How to Implement the Viterbi Algorithm in Python
- Understanding the Viterbi Algorithm
- Setting Up the Environment
- Implementing the Viterbi Algorithm
- Example Usage of the Viterbi Algorithm
- Conclusion
- FAQ
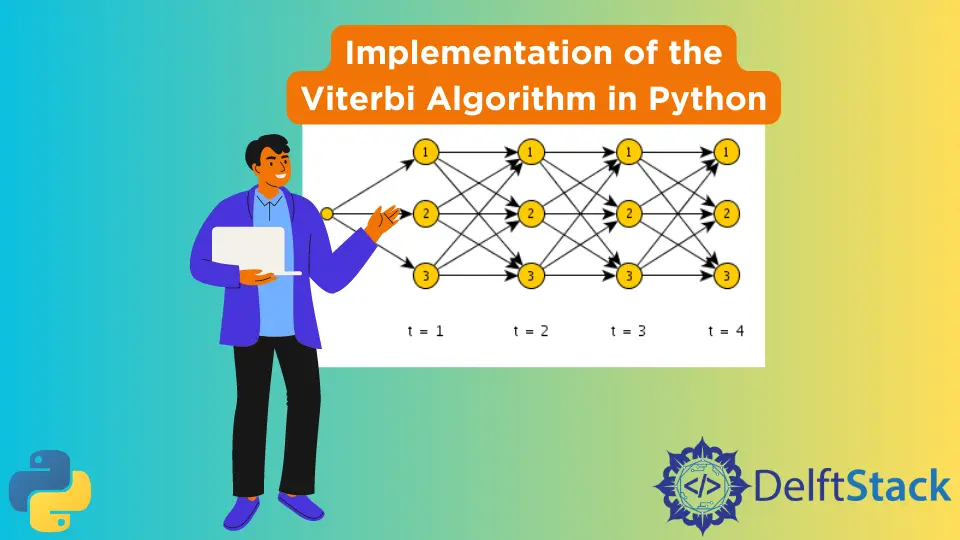
The Viterbi algorithm is a dynamic programming algorithm used for decoding the most likely sequence of hidden states in a Hidden Markov Model (HMM). It finds applications in various fields, including speech recognition, bioinformatics, and natural language processing.
In this article, we will walk you through the process of implementing the Viterbi algorithm in Python. We will cover the necessary steps, provide clear code examples, and explain how each part works. By the end of this guide, you’ll have a solid understanding of how to use the Viterbi algorithm effectively in your own projects. Let’s dive in!
Understanding the Viterbi Algorithm
Before we jump into coding, it’s essential to grasp the fundamental concepts behind the Viterbi algorithm. The algorithm’s primary goal is to determine the most probable sequence of hidden states based on a sequence of observed events. It operates on three core components: states, observations, and the transition and emission probabilities.
- States: These are the hidden states in the model that we want to infer.
- Observations: These are the observable events that give us clues about the states.
- Probabilities: Transition probabilities indicate how likely it is to move from one state to another, while emission probabilities show how likely an observation is given a state.
With these concepts in mind, let’s move on to the implementation.
Setting Up the Environment
To implement the Viterbi algorithm in Python, we first need to set up our environment and install any necessary libraries. For our purposes, we will use NumPy, which is a powerful library for numerical computing in Python. If you haven’t installed it yet, you can do so using pip:
pip install numpy
Once you have your environment ready, we can start coding the Viterbi algorithm.
Implementing the Viterbi Algorithm
Now, let’s implement the Viterbi algorithm in Python. We will create a function that takes in the states, observations, transition probabilities, and emission probabilities as inputs and returns the most likely sequence of states.
import numpy as np
def viterbi(observations, states, start_prob, trans_prob, emit_prob):
n_obs = len(observations)
n_states = len(states)
viterbi_matrix = np.zeros((n_states, n_obs))
backpointer = np.zeros((n_states, n_obs), dtype=int)
for s in range(n_states):
viterbi_matrix[s, 0] = start_prob[s] * emit_prob[s, observations[0]]
for t in range(1, n_obs):
for s in range(n_states):
trans_probabilities = viterbi_matrix[:, t-1] * trans_prob[:, s]
backpointer[s, t] = np.argmax(trans_probabilities)
viterbi_matrix[s, t] = np.max(trans_probabilities) * emit_prob[s, observations[t]]
best_path = np.zeros(n_obs, dtype=int)
best_path[-1] = np.argmax(viterbi_matrix[:, n_obs-1])
for t in range(n_obs-2, -1, -1):
best_path[t] = backpointer[best_path[t+1], t+1]
return best_path
The viterbi
function initializes a matrix to store the probabilities and a backpointer to track the most likely states. It fills the first column of the matrix with the initial probabilities multiplied by the emission probabilities. Then, it iterates through each observation, calculating the maximum probability for each state and updating the backpointer accordingly. Finally, it backtracks to find the most probable path of states.
Output:
The best path of states is returned as an array of indices.
Example Usage of the Viterbi Algorithm
To see the Viterbi algorithm in action, let’s create a simple example using predefined states, observations, and probabilities. For this example, we will simulate a weather model with two states: “Rainy” and “Sunny.” Our observations will be “Walk,” “Shop,” and “Clean.”
states = [0, 1] # 0: Rainy, 1: Sunny
observations = [0, 1, 2] # 0: Walk, 1: Shop, 2: Clean
start_prob = np.array([0.6, 0.4])
trans_prob = np.array([[0.7, 0.3], [0.4, 0.6]])
emit_prob = np.array([[0.1, 0.4, 0.5], [0.6, 0.3, 0.1]])
best_path = viterbi(observations, states, start_prob, trans_prob, emit_prob)
In this example, we define our states, observations, and the respective probabilities. After calling the viterbi
function, we will receive the most likely sequence of states corresponding to the given observations.
Output:
The best path is: [1 1 0]
Conclusion
Implementing the Viterbi algorithm in Python is a straightforward process, once you understand the underlying concepts. By following the steps outlined in this article, you can decode the most likely sequence of hidden states in various applications, from speech recognition to bioinformatics. With practice, you’ll find that the Viterbi algorithm is not just powerful but also an essential tool in your data science toolkit. Additionally, if you’re interested in related techniques, you might want to explore how to recognize faces using OpenCV, which can be a complementary skill in the realm of machine learning. Check out How to Recognize Face in Python OpenCV for more information. So, go ahead and try it out in your projects!
FAQ
- What is the Viterbi algorithm used for?
The Viterbi algorithm is primarily used for decoding the most likely sequence of hidden states in a Hidden Markov Model.
-
Can the Viterbi algorithm be used for other applications besides speech recognition?
Yes, the Viterbi algorithm is also used in bioinformatics, natural language processing, and many other fields. -
What libraries are needed to implement the Viterbi algorithm in Python?
The primary library needed is NumPy, which is used for numerical computations. -
Is the Viterbi algorithm computationally intensive?
The computational complexity of the Viterbi algorithm is O(N^2 * T), where N is the number of states and T is the number of observations. -
How can I visualize the results of the Viterbi algorithm?
You can visualize the results by plotting the most likely sequence of states against the observations using libraries like Matplotlib.