Python Cache Library
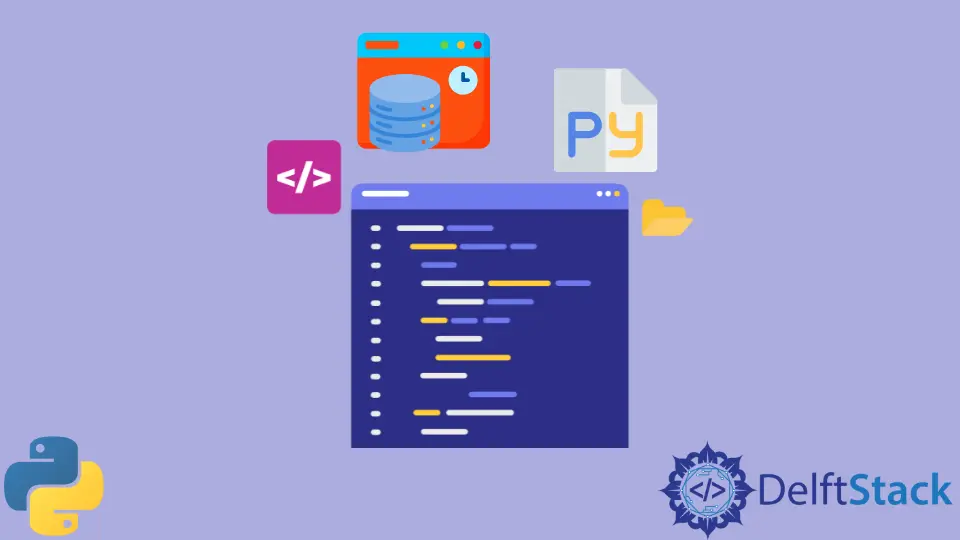
The cache is a type of memory that can store data for quick access. It is a small, fast memory that holds frequently accessed data.
The cache is essential because it can help improve the performance of a system by reducing the many times the system has to access slow, main memory.
Cache Library
A cache library is a Python library that provides a way to cache data in memory. It can be utilized to speed up access to frequently accessed data or decrease the number of data retrieved from a backend store.
Cache libraries typically provide a simple API that allows developers to store and retrieve data from the cache.
Types of Cache Libraries
There are two types of cache libraries available for use:
- The standard cache library
- And the high-performance cache library
Every type has pros and cons that should be checked when deciding what to use.
The standard cache library is the most widely used and is compatible with most systems. However, it is not as speedy as the high-performance cache library and does not provide as much protection against data loss.
The high-performance cache library is much faster than the standard and provides more protection against data loss. However, it is not as widely compatible with systems and can be more challenging to use.
Useful Caching Libraries in Python
A cache library is a collection of routines used to manage the cache. The cache library provides a way for the system to access the data in the cache, and it also provides a way to manage the cache.
Python is a universal language that can be used for various programming tasks. As such, it has several different caching libraries available to suit the needs of other applications.
The most useful caching libraries for Python are discussed in the following sections.
Redis
Cache Library in Python
Redis
is a powerful in-memory caching library that supports many data structures. It is speedy and scalable, making it ideal for high-traffic applications.
Redis
is an open-source, in-memory data structure store that can be used as a database, cache, and message broker.
To use the Redis
cache library with Python, you must install the Redis-py
library, a Python interface to the Redis
key-value store. Once you have installed the Redis-py
library, you can use the following code to connect to a Redis
server.
Example Code:
import redis
r = redis.Redis(host="localhost", port=6379, db=0)
The code above will connect to a Redis
server running on localhost on port 6379. The Redis
server will be using database 0.
Once connected to the Redis
server, you can start using the Redis
cache. For example, you can set a key-value pair in the cache as follows:
r.set("foo", "bar")
You can then retrieve the value of the key foo
from the cache as follows:
r.get("foo")
The value of the key foo
will be returned as a string.
lru_cache
Library in Python
The lru_cache
library is an excellent tool for caching data in Python. It is easy to work with and can be very helpful in improving performance.
This library works by keeping a cache of recently used data in memory, so it can be easily accessed the next time it is needed. This can be a great way to improve the speed of your Python code.
Example Code:
from functools import lru_cache
@lru_cache(maxsize=256)
def f(x):
return x * x
for x in range(4):
print(f(x))
print("")
for x in range(4):
print(f(x))
Output:
0
1
4
9
0
1
4
9
Alternative Cache Libraries in Python
Some other Python cache libraries are the following.
Memcached
is another popular in-memory caching solution. It is simple to work with and has a wide range of features.Python-Memcached
is a wrapper around the famousMemcached
library; it makesMemcached
easier to use in Python applications.pylibmc
is a high-performanceMemcached
client in pure Python. It is speedy and scalable, making it ideal for high-traffic applications.Django Cache Machine
is a caching library for Django applications. It is very efficient and provides a wide range of features.Flask-Cache
is a caching extension for Flask applications. It is effortless to use and provides a wide range of features.
Conclusion
The cache is typically implemented as a hardware device but can also be implemented in software. The cache implemented in software is usually called a cache library.
A caching library is essential to improve your Python applications’ performance. Python cache package is a very lightweight package used to speed up applications.
Not all caching libraries are the same. Choose the one that will be perfect for your project.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn