String Builder Equivalent in Python
- Using String Concatenation
-
Using the
join()
Method - Using StringIO for Large Strings
- Using f-Strings for String Formatting
- Conclusion
- FAQ
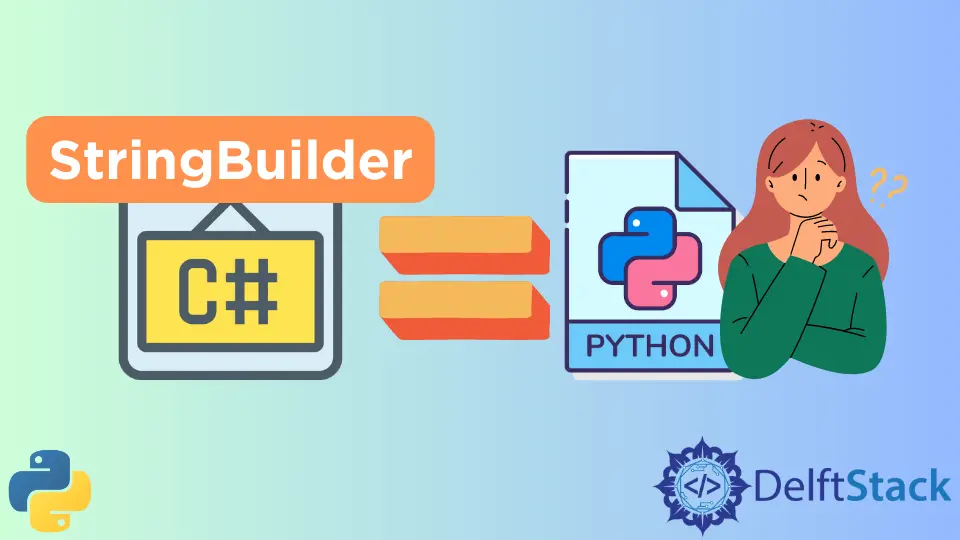
When it comes to string manipulation in programming, efficiency is key. In languages like Java, the StringBuilder class is a go-to for building strings dynamically without the overhead of creating multiple immutable string instances. But what about Python? Python doesn’t have a direct equivalent of StringBuilder, yet it provides several powerful ways to achieve similar functionality.
In this tutorial, we will explore various methods to build strings efficiently in Python. Whether you’re constructing complex strings from multiple components or optimizing performance in your applications, this guide will equip you with the knowledge you need to handle strings like a pro.
Using String Concatenation
One of the simplest methods for building strings in Python is through concatenation. This involves using the +
operator to combine multiple strings. Although this method is straightforward, it’s essential to note that each concatenation creates a new string object, which can lead to inefficiencies in scenarios involving a large number of concatenations.
Here’s how string concatenation works in Python:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
Output:
Hello World
In this example, we start with two strings, str1
and str2
. By using the +
operator, we concatenate them with a space in between. While this works well for a small number of strings, you might notice performance issues if you attempt to concatenate strings in a loop or with a large dataset. Each concatenation generates a new string, leading to increased memory usage and slower execution times.
Using the join()
Method
A more efficient way to build strings in Python is by using the join()
method. This method is particularly useful when you have a list of strings that you want to combine into a single string. The join()
method is faster than concatenation because it constructs the final string in one go, rather than creating multiple intermediate strings.
Here’s an example:
words = ["Hello", "World", "from", "Python"]
result = " ".join(words)
print(result)
Output:
Hello World from Python
In this code snippet, we have a list of words that we want to join with spaces. By calling " ".join(words)
, we efficiently concatenate all the strings in the list into one single string. This method is not only faster but also more memory-efficient, especially when dealing with large datasets. It’s a best practice to use join()
when you need to combine multiple strings, as it significantly improves performance.
Using StringIO for Large Strings
For cases where you need to build large strings or perform extensive string manipulations, Python’s io.StringIO
module offers a powerful alternative. The StringIO
class provides an in-memory stream for text I/O, allowing you to append strings without creating multiple string objects. This method is especially beneficial when constructing large strings in loops.
Here’s an example of how to use StringIO
:
from io import StringIO
output = StringIO()
output.write("Hello")
output.write(" ")
output.write("World")
result = output.getvalue()
output.close()
print(result)
Output:
Hello World
In this example, we create an instance of StringIO
and use the write()
method to append strings. After all the strings have been added, we call getvalue()
to retrieve the complete string. This approach is significantly more efficient than concatenating strings directly, especially when dealing with a large number of strings or performing repeated operations. By using StringIO
, you minimize memory overhead and improve performance, making it an excellent choice for complex string-building tasks.
Using f-Strings for String Formatting
Another modern and efficient way to build strings in Python is through f-strings, introduced in Python 3.6. F-strings allow for inline expression evaluation, making string formatting more readable and concise. This method is particularly useful when you want to include variables or expressions directly within your strings.
Here’s how f-strings work:
name = "World"
greeting = f"Hello {name}!"
print(greeting)
Output:
Hello World!
In this example, we define a variable name
and use it within an f-string to create a greeting. F-strings are not only easy to read but also efficient, as they are evaluated at runtime. This makes them a great choice for building strings dynamically, especially when combining multiple variables or expressions. For anyone looking to improve their string manipulation skills in Python, mastering f-strings is a must.
Conclusion
In summary, while Python does not have a direct equivalent to Java’s StringBuilder, it offers various methods for efficient string manipulation. From simple concatenation to the more advanced join()
method, StringIO
, and f-strings, each approach has its advantages depending on the context. By understanding these methods, you can optimize your string handling in Python, making your code cleaner and more efficient. Whether you’re working on small scripts or large applications, mastering these techniques will enhance your programming toolkit.
FAQ
-
What is the best method for concatenating strings in Python?
The best method for concatenating strings in Python is using thejoin()
method, especially when combining multiple strings from a list. -
Are f-strings faster than other string formatting methods?
Yes, f-strings are generally faster and more readable than other string formatting methods, such as the%
operator orstr.format()
. -
When should I use StringIO in Python?
You should useStringIO
when you need to build large strings or perform extensive string manipulations, as it minimizes memory usage and improves performance. -
Can I use StringBuilder in Python?
While there is no direct equivalent of StringBuilder in Python, the methods discussed in this article, such asjoin()
andStringIO
, provide similar functionality. -
Is string concatenation inefficient in Python?
Yes, string concatenation using the+
operator can be inefficient for multiple concatenations, as it creates new string objects for each operation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn