Raw String and Unicode String in Python
- What are Unicode Strings in Python?
- What are Raw Strings in Python?
- Differences Between Raw Strings and Unicode Strings
- When to Use Raw Strings in Python
- When to Use Unicode Strings in Python
- Conclusion
- FAQ
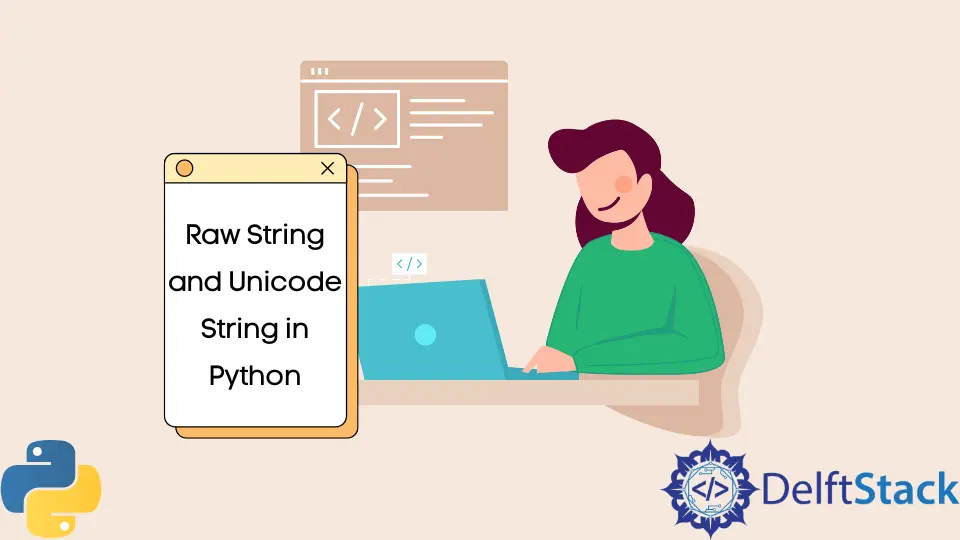
Understanding string types in Python is crucial for effective programming. Two significant string types are raw strings and Unicode strings, which are denoted by the ‘r’ and ‘u’ prefixes, respectively. While both serve specific purposes in handling text, they differ fundamentally in how they process escape characters and encode characters.
In this article, we will delve into the differences between these two string types, explain how raw string literals work, and provide practical examples to illustrate their usage. By the end of this article, you will have a clear understanding of when to use raw strings and Unicode strings in your Python projects.
What are Unicode Strings in Python?
Unicode strings are a way to represent characters from a wide range of languages and symbols. In Python 2, Unicode strings are defined using the ‘u’ prefix. This allows developers to handle text that includes characters from multiple languages, ensuring that their applications can work with international text seamlessly.
Here’s an example of a Unicode string:
unicode_string = u"Hello, 世界"
print(unicode_string)
Output:
Hello, 世界
In this example, the Unicode string contains both English and Chinese characters. The ‘u’ prefix indicates that the string should be treated as a Unicode string, allowing Python to correctly interpret and display the characters. This is particularly useful in applications dealing with user input from various languages or when interfacing with external systems that require Unicode encoding.
Unicode strings are essential for global applications, as they ensure that text is represented correctly regardless of the language. With the rise of globalization, understanding and using Unicode strings is more important than ever for developers.
What are Raw Strings in Python?
Raw strings, denoted by the ‘r’ prefix, are strings where escape sequences are not processed. This means that backslashes are treated as literal characters, making raw strings particularly useful for regular expressions and file paths, where backslashes are common.
Consider the following example of a raw string:
raw_string = r"C:\Users\Name\Documents"
print(raw_string)
Output:
C:\Users\Name\Documents
In this case, the raw string preserves the backslashes in the file path. If we had used a regular string without the ‘r’ prefix, Python would interpret the backslashes as escape characters, potentially leading to errors or unexpected behavior.
Using raw strings can simplify code when working with regular expressions, as they often contain many backslashes. By using raw strings, developers can avoid the confusion of double escaping characters, making the code cleaner and easier to read.
Differences Between Raw Strings and Unicode Strings
While both raw strings and Unicode strings serve specific purposes, they are fundamentally different in how they handle characters and escape sequences.
-
Escape Characters: Raw strings ignore escape sequences, while Unicode strings process them. For instance, in a raw string, a backslash remains a backslash, whereas in a Unicode string, it may be interpreted as an escape character.
-
Character Encoding: Unicode strings are designed to handle a wide range of characters from various languages, ensuring that they can represent text accurately. Raw strings, on the other hand, are not concerned with character encoding; they simply treat all characters literally.
-
Usage Context: Raw strings are typically used in scenarios involving file paths or regular expressions, where backslashes are common. Unicode strings are essential when dealing with international text, ensuring that applications can handle characters from multiple languages.
Understanding these differences can help developers choose the appropriate string type based on the specific requirements of their applications.
When to Use Raw Strings in Python
Raw strings are particularly useful in scenarios where backslashes are prevalent, such as file paths and regular expressions. By using raw strings, developers can avoid the need for double escaping backslashes, which can make code cleaner and easier to read.
For example, consider a regular expression that matches a pattern with backslashes:
import re
pattern = r"\d{3}-\d{2}-\d{4}"
text = "My SSN is 123-45-6789"
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
Output:
Match found: 123-45-6789
In this example, the raw string allows us to define the regular expression pattern without worrying about escaping the backslashes. This makes the code more readable and easier to maintain.
Using raw strings in regular expressions is a best practice, as it simplifies the pattern definition and reduces the likelihood of errors. Whenever you find yourself working with backslashes, consider using a raw string to make your code cleaner.
When to Use Unicode Strings in Python
Unicode strings are essential for applications that need to handle text in multiple languages or special characters. By using Unicode strings, developers can ensure that their applications can accurately represent and manipulate international text.
For example, consider an application that collects user input in various languages:
user_input = u"Bonjour, ça va?"
print(user_input)
Output:
Bonjour, ça va?
In this case, the Unicode string allows us to represent French characters correctly. This is particularly important in applications that require user input in different languages, as it ensures that the text is displayed accurately.
Using Unicode strings is crucial in today’s globalized world, where applications often need to support multiple languages. By understanding how to use Unicode strings effectively, developers can create applications that cater to a diverse user base, enhancing user experience and accessibility.
Conclusion
In conclusion, understanding the differences between raw strings and Unicode strings in Python is essential for effective programming. Raw strings simplify the handling of escape characters, making them ideal for file paths and regular expressions. On the other hand, Unicode strings are crucial for representing text from various languages, ensuring accurate character representation. By knowing when to use each type, developers can write cleaner, more efficient code that meets the needs of their applications. Embrace these string types in your Python projects to enhance functionality and maintainability.
FAQ
-
What is the difference between raw strings and Unicode strings in Python?
Raw strings ignore escape sequences, while Unicode strings process them for character representation. -
When should I use raw strings in Python?
Use raw strings when working with file paths or regular expressions that contain backslashes. -
Are Unicode strings necessary for modern applications?
Yes, Unicode strings are essential for handling text in multiple languages and ensuring accurate character representation. -
Can I use raw strings with Unicode characters?
Yes, you can combine both by using raw Unicode strings, likeru"example"
.
- How do I define a raw string in Python?
You define a raw string by prefixing it with ‘r’, liker"example"
.