How to Remove Special Characters From the String in Python
-
Remove Special Characters From the String in Python Using the
str.isalnum()
Method -
Remove Special Characters From the String in Python Using
filter(str.isalnum, string)
Method - Remove Special Characters From the String in Python Using Regular Expression
-
Remove Special Characters From the String in Python Using
str.translate()
andstr.maketrans()
Methods -
Remove Special Characters From the String in Python Using
map()
and Lambda Functions - Example Code:
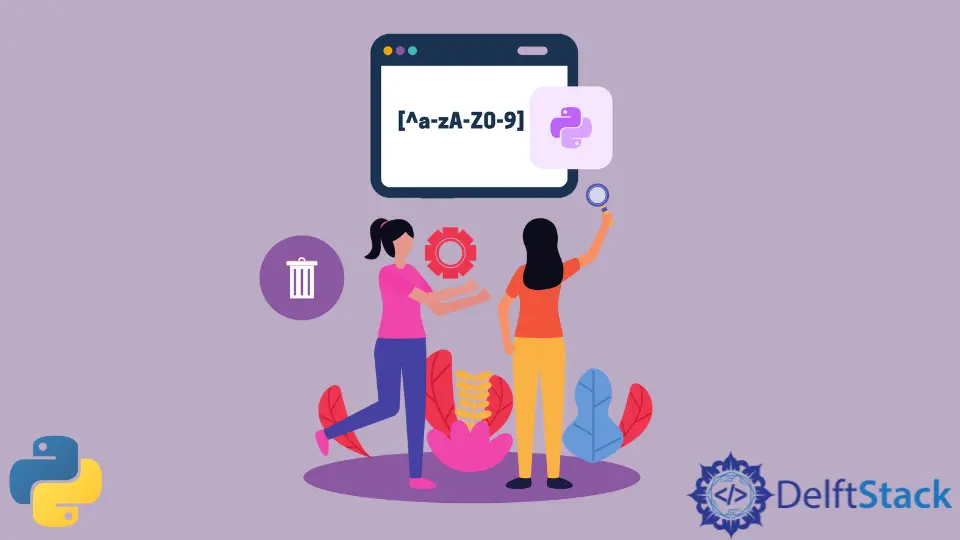
In this tutorial, we will discuss various ways to remove all the special characters from the string in Python. We can remove the special characters from the string by using functions or regular expressions.
Removing special characters from strings is a common task to ensure that text data is clean and suitable for further processing. We’ll cover below methods for achieving this goal.
- Using
str.isalnum()
andstr.join()
methods. - Using the
filter()
function. - Using regular expressions (
re
module). - Using
str.translate()
andstr.maketrans()
methods. - Using
map()
and lambda functions
Remove Special Characters From the String in Python Using the str.isalnum()
Method
The str.isalnum()
method is a powerful tool to help us identify whether a character is alphanumeric or not, and the str.join()
method allows us to reconstruct the cleaned string.
Let’s use the string "Hey! What's up bro?"
as our example string for this article.
Here’s a complete code example that demonstrates how to remove special characters from a string using the str.isalnum()
method and the str.join()
method:
# Example string with special characters
original_string = "Hey! What's up bro?"
# Step 1: Remove special characters using list comprehension and str.isalnum()
cleaned_list = [char for char in original_string if char.isalnum()]
# Step 2: Reconstruct the cleaned string using str.join()
cleaned_string = "".join(cleaned_list)
# Print the cleaned string
print("Original String:", original_string)
print("Cleaned String:", cleaned_string)
Output:
Original String: Hey! What's up bro?
Cleaned String: HeyWhatsupbro
Step-by-Step Explanation
- Step 1:
str.isalnum()
The str.isalnum()
method is a built-in string method that checks if all the characters in the given string are alphanumeric (either letters or digits). It returns True
if all characters are alphanumeric and False
otherwise.
In our example, we use a list comprehension to iterate over each character in the original string. If the character is alphanumeric, it is included in the cleaned_list
.
- Step 2:
str.join()
The str.join()
method is another string method that allows us to join a sequence of strings using a specified delimiter. In this case, we use an empty string as the delimiter to concatenate the characters in the cleaned_list
without any separation.
By applying the str.isalnum()
method and then using the str.join()
method, we efficiently remove special characters and reconstruct the cleaned string.
Remove Special Characters From the String in Python Using filter(str.isalnum, string)
Method
The filter()
function provides a powerful way to remove unwanted characters from a string based on certain criteria.
It allows us to apply a filter condition to each character and retain only those that meet the condition.
Using filter()
can result in a cleaner and more structured text dataset.
Before diving into the detailed explanation, let’s take a look at the complete code example that demonstrates how to remove special characters from a string using the filter()
function:
# Example string with special characters
original_string = "Hey! What's up bro?"
# Apply the filter() function to the string
filtered_chars = filter(str.isalnum, original_string)
# Convert the filtered characters back to a string
cleaned_string = "".join(filtered_chars)
# Print the cleaned string
print("Original String:", original_string)
print("Cleaned String:", cleaned_string)
Output:
Original String: Hey! What's up bro?
Cleaned String: HeyWhatsupbro
Step-by-Step Explanation
- Step 1: Applying
filter()
to the String
The filter()
function takes two arguments: the filtering function and the iterable (in this case, the original string). It applies the filtering function to each character in the string and returns an iterable containing only the characters that satisfy the filter condition.
