How to Get Values of Dictionary in Python
-
Using the
values()
Method - Accessing Values by Key
-
Using the
get()
Method - List Comprehension for Extracting Values
- Conclusion
- FAQ
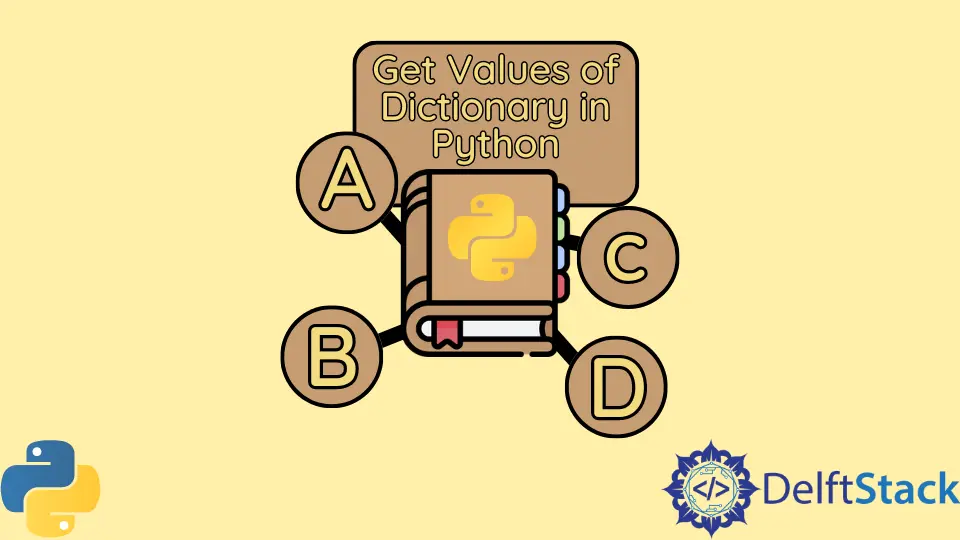
Dictionaries are one of the most versatile and widely used data structures in Python. They allow you to store data in key-value pairs, making it easy to retrieve, update, and manipulate information. If you’re working with dictionaries, knowing how to extract values is essential for effective coding.
This tutorial will guide you through various methods to get values from a dictionary in Python, providing clear examples and detailed explanations. Whether you’re a beginner or looking to brush up on your skills, this guide will help you navigate the world of Python dictionaries with ease.
Using the values()
Method
The simplest way to retrieve all the values from a dictionary in Python is to use the built-in values()
method. This method returns a view object that displays a list of all the values in the dictionary. Here’s how it works:
my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
values = my_dict.values()
print(values)
Output:
dict_values(['Alice', 30, 'New York'])
In this example, we create a dictionary called my_dict
with three key-value pairs. When we call my_dict.values()
, it returns a view object containing all the values. Note that the output is a special type of view object, which means it reflects changes to the dictionary. If you were to add or remove items from my_dict
, the view would update accordingly.
This method is particularly useful when you want to iterate over the values without needing to access the keys. It’s a straightforward approach that keeps your code clean and efficient.
Accessing Values by Key
Another common way to get values from a dictionary is by accessing them through their keys. This method allows you to retrieve specific values based on their associated keys. Here’s an example:
my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
name = my_dict['name']
age = my_dict['age']
print(name)
print(age)
Output:
Alice
30
In this example, we directly access the values associated with the keys 'name'
and 'age'
. When you use the syntax my_dict['key']
, Python retrieves the value corresponding to that key. This method is straightforward and efficient, especially when you know the keys you want to access.
However, be cautious: if you attempt to access a key that doesn’t exist in the dictionary, Python will raise a KeyError
. To avoid this, you can use the get()
method, which allows you to specify a default value if the key is not found.
Using the get()
Method
The get()
method is another powerful way to retrieve values from a dictionary. It not only fetches the value associated with a specified key but also allows you to provide a fallback value if the key is missing. Here’s how to use it:
my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
city = my_dict.get('city')
country = my_dict.get('country', 'USA')
print(city)
print(country)
Output:
New York
USA
In this example, we use my_dict.get('city')
to retrieve the value associated with the key 'city'
. Since this key exists, we get 'New York'
. However, when we try to access 'country'
, which does not exist in the dictionary, we provide a default value of 'USA'
. This prevents a KeyError
and ensures that our code runs smoothly.
The get()
method is particularly useful when dealing with dictionaries where certain keys may or may not be present, making your code more robust and less error-prone.
List Comprehension for Extracting Values
If you want to extract specific values based on certain conditions, list comprehension can be a powerful tool. This method allows you to create a new list containing only the values that meet your criteria. Here’s an example:
my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York', 'is_student': False}
values_above_25 = [value for key, value in my_dict.items() if isinstance(value, int) and value > 25]
print(values_above_25)
Output:
[30]
In this example, we use a list comprehension to filter and extract values that are integers and greater than 25. The expression iterates over each key-value pair in the dictionary using my_dict.items()
, checking if the value meets our criteria. The result is a new list, values_above_25
, containing only the values that satisfy the condition.
List comprehension not only makes your code concise but also enhances readability, allowing you to perform complex operations with minimal lines of code.
Conclusion
In this tutorial, we explored various methods to get values from a dictionary in Python. From using the values()
method to accessing values by keys, utilizing the get()
method, and employing list comprehension for conditional extraction, each method offers unique advantages. Understanding these techniques will empower you to work more effectively with dictionaries, making your Python programming experience smoother and more enjoyable. Whether you’re developing applications or analyzing data, mastering dictionary operations is a crucial skill in your coding toolkit.
FAQ
-
How do I retrieve all values from a Python dictionary?
You can use thevalues()
method to get all values from a dictionary. -
What happens if I try to access a non-existing key in a dictionary?
If you try to access a non-existing key, Python will raise aKeyError
. -
How can I avoid a KeyError when accessing dictionary values?
You can use theget()
method, which allows you to specify a default value if the key is not found. -
What is list comprehension in Python?
List comprehension is a concise way to create lists by applying an expression to each item in an iterable, allowing for filtering and transformation. -
Can I update a dictionary while retrieving its values?
Yes, the view object returned by thevalues()
method reflects changes to the dictionary, so it will update automatically if you modify the dictionary.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn