How to Compress and Decompress Data Using Zlib in Python
-
Compress Data With the
zlib.compress()
Function in Python -
Decompress Data With the
zlib.decompress()
Function in Python
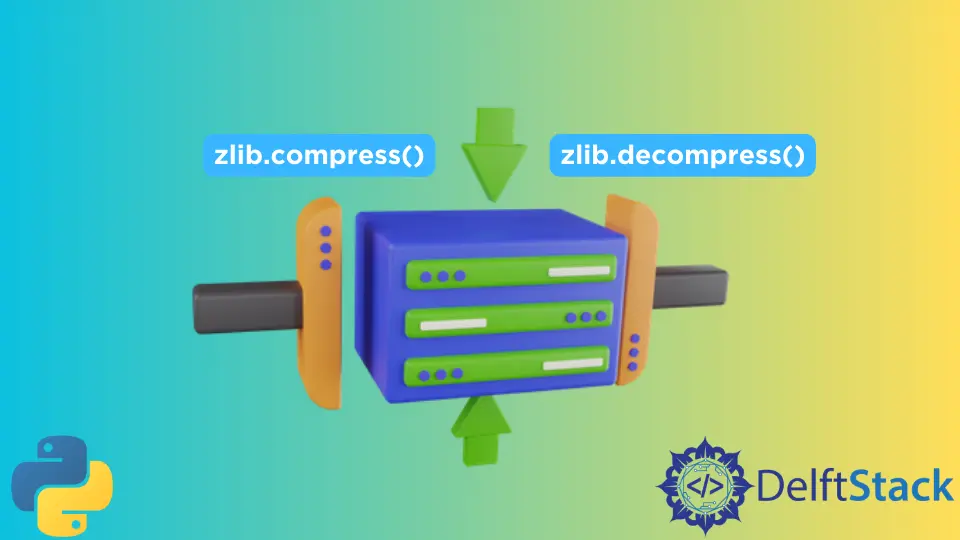
This tutorial will discuss how to compress and decompress byte strings with Python’s zlib
module.
Compress Data With the zlib.compress()
Function in Python
The compress(data, level=-1)
function inside the zlib
module compresses our byte data and returns a compressed byte object. It takes two parameters, data
and level
, where the data
parameter contains the data to be compressed, and the level
parameter specifies the compression level.
The level
parameter values range from 0
to 9
. The value 9
means the highest amount of compression, which is the slowest, and the value 1
means the lowest amount of compression, which is the fastest.
The default value of level
is -1
, which controls the compression to provide the best compromise between compression and speed.
The code snippet below demonstrates how to compress a string with Python’s zlib.compress()
function.
import zlib
s = b"This is a Byte String."
compressed = zlib.compress(s)
print("Compressed String:", compressed)
Output:
Compressed String: b'x\x9c\x0b\xc9\xc8,V\x00\xa2D\x05\xa7\xca\x92T\x85\xe0\x92\xa2\xcc\xbct=\x00VK\x07\x8f'
We compressed the string s
with the zlib.compress(s)
function. We converted our string into a Byte string before compression because the zlib.compress()
function only compresses the bytes of the data.
Decompress Data With the zlib.decompress()
Function in Python
We can use the decompress(data, wbits=MAX_WBITS, bufsize=DEF_BUF_SIZE)
function inside the zlib
module to decompress the bytes in previously compressed byte data and returns a decompressed byte object. It takes three parameters; data
, wbits
, and bufsize
.
The data
parameter contains the compressed data that needs to be decompressed.
The wbits
parameter contains the size of the history buffer while decompressing. Its default value is the maximum history buffer size available.
The wbits
parameter values are discussed in detail in the following table.
Value | Window Size | Input |
---|---|---|
+8 to +15 | base 2 | Must include zlib header and trailer |
-8 to -15 | Absolute value | Must not include zlib header and trailer |
+24 to +31 OR 16 + (+8 to +15) | Lowest 4 bits | Must include gzip header and trailer |
+40 to +47 OR 32 + (+8 to +15) | Lowest 4 bits | Either zlib or gzip format |
The bufsize
parameter specifies the initial buffer size, which automatically increases if more buffer size is required. We don’t always need to define the wbits
and bufsize
parameters while decompressing.
The following code snippet demonstrates how to decompress the previously compressed data with Python’s zlib.decompress()
function.
decompressed = zlib.decompress(compressed)
print("\nDecompressed String:", decompressed)
Output:
Decompressed String: b'This is a Byte String.'
We successfully decompressed the previously compressed string compressed
with the zlib.decompress(compressed)
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn