print("xa0 is Found!")
else:
print("xa0 is not Found!")
print(normalized_str)
In this example, we start with a string containing \xa0
. Before normalization, we check for the presence of \xa0
in the original string.
Then, we use unicodedata.normalize("NFKD", str_with_hard_space)
to obtain a new string with \xa0
removed. After normalization, we again check for the presence of \xa0
.
Output:
xa0 is Found!
xa0 is not Found!
17 kg on 23rd June 2021
In the output, you can observe that the \xa0
is successfully removed from the original string after applying the normalize()
function.
Use the String’s replace()
Function to Remove \xa0
From a String in Python
When it comes to manipulating strings in Python, the replace()
function provides another straightforward way to substitute specific substrings.
The replace()
function is applied directly to a string and takes two arguments: the substring to be replaced and the replacement string. In the context of removing \xa0
, the syntax is as follows:
new_string = original_string.replace("\xa0", " ")
Here, u"\xa0"
represents the non-breaking space character, and u" "
is the replacement—essentially replacing each occurrence of \xa0
with a regular space.
Now, let’s see a code example:
str_with_hard_space = "16\xa0kg on 24th\xa0June 2021"
if "\xa0" in str_with_hard_space:
print("xa0 is Found!")
else:
print("xa0 is not Found!")
new_str = str_with_hard_space.replace("\xa0", " ")
if "\xa0" in new_str:
print("xa0 is Found!")
else:
print("xa0 is not Found!")
print(new_str)
Similar to the previous example, we begin with a string containing \xa0
. Before the replacement operation, we check for the presence of \xa0
in the original string.
The replace()
function is then applied, replacing every occurrence of \xa0
with a regular space. After the replacement, we check again for the presence of \xa0
.
Finally, we print the modified string.
Output:
xa0 is Found!
xa0 is not Found!
16 kg on 24th June 2021
The output demonstrates the successful removal of \xa0
from the original string using the replace()
function. This method provides a simple yet effective solution for substituting specific substrings, making it a valuable tool in string manipulation tasks in Python.
Use the BeautifulSoup
Library’s get_text()
Function to Remove \xa0
From a String in Python
The BeautifulSoup
library is a powerful tool for parsing and manipulating HTML and XML documents in Python. When faced with strings containing special characters like \xa0
, the library’s get_text()
function, combined with proper settings, can provide an elegant solution for their removal.
To use the get_text()
function with BeautifulSoup
for removing \xa0
, you need to create a BeautifulSoup
object from the input HTML or XML and then apply the get_text()
with the strip
parameter set to True
:
The syntax is as follows:
clean_text = BeautifulSoup(html_content, "lxml").get_text(strip=True)
Here, html_content
is the input string containing HTML with non-breaking spaces, and the get_text()
function is applied with strip=True
to remove leading and trailing whitespaces.
Let’s dive into the complete working code example:
import lxml
from bs4 import BeautifulSoup
html_content = (
"This is a test message, Hello This is a test message, Hello\xa0here"
)
print(html_content)
clean_text = BeautifulSoup(html_content, "lxml").get_text(strip=True)
print(clean_text)
In this example, we start with an HTML string containing \xa0
. The original HTML content is printed for reference.
We then use BeautifulSoup(html_content, "lxml").get_text(strip=True)
to obtain clean text by stripping out HTML tags and removing non-breaking spaces. Finally, the clean text is printed.
Output:
This is a test message, Hello This is a test message, Hello here
This is a test message, Hello This is a test message, Hello here
In the output, you can see that the \xa0
is successfully removed, resulting in clean and readable text. The get_text()
function, in conjunction with BeautifulSoup
, offers a convenient way to handle HTML content and extract text while handling non-breaking spaces and other HTML entities.
Use the translate()
Method to Remove \xa0
From a String in Python
The translate()
method provides a versatile way to modify characters within a string, making it an effective tool also for tasks like removing unwanted characters such as \xa0
. The translate()
method is usually employed in conjunction with the str.maketrans()
method, which creates a translation table.
The syntax is as follows:
translation_table = str.maketrans("", "", "\xa0")
new_string = original_string.translate(translation_table)
Here, original_string
is the input string containing \xa0
, and str.maketrans('', '', '\xa0')
generates a translation table instructing the translate()
method to remove occurrences of \xa0
in the string.
