How to Right Justify String in Python
- Use Format Specifiers to Right Justify Strings in Python
-
Use
f-strings
to Right Justify Strings in Python -
Use the
format()
Method to Right Justify Strings in Python -
Use the
rjust()
Method to Right Justify Strings in Python - Conclusion
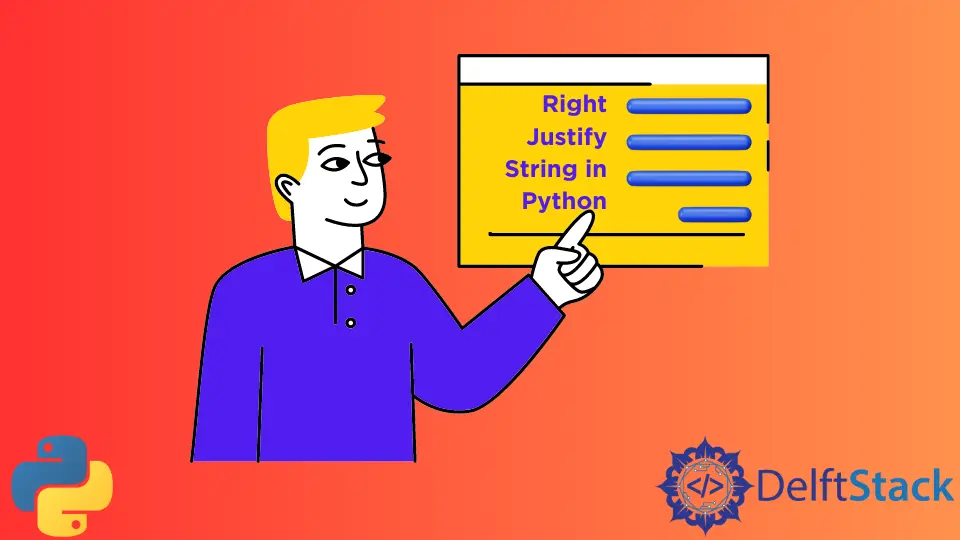
Sometimes, while printing tables in python, we need to justify values to look good.
In this article, we’ll discuss different ways to right justify a string in python. To understand different text alignment techniques, including right justification, see Printing with Column Alignment in Python.
Use Format Specifiers to Right Justify Strings in Python
Format specifiers, as the name suggests, are used for formatting values in a string. The following is the syntax for using format specifiers in python.
myStr = "%s" % (string_variable)
- The
string_variable
is the variable’s name that needs to be printed as a string. %s
is used as a format specifier and placeholder for a string variable. For integers and floating-point numbers, you can use%d
and%f
as the format specifier.
When the above statement is executed, the value in string_variable
is assigned at the place of the format specifier in the string. Finally, the output string is assigned to myStr
.
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "%s %s %s" % (string_variable1, string_variable2, string_variable3)
print(myStr)
Output:
Delftstack Aditya Jinku
In the example above, we defined three string variables. After that, we converted the inputs into a single string using format specifiers.
In this next example, suppose there are fewer variables than the number of format specifiers in the string. In that case, the program will run into a TypeError
exception saying that there aren’t enough arguments given to the format string.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "%s %s %s" % (string_variable1, string_variable2)
print(myStr)
Output:
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 4, in <module>
myStr = "%s %s %s" % (string_variable1, string_variable2)
TypeError: not enough arguments for format string
There are three format specifiers in the code above. However, we have only given two string variables as input arguments to the %
operator.
Because of this, the program runs into a TypeError
exception. Similarly, when there are more variables in the format string than the number of format specifiers, the program runs into a TypeError
exception saying that all the arguments to the format string haven’t been converted during string formatting.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "%s %s" % (string_variable1, string_variable2, string_variable3)
print(myStr)
Output:
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 4, in <module>
myStr = "%s %s" % (string_variable1, string_variable2, string_variable3)
TypeError: not all arguments converted during string formatting
In the above example, there are only two format specifiers, but we have given three string variables as input arguments to the %
operator. Due to this, the program runs into the TypeError
exception while executing.
To right-justify the output strings, we use the length of the output string with the format specifier, as shown in the following snippet.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "%10s" % (string_variable1)
myStr2 = "%10s" % (string_variable2)
myStr3 = "%10s" % (string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
We have specified the output string length in the above example as 10
. After executing, the output strings will be of length 10
.
Output:
Delftstack
Aditya
Jinku
Observe in the output that the strings are right justified. If the length of the string_variable
is less than the length of the output string specified in the format specifier, space characters are padded to the string to right-justify the input string.
If the length of the string_variable
is greater than the length of the output string specified in the format specifier, no change happens to the input string and is printed unchanged.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "%5s" % (string_variable1)
myStr2 = "%5s" % (string_variable2)
myStr3 = "%5s" % (string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
We specified the output string length to be 5
. However, all the strings we passed as input are of length greater than or equal to 5
.
Hence, the specified length of the output string is less than or equal to the length of the input strings. Therefore, no formatting occurs.
Use f-strings
to Right Justify Strings in Python
Using format specifiers requires us to know the datatype of variables to be able to print them. If we don’t pass the variable with the correct data type specified in the format specifier, the program runs into a TypeError
exception.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "%d" % (string_variable1)
myStr2 = "%5s" % (string_variable2)
myStr3 = "%5s" % (string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 4, in <module>
myStr1 = "%d" % (string_variable1)
TypeError: %d format: a number is required, not str
You can see that we used %d
as the format specifier. However, we have passed a string as the input to the %
operator.
Due to this, the program runs into a TypeError
exception saying that it expects an integer input and not a string. To avoid this error, we can use f-strings to right justify the strings.
The syntax for using f-strings
:
myStr = f"{string_variable}"
- The
string_variable
is the variable whose value must be printed. {}
is used as a placeholder for thestring_variable
.- The literal
"f"
before the string shows that the string is an f-string.
After execution, the output string is assigned to myStr
.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = f"{string_variable1}"
myStr2 = f"{string_variable2}"
myStr3 = f"{string_variable3}"
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
We created three variables in the above example. After that, we’ve created three f-strings
corresponding to each variable.
After execution of the f-strings, the outputs are assigned to variables myStr1
, myStr2
, and myStr3
. To right-justify the output string in the above code, we use the length of the output string in the placeholder, as shown in the following example.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = f"{string_variable1:>10}"
myStr2 = f"{string_variable2:>10}"
myStr3 = f"{string_variable3:>10}"
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
We have used the syntax variable_name:> output_length
in the placeholder to right justify the strings in the output. We have specified the output_length
to be 10
.
If the length of the string_variable
is less than the length of the output string specified in the placeholder, space characters are padded to the string to right justify the input string. Like in the following snippet, If the length of the string_variable
is greater than the length of the output string specified in the placeholder, no change happens to the input string, and it is printed unchanged.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = f"{string_variable1:>5}"
myStr2 = f"{string_variable2:>5}"
myStr3 = f"{string_variable3:>5}"
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
We specified the length of the output string to be 5
, which is less than or equal to the length of all the input strings. Therefore, no formatting happens.
Use the format()
Method to Right Justify Strings in Python
Instead of f-strings
, we can also use the format()
method to right justify strings in python.
The syntax for using the format()
method:
myStr = "{}".format(string_variable)
string_variable
is the name of the variable that needs to be printed as a string.{}
is used as a placeholder for a string variable.
When the format()
method is executed, the value in string_variable
is assigned at the place of the placeholder in the string. Finally, the output string is assigned to myStr
.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "{}".format(string_variable1)
myStr2 = "{}".format(string_variable2)
myStr3 = "{}".format(string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
In the above example, we created three variables. After that, we have used the format()
method three times, once for each variable.
The outputs are assigned to variables myStr1
, myStr2
, and myStr3
after executing the format()
method.
Let’s have an example where you can have any number of placeholders with the same number of variables as an input argument to the format()
method.
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "{} {} {}".format(string_variable1, string_variable2, string_variable3)
print(myStr)
Output:
Delftstack Aditya Jinku
We passed all three variables as input arguments to the format()
method. The output is returned as a single string.
If fewer variables are passed to the format()
method than the number of placeholders in the string, the program will run into an IndexError
exception, like in the following example.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "{} {} {}".format(string_variable1, string_variable2)
print(myStr)
Output:
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 4, in <module>
myStr = "{} {} {}".format(string_variable1,string_variable2)
IndexError: Replacement index 2 out of range for positional args tuple
See that there are three placeholders, but we have passed only two strings as input arguments to the format()
method. Due to this, the program runs into the IndexError
exception.
When the number of variables passed to the format()
method is more than the placeholders in the string on which the format()
method is invoked, the variables equal to the number of placeholders are included in the string. The rest of the variables are discarded.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr = "{} {}".format(string_variable1, string_variable2, string_variable3)
print(myStr)
Output:
Delftstack Aditya
You can see that there are only two placeholders, and we have passed three strings as input to the format()
method, but the program doesn’t run into the IndexError
this time. Only the last input argument has been discarded.
To right-justify the output string using the format()
method, we use the length of the output string in the placeholder, as shown in the following example.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "{:>10}".format(string_variable1)
myStr2 = "{:>10}".format(string_variable2)
myStr3 = "{:>10}".format(string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
Here, if the length of the string_variable
is less than the length of the output string specified in the placeholder, space characters are padded to the string to right justify the input string.
If the length of the string_variable
is greater than the length of the output string specified in the placeholder, no change happens to the input string and is printed unchanged.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = "{:>5}".format(string_variable1)
myStr2 = "{:>5}".format(string_variable2)
myStr3 = "{:>5}".format(string_variable3)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
All the approaches discussed above are used to format variables with every data type. Python also provides us with methods specific to strings.
We can use the rjust()
method to right justify strings in python.
Use the rjust()
Method to Right Justify Strings in Python
The syntax for the rjust()
method:
myStr = string_variable.rjust(output_length)
string_variable
is the variable whose value needs to be right-justified.- The
ouput_length
is the length of the output string.
When the rjust()
method is invoked on the string_variable
, it takes output_length
as its input argument and returns the right-justified string, which is assigned to myStr
.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = string_variable1.rjust(10)
myStr2 = string_variable2.rjust(10)
myStr3 = string_variable3.rjust(10)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
If the string_variable
length is less than the output_length
, space characters are padded to the string to right justify the input string.
In this next example, if the length of the string_variable
is greater than output_length
, no change happens to the input string, and it is printed unchanged.
Example Code:
string_variable1 = "Delftstack"
string_variable2 = "Aditya"
string_variable3 = "Jinku"
myStr1 = string_variable1.rjust(5)
myStr2 = string_variable2.rjust(5)
myStr3 = string_variable3.rjust(5)
print(myStr1)
print(myStr2)
print(myStr3)
Output:
Delftstack
Aditya
Jinku
Conclusion
In this article, we’ve discussed four ways to right justify a string in python. Out of all these, I would suggest you use the approach using the format()
method as it is more convenient.
Also, the program will not run into an error if the input variable contains values of other data types in this case.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub