tostring() Equivalent in Python
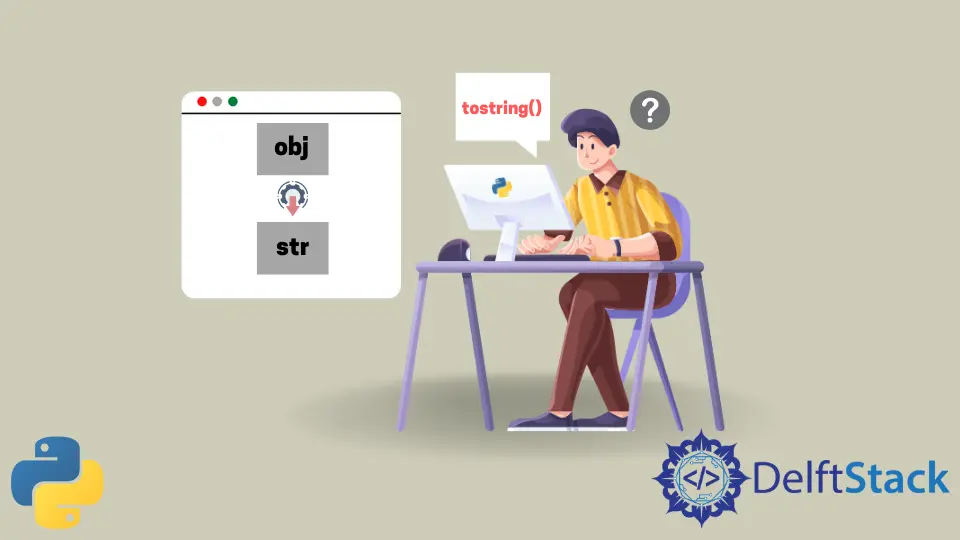
In Python, a string is a sequence of characters. Everything enclosed between quotation marks is considered a string in Python.
Strings are used heavily in almost every programming language. It is a prevalent feature, and every language has different methods to deal with strings. The tostring()
function is a common method available in different languages to cast objects of different types into strings.
In Python, the equivalent of the tostring()
is the str()
function.
The str()
is a built-in function. It can convert an object of a different type to a string. When we call this function, it calls the __str__()
function internally to get the representation of the object as a string.
The following code shows different examples of this function.
a = 15
l1 = [1, 2, 3]
s_l1 = str(l1)
s_a = str(a)
print(s_a, type(s_a))
print(s_l1, type(s_l1))
Output:
15 <class 'str'>
[1, 2, 3] <class 'str'>
As you can see, we were able to convert a number and a list to string type. It is interesting to see how we can also convert a collection object like a list to a string.
In Python, we have a few methods available to format strings. The format()
function is used for this and can also convert objects like numbers to string type.
The following code will show how.
a = 15
l1 = [1, 2, 3]
s_l1 = "{}".format(l1)
s_a = "{}".format(a)
print(s_a, type(s_a))
print(s_l1, type(s_l1))
Output:
15 <class 'str'>
[1, 2, 3] <class 'str'>
In recent versions of Python, we have a new feature called fstring
to format a string.
We can use these fstrings
for string conversion also. For example,
a = 15
l1 = [1, 2, 3]
s_l1 = f"{l1}"
s_a = f"{a}"
print(s_a, type(s_a))
print(s_l1, type(s_l1))
Output:
15 <class 'str'>
[1, 2, 3] <class 'str'>
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn