How to Create Turtle Graphics in Python
- Create a Screen for the Graphics Output in Python
-
Create a Square Using
Turtle()
in Python -
Create an Octagon Using
Turtle()
in Python -
Create a Hexagon Using
Turtle()
in Python -
Create a Star Using
Turtle()
in Python -
Create a Circle Using
Turtle()
in Python - Other Useful Functions in the Turtle Library in Python
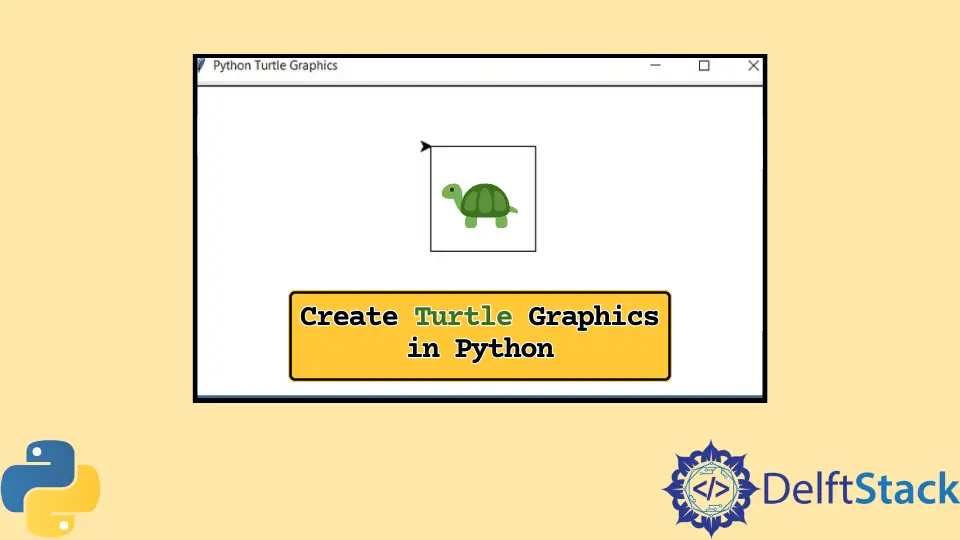
Logo programming is a basic programming language that can create shapes and figures using basic commands. It is introduced to kids to give them a basic understanding of programming.
In Python, we can use the turtle
library to work with turtle graphics which emulate the Logo programming language. This pre-installed library lets us create and work with turtle graphics with simple commands like Logo programming.
This tutorial will discuss some examples of turtle graphics in Python.
Create a Screen for the Graphics Output in Python
We start with creating the screen for the output. The turtle.getscreen()
method initializes a variable as a screen, and a screen pops up.
This variable is altered, and the output is formed on the screen. We then need to initialize the Turtle
object with the turtle.Turtle()
constructor to draw on the screen.
Some basic functions that can move the turtle with the above object are forward()
, right()
, left()
, and backward()
. Short form versions of these functions can also be used as fd()
, rt()
, lt()
, and bk()
, respectively.
The forward()
and backward()
functions will move the turtle up and down, respectively, whereas the right()
and left()
functions will rotate the turtle to a given angle.
We can use these functions with loops and conditional statements to create different shapes and figures. Let us see them below.
Create a Square Using Turtle()
in Python
Creating a square is a relatively simple task. We will iterate a loop four times.
The turtle will move a specific distance in each iteration and turn left or right at 90 degrees.
See the code below.
import turtle
obj = turtle.Turtle()
for i in range(4):
obj.forward(100)
obj.right(90)
turtle.done()
Output:
In the above example, we initialized a Turtle
object and created a square by simply running the loop several times, moving the turtle with the forward()
function, and turning it with the right()
function.
Create an Octagon Using Turtle()
in Python
We have to rotate the turtle at the required angle and iterate the loop eight times to create an octagon. We can calculate the angle necessary to rotate by dividing 360 by the number of sides, which in this case is eight.
See the code below.
import turtle
obj = turtle.Turtle()
angle = 360.0 / 8
for i in range(8):
obj.forward(100)
obj.right(angle)
turtle.done()
Output:
Create a Hexagon Using Turtle()
in Python
Similarly, a hexagon can be created by iterating the loop six times and rotating the turtle at sixty degrees (360/6).
Example:
import turtle
obj = turtle.Turtle()
angle = 360.0 / 6
for i in range(6):
obj.forward(100)
obj.right(angle)
turtle.done()
Output:
Create a Star Using Turtle()
in Python
A star is a much more complex figure than the ones discussed previously. We will start by rotating the turtle to 75 degrees and moving it forward.
Then, this will be iterated four times by rotating it to an angle of 144 degrees and moving it the same distance.
See the code below.
import turtle
obj = turtle.Turtle()
obj.right(75)
obj.forward(100)
for i in range(4):
obj.right(144)
obj.forward(100)
turtle.done()
Output:
Create a Circle Using Turtle()
in Python
The turtle
library has some pre-built functions to create shapes directly.
The circle()
function can create a circle using turtle graphics. We only need to specify the radius of the circle within the function.
Example:
import turtle
obj = turtle.Turtle()
obj.circle(100)
turtle.done()
Output:
Other Useful Functions in the Turtle Library in Python
We can edit the screen on which the output is produced. To change the background color, we use the turtle.bgcolor()
function, and to add a title on the screen, we use the turtle.title()
function.
We can also control the size and color of the turtle using the turtle.shapesize()
and turtle.fillcolor()
functions.
We use the previously discussed functions in the following code.
import turtle
obj = turtle.Turtle()
turtle.title("DelftStack")
turtle.bgcolor("red")
obj.shapesize(3, 3, 3)
obj.fillcolor("blue")
turtle.done()
Output:
As one can observe, the shape and color of the turtle have changed. Also, the screen had a red background and a title on the window.
We can change the shape of the turtle with the turtle.shape()
function.
To control the size and color of the pen drawing on the screen, we use the turtle.pensize()
and turtle.pencolor()
functions.
Example:
import turtle
obj = turtle.Turtle()
obj.pensize(5)
obj.pencolor("red")
obj.forward(100)
turtle.done()
Output:
In the above example, we can see that the size of the pen is magnified by five times, and the color was also altered. The pen()
function can control the attributes discussed previously in one function.
This function can use the pencolor
, pensize
, fillcolor
, and speed
parameters. The fillcolor
attribute will fill the created shape with some color.
The speed
attribute controls the speed at which the pen moves.
See the code below.
import turtle
obj = turtle.Turtle()
obj.pen(pensize=5, pencolor="blue", fillcolor="red", speed=10)
obj.begin_fill()
obj.circle(100)
obj.end_fill()
turtle.done()
Output:
This article discussed the basics of turtle graphics in Python with the turtle
library. We discussed how to move the turtle on the screen and create basic shapes.
We also discussed how to create a shape with a pre-built function. Different methods were discussed to control and edit the screen, turtle, and pen on the screen.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn