Python の値に基づいてカウンターを並べ替える
Preet Sanghavi
2023年6月21日
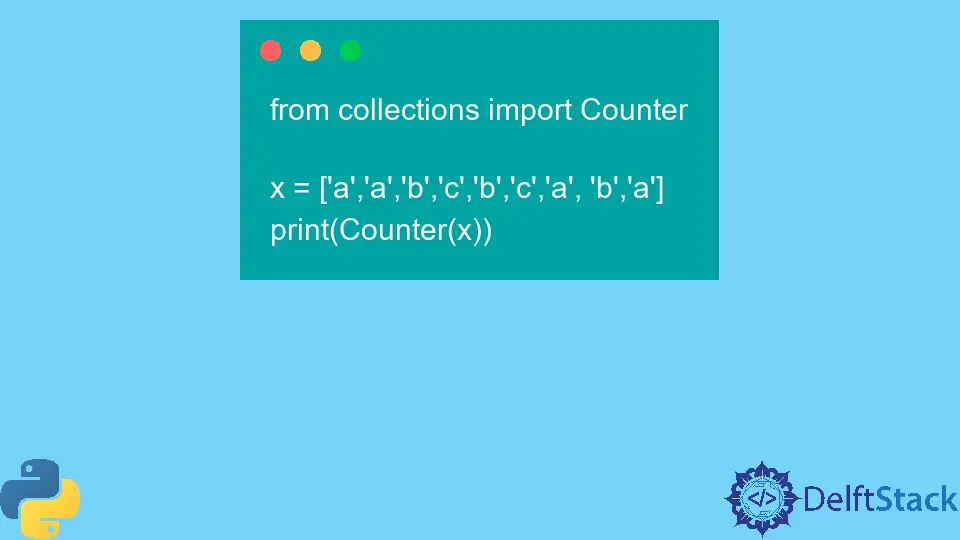
このチュートリアルでは、Python を使用してその値に基づいて Counter
を並べ替える方法を示します。
Python での Counter
の概要
Counter
は Python の collections
モジュールの一部で、特定の文字の出現回数の合計を計算するのに役立ちます。 このオカレンスは、配列または文字列の一部である可能性があります。
コレクションを使用して、Python で特定の文字の出現回数をカウントする方法を理解しましょう。 次のコードを使用してそれを行うことができます。
from collections import Counter
x = ["a", "a", "b", "c", "b", "c", "a", "b", "a"]
print(Counter(x))
出力:
Counter({'a': 4, 'b': 3, 'c': 2})
Python の値に基づいて Counter
を並べ替える
collections
モジュールを使用して Python で Counter
を使用する方法を学習したので、Counter
の値をソートする方法を理解してみましょう。
Counter
の most_common()
関数の助けを借りてそれを行うことができます。 most_common()
関数は、特定のデータ構造で最も頻繁に出現する文字を見つけるのに役立ちます。
次の Python コードの助けを借りて most_common()
関数を利用できます。
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common())
出力:
[('a', 4), ('b', 3), ('c', 2)]
Counter
の most_common()
関数の出力は、降順でソートされた値の配列であることに注意してください。
同様に、Counter
内に least_common
関数があります。 この関数はカウントを取得し、出現回数が最も少ない文字を見つけます。 次のコードを参照してください。
from collections import Counter
x = Counter(["a", "a", "b", "c", "b", "c", "a", "b", "a"])
print(x.most_common()[::-1])
出力:
[('c', 2), ('b', 3), ('a', 4)]
このように、Python での値に基づいて collections
モジュールから Counter
を使用してデータ構造の文字をソートする方法を調べることに成功しました。
著者: Preet Sanghavi