How to Hide Turtle Icon in Python
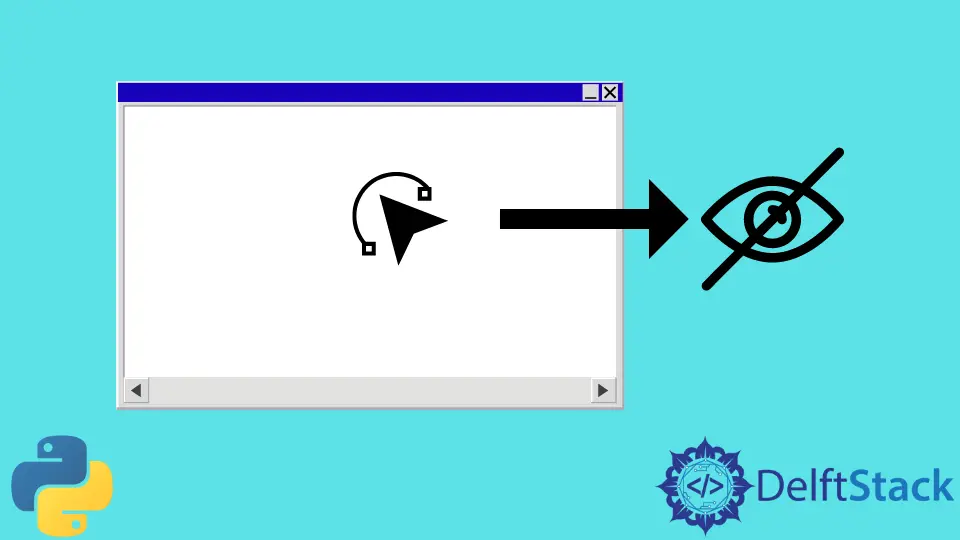
Turtle
is a pre-installed library that can be accessed when Python is installed. It is useful in creating pictures, drawing shapes, creating designs for users by providing them with a virtual canvas.
Turtle is the pen seen on the screen that is used for drawing.
While drawing an icon, we can choose to hide the turtle drawing icon. The benefits of this include improved visibility or aesthetics of the drawing by the turtle. It also observably increases drawing speed, especially when a complex type of drawing is in progress. The library has special methods to control the visibility of the turtle icon. These are .hideturtle()
and .showturtle()
which hide and show the drawing icon respectively.
By default, the icon is in a .showturtle()
state. To hide the icon will require you to call the .hideturtle()
method. Drawings still proceed even when the turtle icon cannot be seen.
Use .hideturtle()
to Hide Turtle in Python
To hide the turtle icon, add this method to the name of the turtle variable or add it directly to the turtle.
turtle.hideturtle()
Alternatively, the library provides a different way to call the hide method, as shown below.
turtle.ht()
Where ht
stands for hide turtle
.
After hiding the icon, you may want to confirm the invisibility of the icon by calling the .isvisible()
method to turtle.
import turtle
turtle.hideturtle()
turtle.isvisible()
Output:
False
Initially, we hide the turtle icon using the .hideturtle()
method. Then, we check whether the icon is still visible onscreen, which will return False
.
The default state for the turtle icon is always visible, and the user can instead set the icon to an invisible state from the beginning of the program. Do this by setting the visible
keyword argument passed to the Turtle
object as False
.
import turtle
turtle_icon = turtle.Turtle(visible=False)
To set the icon to invisible from the start allows the user to move the icon to the problem’s logical starting point before making the icon visible. This is especially useful when you perform utility purposes like writing text.
When the Turtle object is defined without setting the visible keyword to False
, the turtle icon will only for a moment be visible.
import turtle
# turtle icon not hidden initially
turtle_icon = turtle.Turtle() # icon will momentarily show
# calll .hideturtle method to hide the icon
turtle_icon.hideturtle()
Calling the .showturtle()
method after .hideturtle()
will turn turtle’s icon state from invisible to visible. To ensure this test is true, call the .isvisible() method.
turtle.hideturtle()
turtle.showturtle()
turtle.isvisible()
Output:
True
The visible state of the icon will be true as expected.
Alternatively, the following syntax can be used instead of the turtle.showturtle
method.
turtle.st()
Here, st
stands for show turtle
.