How to Use of Memory Caching in Python
- Understanding Memory Caching
- Setting Up Memcached
- Basic Caching Operations
- Advanced Caching Patterns
- Using Cache as a Decorator
- Conclusion
- FAQ
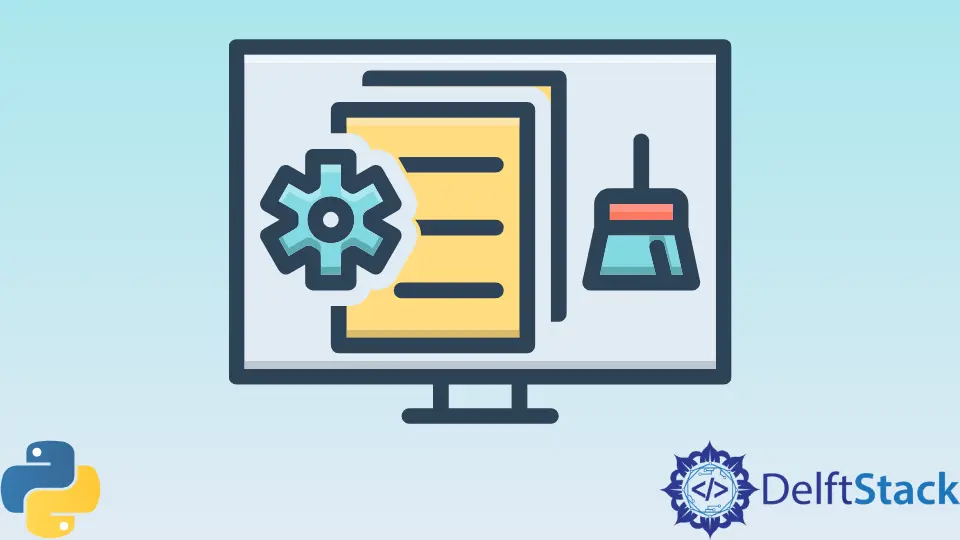
Memory caching is a powerful technique that can significantly enhance the performance of your Python applications. By storing frequently accessed data in memory, you can reduce the time it takes to retrieve that data, leading to faster response times and a better user experience.
In this article, we’ll explore how to effectively implement memory caching in Python, focusing on Memcached as a primary tool. We will also cover some advanced caching patterns, including the use of cache and set operations. Whether you’re building a web application or a data-heavy service, mastering memory caching can give your project a substantial performance boost.
Understanding Memory Caching
Memory caching is a method of storing data in a temporary storage area (the cache) to speed up data retrieval. Instead of fetching data from a database or a remote server every time a request is made, your application can check the cache first. If the data is present, it can be returned almost instantly. If not, the application fetches the data from the original source, stores it in the cache for future requests, and then returns it to the user.
Using a caching system like Memcached, you can easily manage this process. Memcached is an in-memory key-value store that is designed to speed up dynamic web applications by alleviating database load. It is particularly effective for caching database query results, API calls, or any data that is expensive to compute.
Setting Up Memcached
To get started with memory caching in Python, the first step is to install Memcached and the Python client library. You can install Memcached on your system using the following command:
sudo apt-get install memcached
Next, you need to install the python-memcached
library. This library allows your Python application to interact with Memcached easily. You can install it via pip:
pip install python-memcached
Once you have Memcached and the library installed, you can start the Memcached server. By default, it runs on port 11211, but you can customize this if needed.
Output:
memcached is running on port 11211
Now that you have Memcached up and running, you can start implementing caching in your Python application.
Basic Caching Operations
The first step in using Memcached with Python is to perform basic caching operations. You can set, get, and delete cached items using the Memcached
client. Here’s a simple example to illustrate these operations.
import memcache
# Connect to the Memcached server
client = memcache.Client(['127.0.0.1:11211'], debug=0)
# Set a value in the cache
client.set('key', 'value')
# Retrieve the value
value = client.get('key')
# Delete the value
client.delete('key')
In this code, we first import the memcache
library and create a client that connects to the Memcached server. We then set a key-value pair in the cache, retrieve it, and finally delete it.
Output:
value
The set
method stores the value associated with the key in the cache. When we call get
, it retrieves the value if it exists, and delete
removes the key-value pair from the cache. This basic functionality allows you to manage cached data effectively.
Advanced Caching Patterns
Once you are comfortable with basic operations, you can explore advanced caching patterns. These patterns can optimize your caching strategy further, especially in applications with complex data requirements. One common pattern is using cache expiration and cache invalidation.
import memcache
import time
client = memcache.Client(['127.0.0.1:11211'], debug=0)
# Set a value with an expiration time of 5 seconds
client.set('temporary_key', 'temporary_value', time=5)
# Wait for 6 seconds to allow the key to expire
time.sleep(6)
# Try to retrieve the value after expiration
expired_value = client.get('temporary_key')
In this example, we set a key-value pair with a 5-second expiration time. After waiting for 6 seconds, we attempt to retrieve the value.
Output:
None
After the expiration period, the key is no longer available in the cache, and the get
method returns None
. This pattern is particularly useful for data that is only relevant for a limited time, such as session data or temporary results from a computation.
Using Cache as a Decorator
Another advanced pattern is using caching as a decorator. This method allows you to cache the results of a function call automatically. Here’s how you can implement it:
import memcache
from functools import wraps
client = memcache.Client(['127.0.0.1:11211'], debug=0)
def cache_decorator(key):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
value = client.get(key)
if value is not None:
return value
value = func(*args, **kwargs)
client.set(key, value)
return value
return wrapper
return decorator
@cache_decorator('expensive_function_key')
def expensive_function():
# Simulate an expensive operation
return sum(i * i for i in range(10000))
In this code, we define a cache_decorator
that takes a key as an argument. The decorator checks if the result is already cached. If it is, it returns the cached value; otherwise, it calls the original function, caches the result, and returns it.
Output:
333383335000
This approach is highly efficient for functions that are called frequently and have expensive computations. By caching the results, you can save time and resources, improving the overall performance of your application.
Conclusion
Memory caching is an essential technique for optimizing Python applications. By leveraging tools like Memcached, you can significantly reduce data retrieval times and improve user experience. In this article, we covered the basics of setting up Memcached, performing fundamental caching operations, and exploring advanced caching patterns. Whether you’re a seasoned developer or just starting, understanding memory caching can help you build faster and more efficient applications. Start implementing these techniques today, and watch your application’s performance soar.
FAQ
-
what is memory caching?
Memory caching is a technique that stores frequently accessed data in a temporary storage area to speed up data retrieval. -
how does memcached work?
Memcached is an in-memory key-value store that caches data to reduce the load on databases and improve application performance. -
can I use memcached with any programming language?
Yes, Memcached has client libraries available for various programming languages, including Python, PHP, Java, and more. -
what are the benefits of using caching in applications?
Caching improves application performance, reduces latency, and decreases the load on databases, resulting in a better user experience. -
how do I clear the cache in memcached?
You can use theflush_all
command to clear all items from the Memcached cache.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn