How to Remove the First Character From the String in Python
- Remove the First Character From the String in Python Using the Slicing Method
-
Remove the First Character From the String in Python Using the
str.lstrip()
Method -
Remove the First Character From the String in Python Using the
re.sub()
Method -
Remove the First Character From the String in Python Using a
for
Loop With Slicing - Conclusion
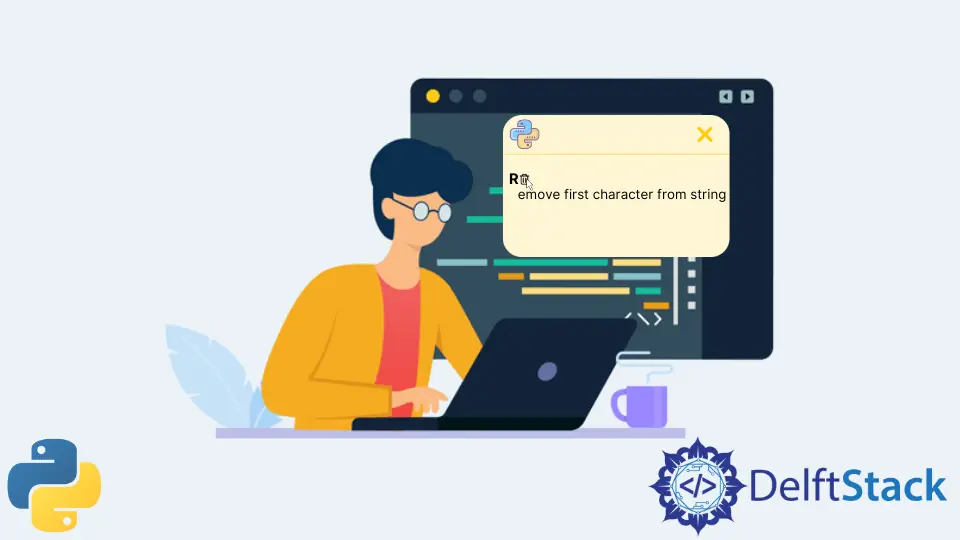
Removing the first character from a string in Python is a common task, and there are various methods to achieve it. Since strings in Python are immutable, we create a new string without the undesired character.
In this tutorial, we’ll explore multiple methods to remove the first character from the string in Python.
Remove the First Character From the String in Python Using the Slicing Method
If we want to remove the first or some specific character from the string, we can remove that character by slicing - str[1:]
. The str[1:]
gets the whole string except the first character.
The following syntax effectively removes the first character from original_string
. The result is then stored in the new_string
variable, leaving the original string unchanged.
This approach allows us to work with a modified version of the string while preserving the integrity of the original data.
Syntax:
new_string = original_string[1:]
Parameters:
original_string
: This is the original string from which you want to remove the first character.new_string
: This is the new string that will result from the slicing operation and will contain all characters except the first character fromoriginal_string
.[1:]
: This is the slicing notation. The[1:]
indicates that you want to start the slice from the second character (index1
) and include all characters up to the end of the string, and this effectively removes the first character.
For example, we need to remove the first character from the string hhello
.
string = "hhello"
new_string = string[1:]
print(new_string)
We begin with a string variable named string
set to "hhello"
. This string comprises five characters, with 'h'
as the initial character, forming the word "hello"
.
Then, we establish a new string variable, new_string
, and employ slicing with the syntax string[1:]
. This instructs Python to create new_string
by excluding the first character 'h'
from the original string and including the rest of the characters.
Finally, we print new_string
to the console using print(new_string)
.
Output:
hello
In the output, we see that the initial "h"
has been effectively removed from the original string. The modified string, housed in new_string
, now holds "hello"
.
Remove the First Character From the String in Python Using the str.lstrip()
Method
The str.lstrip()
method takes one or more characters as input, removes them from the start of the string, and returns a new string with removed characters. But be aware that the str.lstrip()
method will remove the character(s) if they occur at the start of the string one or multiple times.
The following syntax will remove specific characters from the start of original_string
and store the modified string in new_string
. The optional characters
argument grants us control over which characters to remove.