Let’s explore the complete working code example:
str_with_hard_space = "Your string with\xa0non-breaking space."
if "\xa0" in str_with_hard_space:
print("xa0 Found!")
else:
print("xa0 not Found!")
translation_table = str.maketrans("", "", "\xa0")
new_str = str_with_hard_space.translate(translation_table)
if "\xa0" in new_str:
print("xa0 Found!")
else:
print("xa0 not Found!")
print(new_str)
In this example, we check for the presence of \xa0
in the original string (containing \xa0
) before applying the translation. The str.maketrans('', '', '\xa0')
function creates a translation table indicating that \xa0
should be replaced with an empty string.
The translate()
method then uses this table to remove \xa0
from the original string, resulting in a new string. We check for the presence of \xa0
in the new string and print the modified string.
Output:
xa0 Found!
xa0 not Found!
Your string withnon-breaking space.
In the output, you can observe that the \xa0
has been successfully removed from the original string using the translate()
method.
Use List Comprehension to Remove \xa0
From a String in Python
List comprehension provides a concise and readable way to transform strings, making it a flexible approach for tasks like removing specific characters such as \xa0
.
List comprehension allows us to create a new string by iterating over the characters of the original string and including only those that do not match the unwanted character, in this case, \xa0
.
The syntax is as follows:
new_string = "".join([char for char in original_string if char != "\xa0"])
Here, original_string
is the input string containing \xa0
, and the list comprehension [char for char in original_string if char != '\xa0']
generates a list of characters excluding \xa0
. The join()
method then combines these characters into a new string.
Let’s delve into the complete code example:
str_with_hard_space = "Your string with\xa0non-breaking space."
if "\xa0" in str_with_hard_space:
print("xa0 Found!")
else:
print("xa0 not Found!")
new_str = "".join([char for char in str_with_hard_space if char != "\xa0"])
if "\xa0" in new_str:
print("xa0 Found!")
else:
print("xa0 not Found!")
print(new_str)
Here, we first check for the presence of any \xa0
in the original string. Then, the list comprehension [char for char in str_with_hard_space if char != '\xa0']
iterates over each character in the original string, excluding those equal to \xa0
.
The resulting list is then joined using the join()
method to form a new string. Again, we check for the presence of \xa0
in the new string and print the modified string.
Output:
xa0 Found!
xa0 not Found!
Your string withnon-breaking space.
Here, you can see that the \xa0
has been successfully removed from the original string using list comprehension.
Use the str.split()
and str.join()
Methods to Remove \xa0
From a String in Python
The combination of str.split()
and str.join()
provides an alternative method for effectively removing unwanted characters, such as \xa0
, from a string.
The idea behind this approach is to split the original string into a list of substrings using str.split()
, effectively breaking it at instances of \xa0
. Then, we join these substrings back together using str.join()
to form a new string without the unwanted character.
The syntax is as follows:
new_string = " ".join(original_string.split("\xa0"))
Here, original_string
is the input string containing \xa0
, and original_string.split('\xa0')
creates a list of substrings, which are then joined using ' '.join()
to reconstruct the string without \xa0
.
Let’s explore the complete working code example:
str_with_hard_space = "Your string with\xa0non-breaking space."
if "\xa0" in str_with_hard_space:
print("xa0 Found!")
else:
print("xa0 not Found!")
new_str = " ".join(str_with_hard_space.split("\xa0"))
if "\xa0" in new_str:
print("xa0 Found!")
else:
print("xa0 not Found!")
print(new_str)
After checking for the presence of \xa0
in the original string, we apply the method str_with_hard_space.split('\xa0')
, breaking the original string into a list of substrings wherever \xa0
is encountered. Subsequently, we use ' '.join()
to concatenate these substrings, effectively removing \xa0
.
We check again for the presence of \xa0
in the new string after the splitting and joining operations and print the modified string.
Output:
xa0 Found!
xa0 not Found!
Your string with non-breaking space.
In the output, you can observe that the \xa0
has been successfully removed from the original string using the combination of str.split()
and str.join()
. This method offers a simple and intuitive way to handle character removal in Python strings.
Conclusion
By exploring these methods, you have a range of options for handling non-breaking spaces in Python strings. Choose the method that best fits your specific use case, ensuring clean and readable results in your string manipulation tasks.