How to Replace Character in a String in Python
-
Use the
list()
andjoin()
Function to Replace a Character in a String -
Use the
bytearray()
Function to Replace a Character in a String -
Use the
replace()
Function to Replace Characters in a String - Use the String Concatenation Method to Replace a Character in a String
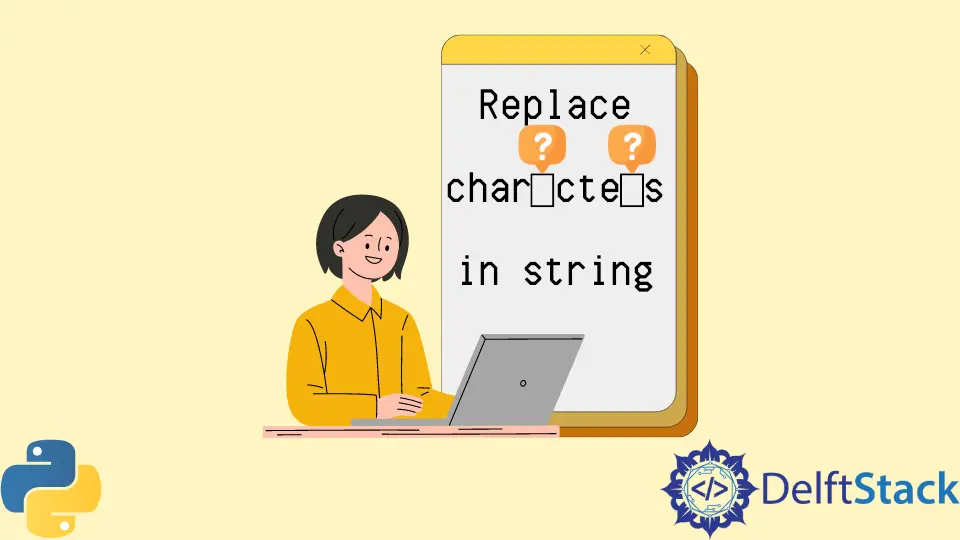
A string is a collection of characters. In Python, strings are immutable. It means that it is impossible to modify such objects and only be reassigned, or a copy can be created.
Due to this, it is not simple to replace characters in a string. If we are dealing with a mutable object, we can easily change its elements, as we can in a list as shown below.
l = ["a", "b", "c"]
l[1] = "d"
print(l)
Output:
['a', 'd', 'c']
We don’t do the same to a string. In this tutorial, we will learn how to replace a character in a string.
Use the list()
and join()
Function to Replace a Character in a String
In this method, we convert the string to a list of characters using the list()
function. We change the necessary character from this list. Then we combine the whole list to a single string using the join()
function.
The following code snippet implements this.
s = "Naze"
l = list(s)
l[2] = "m"
s = "".join(l)
print(s)
Output:
Name
This is a simple, efficient method, although it is considered a little slow.
Use the bytearray()
Function to Replace a Character in a String
A byte array is a mutable object in Python. It is an array of bytes. We can convert the string to a byte array using the bytearray()
function, make the necessary replacements and then convert it back to a string using the str()
function.
For example,
s = "Naze"
b = bytearray(s)
b[2] = "m"
s = str(b)
print(s)
Output:
Name
Note that this method fails in Python 3.x and up since we need to encode the string in the recent versions.
Use the replace()
Function to Replace Characters in a String
Python is very well equipped with many functions for working with strings. We can use the replace()
function to change characters in a string. We have to specify the old and new characters in the function.
The following code demonstrates the use of this function.
s = "Naze"
new_s = s.replace("z", "m")
print(new_s)
Output:
Name
Note that this method replaces all the occurrences of the old character with the new one.
Use the String Concatenation Method to Replace a Character in a String
If we know the character’s position that we want to change, then this method can be very useful and fast. We divide the string till that part, add the character, and then concatenate it with the remaining portion of the string that is left.
The following code snippet implements this.
s = "Naze"
new_s = s[:2] + "m" + s[3:]
print(new_s)
Output:
Name
Note that this is the fastest of all the methods.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn