How to Slice a Dictionary in Python
- Dictionaries in Python
- Slice a Dictionary in Python Using Dictionary Comprehension
- Slice a Dictionary in Python Using List Comprehension
-
Slice a Dictionary in Python Using the
collections.ChainMap
Structure -
Slice a Dictionary in Python Using
filter
andlambda
- Conclusion
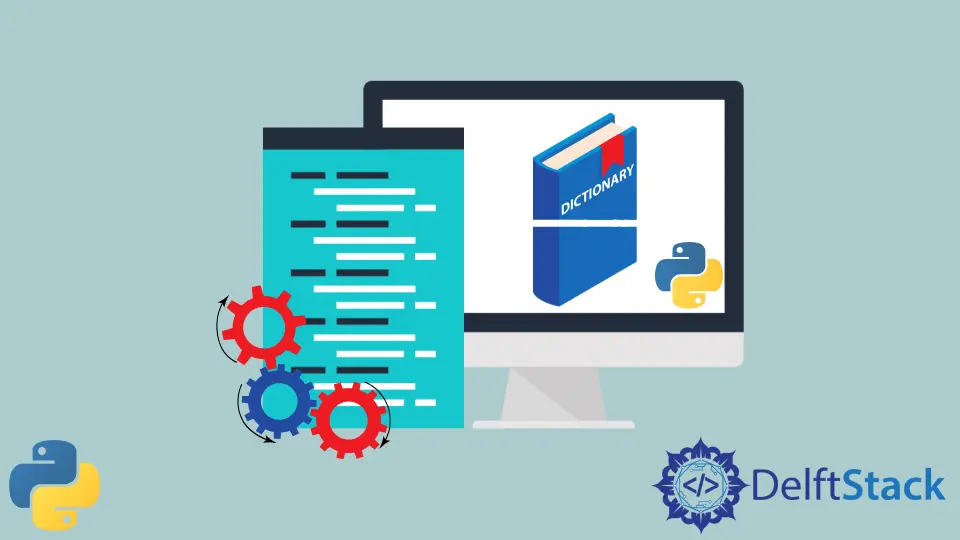
Python, with its versatile and powerful features, provides different ways to manipulate data structures. Among them, dictionaries are a fundamental tool for storing and organizing key-value pairs.
While dictionaries offer efficient methods for accessing and modifying elements, slicing—a common operation on sequences like lists and strings—can be a bit elusive when it comes to dictionaries.
In this article, we’ll explore how to slice a dictionary in Python, exploring techniques such as dictionary comprehension, list comprehension, collections.ChainMap
, and using filter
with lambda
.
Dictionaries in Python
Before diving into slicing, let’s have a quick recap of dictionaries.
In Python, a dictionary is an unordered collection of key-value pairs where each key must be unique. It allows for fast retrieval of values based on their associated keys.
The syntax for creating a dictionary is straightforward:
my_dict = {"key1": "value1", "key2": "value2", "key3": "value3"}
Slice a Dictionary in Python Using Dictionary Comprehension
One efficient method for slicing a dictionary in Python is through dictionary comprehension. Dictionary comprehension is a concise and elegant way to create dictionaries in Python.
It allows you to create a new dictionary by specifying the key-value pairs using a single line of code. This feature is not only useful for creating dictionaries but can also be employed for slicing existing dictionaries.
The basic syntax of dictionary comprehension is as follows:
{key_expression: value_expression for key, value in iterable if condition}
Where:
key_expression
: Expression to determine the keys of the new dictionary.value_expression
: Expression to determine the values of the new dictionary.iterable
: Iterable object (e.g., another dictionary, list, or any iterable) that provides key-value pairs.condition
(optional): A condition that filters which key-value pairs are included in the new dictionary.
Consider a scenario where we have a dictionary, original_dict
, and we want to extract a subset of key-value pairs based on a list of desired keys. Let’s use dictionary comprehension for this task.
import json
original_dict = {
"C": 1.1,
"O": True,
"M": "HelloWorld",
"P": {"X": 1, "Y": 2, "Z": 3},
"U": 25,
"T": None,
"E": ["Python", "Is", "Beautiful"],
"R": [1, 2, 100, 200],
}
desired_keys = ["C", "P", "U"]
sliced_dict = {
key: original_dict[key] for key in original_dict.keys() if key in desired_keys
}
print(json.dumps(sliced_dict, indent=4))
In this example, we have an existing dictionary, original_dict
. This dictionary contains a variety of key-value pairs, including numeric values, Boolean, strings, nested dictionaries, and lists.
The objective of the code is to slice this dictionary and create a new one, referred to as sliced_dict
, which includes only the key-value pairs corresponding to a specific set of desired keys.
The key component of this code is the dictionary comprehension. The comprehension iterates over the keys of original_dict
using the original_dict.keys()
method.
sliced_dict = {
key: original_dict[key] for key in original_dict.keys() if key in desired_keys
}
For each key in this iteration, it checks if the key is present in the desired_keys
list using the condition if key in desired_keys
. If the condition is met, it includes the key-value pair in the new dictionary sliced_dict
, with the key mapped to its corresponding value from original_dict
.
The resulting sliced_dict
effectively filters out the key-value pairs from the original_dict
based on the specified criteria. The final output is a new dictionary containing only the selected key-value pairs.
In this case, sliced_dict
will include the key-value pairs associated with the keys C
, P
, and U
from the original_dict
.
Output:
{
"C": 1.1,
"P": {
"X": 1,
"Y": 2,
"Z": 3
},
"U": 25
}
Dictionary comprehension provides a concise and expressive way to slice dictionaries in Python, allowing you to create new dictionaries based on specific criteria.
Slice a Dictionary in Python Using List Comprehension
While dictionary comprehension is a powerful technique for creating or slicing dictionaries, list comprehension offers another approach for achieving similar results.
List comprehension is a concise and efficient way to create lists in Python. It allows you to create a new list by specifying the elements using a single line of code.
Although list comprehension is commonly associated with creating lists, it can also be applied for slicing dictionaries when needed.
The syntax for list comprehension when slicing a dictionary is as follows:
[(key, value) for key, value in iterable if condition]
Where:
(key, value)
: Tuple expression representing a key-value pair.iterable
: Iterable object (e.g., another dictionary, list, or any iterable) providing key-value pairs.condition
(optional): A condition that filters which key-value pairs are included in the list.
Let’s explore how list comprehension can be used to slice a dictionary based on certain conditions. Consider a scenario where we have a dictionary, original_dict
, and we want to obtain a list of tuples containing key-value pairs based on a list of desired keys.
import json
original_dict = {
"C": 1.1,
"O": True,
"M": "HelloWorld",
"P": {"X": 1, "Y": 2, "Z": 3},
"U": 25,
"T": None,
"E": ["Python", "Is", "Beautiful"],
"R": [1, 2, 100, 200],
}
desired_keys = ["C", "P", "U"]
sliced_list = [
(key, original_dict[key]) for key in original_dict.keys() if key in desired_keys
]
print(json.dumps(sliced_list, indent=4))
In the Python code above, we begin by defining the original_dict
with its assortment of key-value pairs.
We then declare a list named desired_keys
, which contains the keys we are interested in. In this example, the keys C
, P
, and U
are the desired ones.
The core component of this code lies in the list comprehension, which iterates over the keys of original_dict
using original_dict.keys()
.
sliced_list = [
(key, original_dict[key]) for key in original_dict.keys() if key in desired_keys
]
For each key in this iteration, it checks if the key is present in the desired_keys
list using the condition if key in desired_keys
. If the condition is satisfied, a tuple (key, original_dict[key])
is created and included in the resulting list, sliced_list
.
The resulting sliced_list
is a concise representation of the selected key-value pairs. Each tuple in the list corresponds to a key from original_dict
that matches the specified criteria, and the tuple includes both the key and its associated value.
Output:
[
[
"C",
1.1
],
[
"P",
{
"X": 1,
"Y": 2,
"Z": 3
}
],
[
"U",
25
]
]
Here, each tuple in the list corresponds to a key-value pair from original_dict
where the key is present in the desired_keys
list. List comprehension provides an efficient and expressive way to slice dictionaries in Python when the desired output is a list of tuples.
Slice a Dictionary in Python Using the collections.ChainMap
Structure
Python’s collections
module provides a powerful data structure called ChainMap
, a module introduced in Python 3.3, which allows developers to combine multiple dictionaries into a single view. This class provides an elegant solution for slicing and manipulating dictionaries without modifying the original data.
collections.ChainMap
is part of the Python standard library and allows for easy combining and slicing of dictionaries. It represents a view over a list of dictionaries, treating them as a single mapping.
The order of dictionaries in the list determines the lookup priority.
The basic syntax of collections.ChainMap
involves creating an instance with a list of dictionaries:
import collections
sliced_dict = dict(collections.ChainMap(dict1, dict2, ...))
Where:
dict1
,dict2
, …: Dictionaries to be combined and sliced.