In our example, we apply the str.isalnum()
function using filter(str.isalnum, original_string)
to obtain an iterable of filtered characters.
- Step 2: Converting Filtered Characters to String
The iterable obtained from the filter()
function needs to be converted back to a string. We achieve this by using the join()
method, which concatenates the characters without any delimiter. The result is the cleaned string containing only alphanumeric characters.
Remove Special Characters From the String in Python Using Regular Expression
Regular expressions, often abbreviated as regex or regexp, offer a versatile and flexible way to work with strings.
They allow you to define patterns that match specific sequences of characters, making them perfect for identifying and removing special characters from text.
Python’s built-in re
module provides functions for working with regular expressions.
Working Example Code:
import re
# Example string with special characters
original_string = "Hey! What's up bro?"
# Define the regular expression pattern for non-alphanumeric characters
pattern = r"[^a-zA-Z0-9\s]"
# Use re.sub() to replace special characters with an empty string
cleaned_string = re.sub(pattern, "", original_string)
# Print the cleaned string
print("Original String:", original_string)
print("Cleaned String:", cleaned_string)
Output:
Original String: Hey! What's up bro?
Cleaned String: Hey Whats up bro
Step-by-Step Explanation
- Step 1: Importing the
re
Module
To work with regular expressions, we need to import Python’s built-in re
module. This module provides functions for working with regular expressions, including pattern matching and replacement.
- Step 2: Defining the Regular Expression Pattern
The regular expression pattern defines the criteria for matching characters we want to remove. In this example, we define the pattern r'[^a-zA-Z0-9\s]'
, which matches any character that is not a letter, digit, or whitespace.
[^a-zA-Z0-9\s]
, this part of the pattern, matches any character that is not an uppercase or lowercase letter, a digit, or a whitespace character.
- Step 3: Using
re.sub()
to Replace Special Characters
The re.sub()
function allows us to substitute occurrences of a pattern in a string with a replacement string. In our case, we want to replace the matched special characters with an empty string, effectively removing them.
We use re.sub(pattern, replacement, string)
to perform the substitution. Here, pattern
is the regular expression pattern we defined, replacement
is an empty string (''
), and string
is the original input string.
- Step 4: Printing the Cleaned String
After applying the re.sub()
function, we obtain the cleaned string with special characters removed. We print both the original and cleaned strings to compare the results.
Remove Special Characters From the String in Python Using str.translate()
and str.maketrans()
Methods
Python’s str.translate()
method is a versatile tool for string manipulation. It’s particularly useful when you want to remove specific characters from a string or perform character-level substitutions.
The method takes advantage of the str.maketrans()
method to create a translation table, which defines the mapping of characters to be replaced or removed.
The str.translate()
method then applies this translation table to the string.
For the purpose of this article, we’ll work with the example string: “Hey! What’s up bro?”
Example Code:
# Example string with special characters
original_string = "Hey! What's up bro?"
# Define a translation table to remove special characters
special_characters = "!@#$%^&*()_-+=<>,./?;:'\"[]{}\\|`~"
translation_table = str.maketrans("", "", special_characters)
# Apply the translation table using the translate() method
cleaned_string = original_string.translate(translation_table)
# Print the cleaned string
print("Original String:", original_string)
print("Cleaned String:", cleaned_string)
Output:
Original String: Hey! What's up bro?
Cleaned String: Hey Whats up bro
Step-by-Step Explanation
- Step 1: Define the Special Characters
Next, we define a string called special_characters
that contains all the special characters we want to remove. You can customize this string to include any special characters that need to be removed from your input string.
- Step 2: Create the Translation Table
We use the str.maketrans()
method to create a translation table. This method takes three arguments: the characters to be replaced, the characters to replace them with, and a third argument that specifies characters to be removed.
In our case, we want to remove the special characters, so we provide an empty string ''
as the second argument. The third argument is the string special_characters
that we defined earlier.
The resulting translation_table
maps each special character to None
, effectively indicating that they should be removed from the string.
- Step 3: Apply the Translation using the
translate()
Method
With the translation table in place, we apply it to the original string using the translate()
method. This method applies the translation table and returns a new string with the specified character substitutions or removals.
Remove Special Characters From the String in Python Using map()
and Lambda Functions
The map()
function is a built-in Python function that applies a given function to each item of an iterable (e.g., a list, tuple, or string) and returns an iterator. When combined with a lambda function, map()
can be a concise and efficient way to perform element-wise operations on a collection.
Example Code:
# Example string with special characters
original_string = "Hey! What's up bro?"
# Define a lambda function to remove special characters
cleaned_string = "".join(
map(lambda char: char if char.isalnum() or char.isspace() else "", original_string)
)
# Print the cleaned string
print("Original String:", original_string)
print("Cleaned String:", cleaned_string)
Output:
Original String: Hey! What's up bro?
Cleaned String: Hey Whats up bro
Step-by-Step Explanation
- Step 1: Define the Lambda Function
Next, we define a lambda function that takes a character as input and returns the character if it’s alphanumeric or a space, and an empty string otherwise. The lambda function performs character-wise filtering, removing all non-alphanumeric characters except spaces.
- Step 2: Apply
map()
andjoin()
We use the map()
function to apply the lambda function to each character in the original string. The map()
function returns an iterator of the modified characters. To obtain the final cleaned string, we use the join()
method to concatenate the characters from the iterator into a single string.