How to Sort Counter Based on Values in Python
- Understanding the Counter Class
-
Sorting a Counter Using the
most_common()
Method -
Using the
sorted()
Function for Custom Sorting - Sorting a Counter with a Dictionary Comprehension
- Conclusion
- FAQ
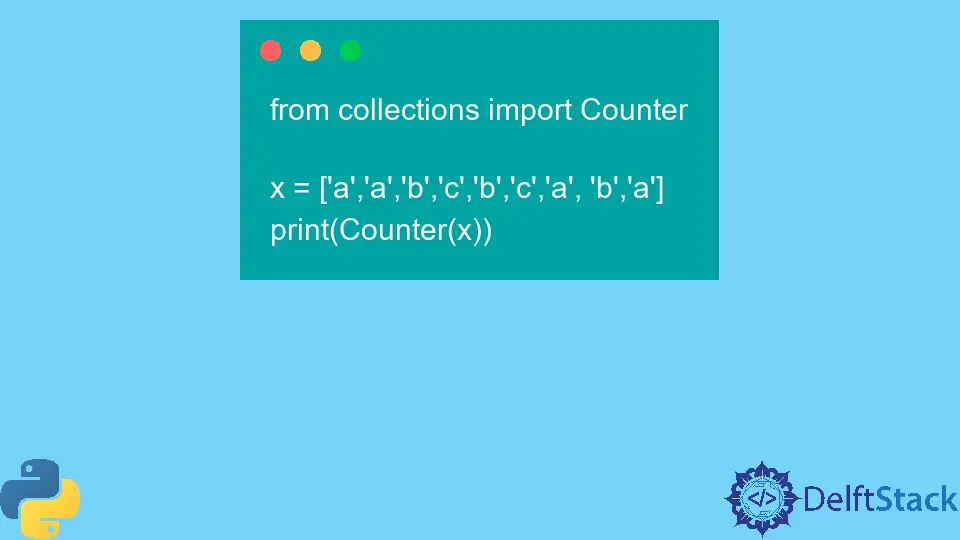
Sorting data is a common task in programming, and when working with collections, Python’s Counter
class from the collections
module simplifies counting hashable objects. However, you might often need to sort these counters based on their values for better analysis or presentation.
In this tutorial, we will explore various methods to sort a Counter
object by its values, enabling you to extract meaningful insights from your data. Whether you are a beginner or an experienced Pythonista, this guide will provide clear examples and explanations to help you master this essential skill.
Understanding the Counter Class
Before diving into sorting, let’s briefly understand what a Counter
is. A Counter
is a subclass of the dictionary that counts hashable objects. It is especially useful for tallying occurrences of items in a list or string. For instance, if you have a list of fruits and want to know how many of each type you have, a Counter
can provide that information quickly and efficiently.
Here’s a simple example of creating a Counter
:
from collections import Counter
fruits = ['apple', 'banana', 'apple', 'orange', 'banana', 'apple']
fruit_counter = Counter(fruits)
print(fruit_counter)
Output:
Counter({'apple': 3, 'banana': 2, 'orange': 1})
In this example, we created a Counter
that counts the occurrences of each fruit in the list. Now that we have a Counter
, let’s explore how to sort it based on its values.
Sorting a Counter Using the most_common()
Method
One of the simplest ways to sort a Counter
based on its values is by using the most_common()
method. This method returns a list of the n most common elements and their counts from the most common to the least. If you don’t specify n, it returns all elements.
Here’s how to use it:
sorted_fruits = fruit_counter.most_common()
print(sorted_fruits)
Output:
[('apple', 3), ('banana', 2), ('orange', 1)]
The most_common()
method sorts the Counter
in descending order by default. In our example, it lists ‘apple’ first, followed by ‘banana’ and ‘orange’. This method is straightforward and efficient, especially when you need the most common items quickly.
If you want to sort in ascending order, you can use slicing:
sorted_fruits_asc = sorted(fruit_counter.items(), key=lambda x: x[1])
print(sorted_fruits_asc)
Output:
[('orange', 1), ('banana', 2), ('apple', 3)]
This code snippet sorts the items based on their counts in ascending order. Here, we used the sorted()
function along with a lambda function to specify that we want to sort by the second element of each tuple, which represents the count.
Using the sorted()
Function for Custom Sorting
Another effective way to sort a Counter
is to use the built-in sorted()
function. This method gives you more flexibility, allowing you to sort based on various criteria. You can sort in ascending or descending order and even customize your sorting logic.
Here’s an example of sorting a Counter
in descending order based on its values:
sorted_fruits_desc = sorted(fruit_counter.items(), key=lambda item: item[1], reverse=True)
print(sorted_fruits_desc)
Output:
[('apple', 3), ('banana', 2), ('orange', 1)]
In this code, we used the sorted()
function to sort the items of the Counter
. The key
parameter is set to a lambda function that specifies we want to sort by the second item of the tuples (the counts). The reverse=True
argument sorts the items in descending order.
If you want to sort in ascending order, simply omit the reverse
argument or set it to False
:
sorted_fruits_asc = sorted(fruit_counter.items(), key=lambda item: item[1])
print(sorted_fruits_asc)
Output:
[('orange', 1), ('banana', 2), ('apple', 3)]
This flexibility makes the sorted()
function a powerful tool for sorting Counter
objects based on various criteria.
Sorting a Counter with a Dictionary Comprehension
If you prefer a more Pythonic approach, you can use a dictionary comprehension to create a new sorted dictionary from your Counter
. This method allows you to maintain the order of items while also filtering them if necessary.
Here’s how you can achieve this:
sorted_fruit_dict = {k: v for k, v in sorted(fruit_counter.items(), key=lambda item: item[1])}
print(sorted_fruit_dict)
Output:
{'orange': 1, 'banana': 2, 'apple': 3}
In this example, we used a dictionary comprehension to create a new dictionary from the sorted items of the Counter
. The resulting dictionary is sorted in ascending order based on the counts. This method is particularly useful if you want to create a new data structure while sorting.
By using dictionary comprehensions, you can also easily apply conditions to filter out unwanted items. For instance, if you only want to include fruits with a count greater than one, you could modify the comprehension:
filtered_sorted_fruit_dict = {k: v for k, v in sorted(fruit_counter.items(), key=lambda item: item[1]) if v > 1}
print(filtered_sorted_fruit_dict)
Output:
{'banana': 2, 'apple': 3}
This approach provides both sorting and filtering in a clean, readable manner.
Conclusion
Sorting a Counter
based on its values in Python is a straightforward process that can be accomplished using various methods. Whether you prefer the simplicity of the most_common()
method, the flexibility of the sorted()
function, or the elegance of dictionary comprehensions, Python offers you the tools to efficiently organize your data. By mastering these techniques, you can enhance your data analysis capabilities and make your code more robust and readable.
FAQ
-
What is a Counter in Python?
A Counter is a subclass of the dictionary that counts hashable objects, useful for tallying occurrences in a collection. -
How do I sort a Counter in descending order?
You can use themost_common()
method or thesorted()
function with thereverse
parameter set toTrue
. -
Can I filter items while sorting a Counter?
Yes, you can use dictionary comprehensions to filter items while sorting based on specific criteria. -
Is there a built-in method to sort a Counter by keys?
While themost_common()
method sorts by values, you can sort by keys using thesorted()
function with the key set to the first item of the tuples. -
Can I sort a Counter in ascending order?
Yes, both themost_common()
method (with slicing) and thesorted()
function can be used to sort a Counter in ascending order.