If we omit it, the method clears leading whitespace characters, like spaces and tabs. Importantly, we maintain the original string’s integrity for future use while creating a modified string in new_string
.
Syntax:
new_string = original_string.lstrip(characters)
Parameters:
new_string
: This is the variable where the resulting string with the specified characters removed from the beginning will be stored.original_string
: This is the original string from which you want to remove characters.characters
(optional): This argument specifies the characters you want to remove from the beginning of the string. If not provided, it removes leading whitespace characters.
The example code below demonstrates how we can use the str.lstrip()
method to remove character(s) from the start of the string.
string = "Hhello world"
new_string = string.lstrip("H")
print(new_string)
string1 = "HHHHhello world"
new_string1 = string1.lstrip("H")
print(new_string1)
In the code example, we utilized the .lstrip()
method in two scenarios. In the first case, we removed 'H'
characters from the left side of the string "Hhello world"
.
The second case involved a string with multiple leading 'H's
("HHHHhello world"
). Then, we use the .lstrip("H")
function to remove specific characters from the string.
Output:
hello world
hello world
In both cases, the .lstrip("H")
method effectively removes all 'H'
characters from the left side of the strings, leaving us with "hello world"
as the content of the new_string
.
Remove the First Character From the String in Python Using the re.sub()
Method
The re.sub()
method of the re
library can also be used to remove the first character from the string. The re.sub()
method replaces all the characters that match the given regular expression pattern argument with the second argument.
In the following syntax, the re.sub()
allows us to find and replace specific patterns in a string using regular expressions. We define the pattern
you want to match, provide the replacement
string, specify the original_string
, and optionally limit the number of substitutions with the count
parameter.
Syntax:
import re
new_string = re.sub(pattern, replacement, original_string, count=0)
Parameters:
pattern
: The regular expression pattern to match.replacement
: The replacement string for the matched pattern.string
: The original string in which substitutions will be made.count
(optional): The maximum number of substitutions to perform (default is0
for all occurrences).
Example code:
import re
string = "Hhello world"
new_string = re.sub(r'.', '', string, count = 1)
print(new_string)
In this code, we import the re
module to manipulate a string. We start with a string variable, string
, set to "Hhello world"
, consisting of 12 characters.
Then, we use the re.sub()
method to perform a substitution by specifying a regular expression pattern, in this case, a dot(.
), to match any character. Our goal is to remove the first character that matches the pattern.
Next, we indicate this by setting the count parameter to 1
, limiting the substitution to only one occurrence. Lastly, we saved the modified string in new_string
and printed it to the console.
Output:
hello world
In the output, the "H"
at the beginning of the string is effectively removed.
Remove the First Character From the String in Python Using a for
Loop With Slicing
Removing the first character from a string in Python using a for
loop with slicing involves iterating through the characters of the original string, starting from the second character (index 1
) to the end of the string. This effectively skips the first character, allowing you to construct a new string without it.
Syntax:
original_string = "example"
new_string = ""
for character in original_string[1:]:
new_string += character
Using a for
loop with slicing to remove the first character from a string in Python doesn’t involve parameters in the same way as a method or function. Instead, it relies on the structure of the loop and the slicing notation.
original_string = "Hhello world"
new_string = ""
for i in range(1, len(original_string)):
new_string += original_string[i]
print(new_string)
In this code, we begin by defining an original_string
variable with the string value "Hhello world"
. We initialize an empty new_string
variable to construct the modified string that omits the initial character.
Next, a for
loop is set up, iterating through indices from 1
(the second character) to one less than the length of the original_string
. Inside the loop, each character (starting from index 1
) is appended to new_string
, constructing a modified string without 'H'
.
Finally, we display the content of new_string
using print(new_string)
.
Output:
hello world
In the output, the 'H'
at the beginning of the string is effectively removed. Our for
loop adeptly excludes the first character ('H'
) during its iteration through the original_string
.
This is achieved by consistently appending characters at each index to new_string
.
Conclusion
In the article, we’ve explored multiple strategies for removing the first character from a string in Python. These methods include slicing, the str.lstrip()
method, and regular expressions with the re.sub()
function.
Each method offers a distinct approach, enabling precise character removal while preserving the integrity of the original string. These techniques provide valuable tools for string manipulation in Python.