How to Strip Multiple Characters in Python
-
Use
str.replace()
to Strip Multiple Characters in Python String -
Use the
re.sub()
Function to Strip Multiple Characters in Python String -
Use the
string.translate()
Method to Strip Multiple Characters in Python String -
Use the
str.strip()
in a Loop to Strip Multiple Characters in Python String - Conclusion
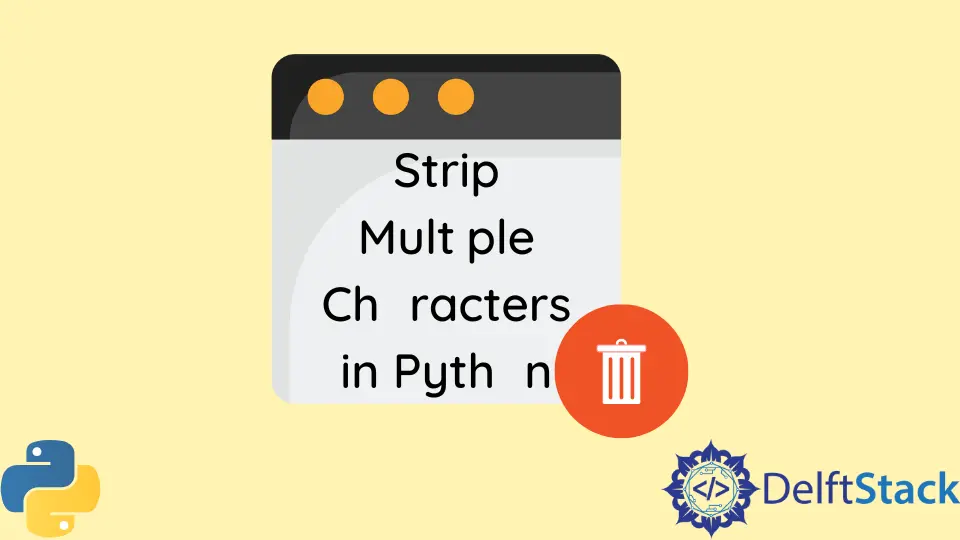
Stripping characters in a string object is an important task you must know as a programmer. Stripping means that you should be able to remove characters from any position.
Before starting with stripping, you must keep in mind that Python string objects are immutable. Therefore, they cannot be modified, and that’s why we will store our stripped string in a new string in each example.
In this tutorial, we will look at several methods and provide examples of striping multiple characters in Python.
Use str.replace()
to Strip Multiple Characters in Python String
The str.replace()
method in Python is a built-in string method used for replacing occurrences of a specified substring with another substring.
Syntax:
new_string = original_string.replace(old, new, count)
In the syntax, the original_string
is where replacements will be performed. The old
is the substring to be replaced.
The new
is the substring that will replace occurrences of the old
substring. Lastly, count
is optional, is the maximum number of occurrences to replace and if not specified or set to -1
, all occurrences will be replaced.
Example Code:
original_string = "!(Hell0o)"
characters_to_remove = "!()0"
new_string = original_string
for character in characters_to_remove:
new_string = new_string.replace(character, "")
print(new_string)
Output:
Hello
In the given code, we start with an original_string
that contains the value "!(Hell0o)"
. We initialize a characters_to_remove
string with the characters "!()0"
. Next, we create a copy of the original_string
called new_string
.
We then iterate through each character in characters_to_remove
using a for loop. During each iteration, we use the str.replace()
method to remove the current character from the new_string
.
This process is repeated for each character in characters_to_remove
. Finally, we print the resulting new_string
.
In this specific example, the characters !
, (
, )
, and 0
are removed from the original string, and the output is Hello
.
Use the re.sub()
Function to Strip Multiple Characters in Python String
The re.sub()
function in Python is part of the re
(regular expression) module and is used for performing substitutions in a string based on a regular expression pattern. It allows us to look for a pattern in a string and replace it with a specific value.
This is another common way to strip the characters from strings in Python.
Syntax:
re.sub(pattern, repl, string, count=0, flags=0)
The syntax performs string substitutions in the input string by replacing occurrences of a specified regular expression pattern with a designated replacement string, with optional parameters for limiting the number of substitutions and controlling the behavior of the regular expression.
Parameters:
pattern
: The pattern you want to search for and replace using regular expressions.repl
: The string that will replace the matches found by the pattern.string
: The input string where substitutions will be performed.count
(optional): The maximum number of substitutions to perform. If not specified or set to0
, all occurrences will be replaced.flags
(optional): Additional flags that control the behavior of the regular expression. For example,re.IGNORECASE
can be used to perform a case-insensitive search.
