How to Remove \n From the String in Python
- Understanding the Newline Character
- Method 1: Using the str.replace() Method
- Method 2: Using the str.split() and str.join() Methods
- Method 3: Using Regular Expressions
- Conclusion
- FAQ
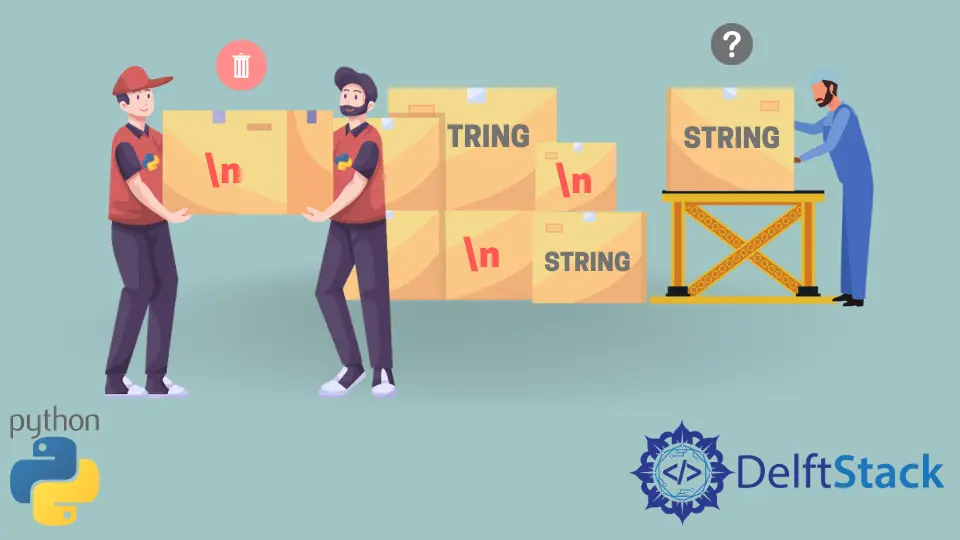
In the world of programming, handling strings is a common task, especially in Python. One frequent issue developers encounter is the presence of newline characters, represented as \n
, within strings. These characters can disrupt the flow of text and formatting, making it essential to know how to remove them effectively. Whether you’re cleaning up user input, processing data, or preparing strings for display, knowing how to eliminate these unwanted characters can enhance your code’s functionality and readability.
In this tutorial, we’ll explore various methods to remove \n
from strings in Python, providing clear examples and explanations along the way.
Understanding the Newline Character
Before diving into the methods, let’s briefly discuss what the newline character is. In Python, \n
is used to indicate the end of a line. When you print a string containing \n
, the output will break into a new line at each occurrence. While this can be useful in some contexts, it often needs to be removed for better string manipulation or display.
Method 1: Using the str.replace() Method
One of the simplest ways to remove \n
characters from a string in Python is by using the str.replace()
method. This method allows you to replace a specified substring with another substring. In our case, we want to replace \n
with an empty string.
Here’s how you can do it:
text_with_newline = "Hello,\nWorld!\nThis is a test string."
cleaned_text = text_with_newline.replace('\n', '')
print(cleaned_text)
Output:
Hello,World!This is a test string.
The str.replace()
method takes two arguments: the substring you want to replace and the substring you want to replace it with. In this example, we replaced \n
with an empty string, effectively removing it from the original string. This method is straightforward and works well for simple cases where you want to eliminate all newline characters from a string.
Method 2: Using the str.split() and str.join() Methods
Another effective way to remove newline characters is by using a combination of the str.split()
and str.join()
methods. This method is particularly useful if you want to maintain spaces between the words.
Here’s how it works:
text_with_newline = "Hello,\nWorld!\nThis is a test string."
cleaned_text = ' '.join(text_with_newline.split())
print(cleaned_text)
Output:
Hello, World! This is a test string.
In this example, the str.split()
method splits the original string into a list of words, automatically removing any whitespace characters, including \n
. Then, the str.join()
method combines the list back into a single string, using a space as the separator. This approach not only removes the newline characters but also ensures that the words are properly spaced, making it a great choice for cleaning up user input or text data.
Method 3: Using Regular Expressions
For more complex string manipulations, Python’s re
module provides powerful tools for handling patterns in strings. If you’re dealing with various whitespace characters, including \n
, \r
, or even multiple spaces, using regular expressions can be highly effective.
Here’s an example of how to use regular expressions to remove newline characters:
import re
text_with_newline = "Hello,\nWorld!\nThis is a test string."
cleaned_text = re.sub(r'\n', '', text_with_newline)
print(cleaned_text)
Output:
Hello,World!This is a test string.
In this code, we import the re
module and use the re.sub()
function to replace all occurrences of \n
with an empty string. The first argument is the pattern we want to match (\n
), the second argument is what we want to replace it with (an empty string), and the third argument is the original string. This method is particularly useful when you need to remove multiple types of whitespace or when the string’s structure is more complex.
Conclusion
Removing newline characters from strings in Python is a common task that can be accomplished in several ways. Whether you choose to use the str.replace()
method for simplicity, the combination of str.split()
and str.join()
for better spacing, or the re
module for more complex patterns, each method has its own advantages. By understanding these techniques, you can enhance your string manipulation skills and ensure your text data is clean and well-formatted.
Now that you have the tools to tackle newline characters effectively, you can apply these methods in your projects and improve your code’s readability and functionality.
FAQ
-
How do I remove only specific newline characters from a string?
You can use thestr.replace()
method to target specific newline characters or any other substring you want to remove. -
Can I remove newline characters from a list of strings?
Yes, you can use a loop or a list comprehension to apply any of the methods discussed to each string in the list. -
What if I want to replace newline characters with a space instead of removing them?
You can modify thestr.replace()
method to replace\n
with a space by changing the second argument to ’ ‘.
-
Are there performance differences between these methods?
Generally,str.replace()
is faster for simple replacements. However, for more complex patterns, regular expressions may be more efficient. -
Can I use these methods with multiline strings?
Yes, all the methods discussed can handle multiline strings effectively.