Python의 값을 기준으로 카운터 정렬
Preet Sanghavi
2023년6월21일
Python
Python Sorting
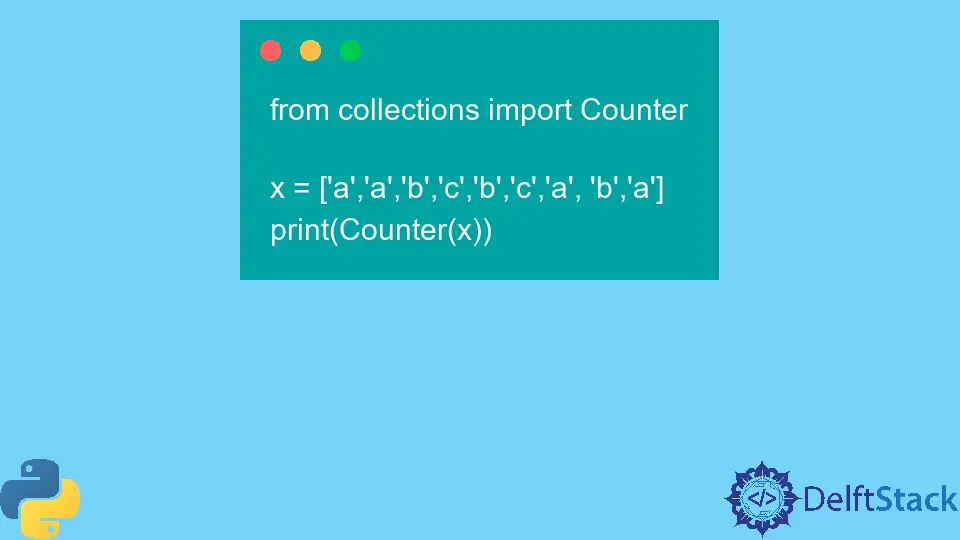
이 튜토리얼은 Python을 사용하여 값을 기준으로 카운터
를 정렬하는 방법을 보여줍니다.
Python의 카운터
개요
카운터
는 특정 문자의 총 발생 횟수를 계산하는 데 도움이 되는 Python의 컬렉션
모듈의 일부입니다. 이 항목은 배열 또는 문자열의 일부일 수 있습니다.
컬렉션을 사용하여 Python에서 특정 문자의 발생 횟수를 계산하는 방법을 이해합시다. 다음 코드의 도움으로 이를 수행할 수 있습니다.
from collections import Counter
x = ["a", "a", "b", "c", "b", "c", "a", "b", "a"]
print(Counter(x))
출력:
Counter({'a': 4, 'b': 3, 'c': 2})
Python의 값을 기준으로 카운터
정렬
이제 collections
모듈을 사용하여 Python에서 Counter
를 사용하는 방법을 배웠으므로 Counter
의 값을 정렬하는 방법을 이해해 보겠습니다.
카운터
의 most_common()
기능을 사용하여 이를 수행할 수 있습니다. most_common()
함수는 주어진 데이터 구조에서 가장 많이 발생한 문자를 찾는 데 도움이 됩니다.
다음 Python 코드의 도움으로 most_common()
함수를 사용할 수 있습니다.
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common())
출력:
[('a', 4), ('b', 3), ('c', 2)]
Counter
의 most_common()
함수 출력은 내림차순으로 정렬된 값의 배열입니다.
마찬가지로 Counter
내에 least_common
기능이 있습니다. 이 함수는 개수를 가져와서 가장 적은 횟수로 나타나는 문자를 찾습니다. 다음 코드를 참조하십시오.
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common()[::-1])
출력:
[('c', 2), ('b', 3), ('a', 4)]
따라서 우리는 Python의 값을 기반으로 collections
모듈의 Counter
를 사용하여 데이터 구조의 문자를 정렬하는 방법을 성공적으로 탐색했습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Preet Sanghavi