How to Generate Random Strings in Python
-
Generate Random Strings in Python Using the
random.choice()
andstring.join()
Methods -
Generate Random String in Python Using the
uuid.uuid4()
Method -
Generate Random String in Python Using the
StringGenerator.render_list()
Method
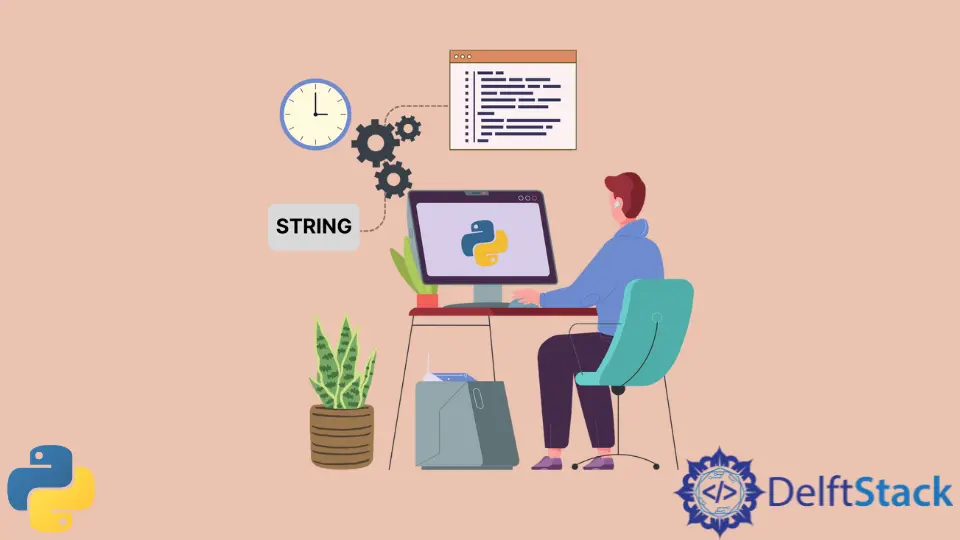
In this tutorial, we will look into various methods to generate random strings in Python. The random string generation techniques are used to generate random usernames, passwords or filenames, etc.
In some cases, we need cryptographically secure strings, i.e., random password or key generation. Just random strings will be enough if we need to use the random strings as random username and filename, etc. We will discuss both types of random string generation in this tutorial, which are explained below.
Generate Random Strings in Python Using the random.choice()
and string.join()
Methods
The random.choice(seq)
method returns a randomly chosen element from the sequence seq
provided as input. And the string.join(iterable)
method joins the elements of the iterable
by using the provided value of the string
as the separator and returns the resulting string as output.
To generate a random string in Python, we need to provide the sequence of characters from which we want our code to generate the random string to the random.choice()
method. The input sequence can consist of capital alphabets, small alphabets, digits and punctuation marks, etc.
We can use string.ascii_uppercase
and string.ascii_lowercase
for capital and small alphabets sequence respectively, string.ascii_letters
for both, string.digits
for digits sequence and string.punctuation
for punctuation marks sequence in Python.
The below example code demonstrates how to generate the required type of random string using the random.choice()
and string.join()
methods in Python.
import string
import random
number_of_strings = 5
length_of_string = 8
for x in range(number_of_strings):
print(
"".join(
random.choice(string.ascii_letters + string.digits)
for _ in range(length_of_string)
)
)
Output:
wOy5ezjl
j34JN8By
clA5SNZ6
D8K0eggH
6LjRuYsb
To generate the cryptographically secured random strings, we can use the random.SystemRandom()
method, which generates random numbers from the operating system’s sources.
Example code:
import string
import random
number_of_strings = 5
length_of_string = 8
for x in range(number_of_strings):
print(
"".join(
random.SystemRandom().choice(string.ascii_letters + string.digits)
for _ in range(length_of_string)
)
)
Output:
PEQBU72q
xuwUInGo
asVWVywB
SAsMRjka
CrbIpuR6
Generate Random String in Python Using the uuid.uuid4()
Method
The uuid.uuid4()
method generates and returns a random UUID. The UUID is a 128-bits long, universally unique identifier used to identify information in a system or network.
This method is useful in case we want to generate random and unique identifiers from the random strings. The below example code demonstrates how to use the uuid.uuid4()
method to get a random string in Python.
import uuid
print(uuid.uuid4())
Output:
440a93fe-45d7-4ccc-a6ee-baf10ce7388a
Generate Random String in Python Using the StringGenerator.render_list()
Method
The StringGenerator().render_list()
is an easy way to generate multiple random strings in Python. The StringGenerator()
takes the regular expression as input, which defines the characters that should be used to generate the random strings. In the renderlist(len, unique=)
method, len
specifies the length of the output list containing the random strings, and the unique
keyword argument can be set to True
if we want the unique output strings.
The StringGenerator
module needs to be installed first to use this method. The below example code demonstrates how to use the StringGenerator.render_list()
method to generate the random strings in Python.
from strgen import StringGenerator
StringGenerator("[\l\d]{10}").render_list(3, unique=True)
Output:
['m98xQHMlBI', 'V4O8hPMWfh', 'cBJk3XcGny']