Example Code 1:
import re
original_string = "!(pyth000on)"
characters_to_remove = "!()0"
pattern = "[" + characters_to_remove + "]"
new_string = re.sub(pattern, "", original_string)
print(new_string)
Output:
python
In the code above, we’re utilizing the re
module in Python to manipulate a string. We start with the original_string
containing "!(pyth000on)"
.
The characters we want to remove are specified in the characters_to_remove
string as "!()0"
. We construct a regular expression pattern pattern
by enclosing these characters in square brackets and using re.sub()
.
We replace any occurrences of this pattern with an empty string in the original_string
, resulting in a modified string stored in new_string
. Finally, we print new_string
, which is the original_string
with the specified characters removed.
In this case, it removes the characters !
, (
, )
, and 0
, yielding the output python
.
In the next code example, we have defined a string consisting of characters we want to remove from our source string. Then, we used the re.sub()
function that takes three arguments: regular expression pattern, the string you want to replace it with, and the source string.
Example Code 2:
import re
original_string = "!(pyth000on is mYy pro0gra@mm!ing langu@age3)"
characters_to_remove = "!()0Y@!3"
pattern = "[" + characters_to_remove + "]"
new_string = re.sub(pattern, "", original_string)
print(new_string)
Output:
python is my programming language
In this code, we set the original_string
to "!(pyth000on is mYy pro0gra@mm!ing langu@age3)"
. The characters we want to remove are specified in the characters_to_remove
string as "!()0Y@!3"
.
Next, we create a regular expression pattern pattern
by enclosing these characters in square brackets and using the re.sub()
function, and any occurrences of this pattern are replaced with an empty string in the original_string
. The modified string is stored in the variable new_string
.
Finally, we print new_string
, which represents the original_string
with the specified characters removed. In this specific case, it removes characters !
, (
, )
, 0
, Y
, @
, and 3
, resulting in the output python is my programming language
.
Use the string.translate()
Method to Strip Multiple Characters in Python String
The str.translate()
method in Python is used for replacing characters in a string with the specified translation table. It’s a powerful and flexible method, often used for advanced string manipulations.
We can also use the string.translate()
method to remove a particular number of characters from a Python string.
Syntax:
string.translate(table)
In the syntax, it takes an argument, table
. This table
could be a dictionary or a mapping table describing the characters we want to replace, and we can assign empty values to both x
and y
to strip the characters.
Example Code:
s = "APythonAProgramming"
x = "A"
y = " "
table = s.maketrans(x, y)
print(s.translate(table))
Output:
Python Programming
In this code, we initialize a string variable s
with the value "APythonAProgramming"
. The characters we want to replace are specified in x
, where we set it to "A"
, and the replacement is defined in y
as a space character (" "
).
Next, we create a translation table using s.maketrans(x, y)
. This table instructs the translate()
method to replace occurrences of characters in x
with the corresponding characters in y
.
Finally, we print the result of applying this translation to the original string, which removes the "A"
characters and replaces them with spaces. Consequently, the output is Python Programming
.
Use the str.strip()
in a Loop to Strip Multiple Characters in Python String
The str.strip()
method in Python is used to remove leading (at the beginning) and trailing (at the end) characters (whitespace by default) from a string. The str.strip()
doesn’t modify the original string; it returns a new string with the specified characters removed.
If we want to modify the original string, we need to assign the result back to the variable.
Example Code:
original_string = "!(pyth000on)"
characters_to_strip = "!()0"
for char in characters_to_strip:
original_string = original_string.replace(char, "")
print(original_string)
Output:
python
In this code, we start with an original_string
initialized to "!(pyth000on)"
. We have a set of characters specified in the characters_to_strip
string as "!()0"
.
Using a for
loop, we iterate through each character in characters_to_strip
, and within each iteration, we apply the replace()
method to remove occurrences of the current character from the original_string
. Consequently, this process eliminates the characters !
, (
, )
, and 0
from the original string.
The final result, stored in original_string
, is then printed, which is python
.
Conclusion
Mastering the skill of stripping characters from strings is crucial for any programmer, especially considering that Python string objects are immutable. This article explored various methods for stripping multiple characters in Python strings, demonstrating the use of str.replace()
for simple replacements, re.sub()
for more complex pattern-based substitutions, str.translate()
for powerful and flexible manipulations with a translation table, and str.strip()
in a loop for removing leading and trailing characters.
Each method provides a unique approach to string manipulation, offering us the flexibility to choose the suitable method based on our specific requirements.