Consider a scenario where we have a dictionary, original_dict
, and we want to create a new dictionary, sliced_dict
, containing only the key-value pairs corresponding to a specific set of desired keys. Let’s use collections.ChainMap
for this task.
import json
import collections
original_dict = {
"C": 1.1,
"O": True,
"M": "HelloWorld",
"P": {"X": 1, "Y": 2, "Z": 3},
"U": 25,
"T": None,
"E": ["Python", "Is", "Beautiful"],
"R": [1, 2, 100, 200],
}
desired_keys = ["C", "P", "U"]
sliced_dict = dict(
collections.ChainMap({key: original_dict[key] for key in desired_keys})
)
print(json.dumps(sliced_dict, indent=4))
Here, we start by importing the collections
module, which includes the ChainMap
class. We then declare the original_dict
and a list named desired_keys
, which represents the keys we wish to include in our sliced dictionary.
The key part of this code is in the single line that employs a dictionary comprehension to create a new dictionary with key-value pairs selected from original_dict
based on the desired_keys
list. This newly created dictionary is then passed as an argument to collections.ChainMap
.
To finalize the process, we wrap the result in dict()
to convert the ChainMap
view into an actual dictionary assigned to the variable sliced_dict
.
Output:
{
"C": 1.1,
"P": {
"X": 1,
"Y": 2,
"Z": 3
},
"U": 25
}
Here, sliced_dict
contains only the key-value pairs associated with the keys C
, P
, and U
from the original_dict
.
The use of collections.ChainMap
is advantageous because it avoids the creation of a completely new dictionary, providing a memory-efficient solution. Instead, it creates a dynamic view that acts as a combined representation of the original dictionary and the newly created one.
This allows updates to either dictionary to be reflected in the ChainMap
view. Overall, this approach is particularly useful when dealing with large dictionaries or when frequent updates are expected.
Slice a Dictionary in Python Using filter
and lambda
In Python, the filter
function, combined with the power of anonymous functions (lambda functions), provides a concise and expressive way to slice dictionaries based on specific conditions.
The filter
function in Python is used to construct an iterator from elements of an iterable for which a function returns true. When combined with lambda functions, which are small, anonymous functions, you can concisely create a filtering condition.
The basic syntax of filter
involves passing a filtering function and an iterable:
filtered_items = filter(lambda item: condition, iterable)
Where:
lambda item: condition
: A lambda function defining the condition for filtering.iterable
: Iterable object providing key-value pairs.
Let’s explore how filter
and lambda
can be used to slice a dictionary. Consider the same scenario that we used in the previous examples:
import json
original_dict = {
"C": 1.1,
"O": True,
"M": "HelloWorld",
"P": {"X": 1, "Y": 2, "Z": 3},
"U": 25,
"T": None,
"E": ["Python", "Is", "Beautiful"],
"R": [1, 2, 100, 200],
}
desired_keys = ["C", "P", "U"]
filtered_items = filter(lambda item: item[0] in desired_keys, original_dict.items())
sliced_dict = dict(filtered_items)
print(json.dumps(sliced_dict, indent=4))
In the example code above, we start by defining the original_dict
and a list named desired_keys
. The filter
function is then applied to original_dict.items()
, which provides a view of the key-value pairs.
The lambda
function, lambda item: item[0] in desired_keys
, is used as the filtering condition. This lambda function checks if the key (item[0]) of each key-value pair is present in the list of desired_keys
.
The filter
function returns an iterable of filtered items, and we convert this iterable back into a dictionary using the dict()
constructor, resulting in the creation of sliced_dict
. This concise approach effectively filters the key-value pairs based on the desired keys, providing a new dictionary with only the selected items.
Output:
{
"C": 1.1,
"P": {
"X": 1,
"Y": 2,
"Z": 3
},
"U": 25
}
Here, sliced_dict
contains only the key-value pairs associated with the keys C
, P
, and U
from the original_dict
.
This method is particularly useful when the filtering condition is dynamic or needs to be more complex than a simple dictionary comprehension. It showcases the versatility and expressiveness of using filter
and lambda
for dictionary slicing in Python.
Note: All the examples discussed above use the
json
module to print the dictionary’s output beautifully. Note that thejson
module has nothing to do with the actual logic for slicing a dictionary, and it is entirely optional to use this module.
Conclusion
Python offers various methods to slice a dictionary based on specific criteria, providing flexibility and efficiency in data manipulation. Whether using dictionary comprehension, list comprehension, collections.ChainMap
, or the combination of filter
and lambda
, Python provides a versatile set of tools to extract and manipulate data from dictionaries efficiently.
The choice of method depends on factors such as readability, memory efficiency, and the complexity of the slicing criteria. Understanding these diverse approaches equips you with the flexibility to adapt your code to different requirements, making dictionary manipulation a powerful aspect of Python programming.