How to Unzip Files in Python
-
Unzip Files in Python Using the
extractall()
Method to the Working Directory -
Unzip Files in Python Using the
extractall()
Method to a Different Directory -
Unzip Files in Python Using the
extractall()
Method Based on the Condition -
Unzip Files in Python Using the
unpack_archive()
Method
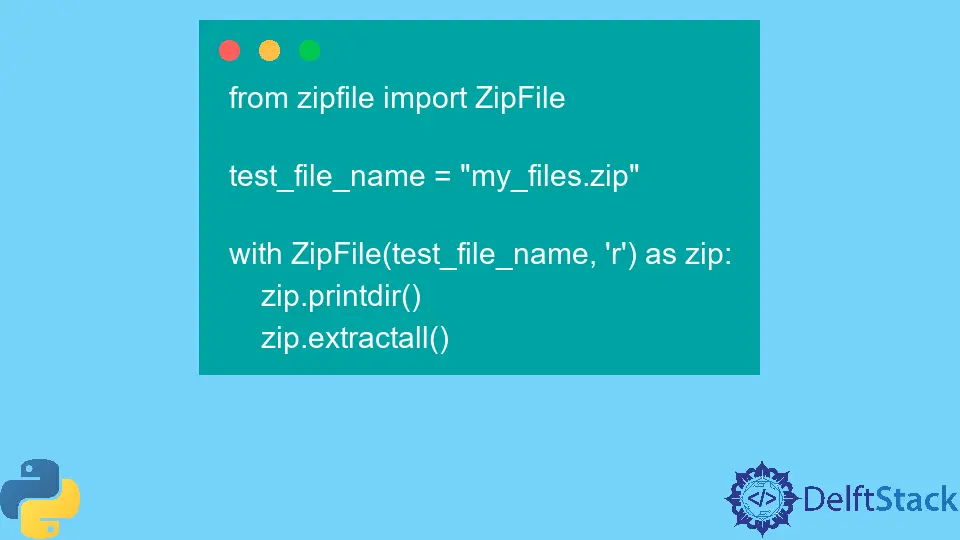
A ZIP
file has an archive file format and helps in lossless data compression. A single ZIP
file can contain more than one compressed file. In a ZIP
file, multiple files are transferred faster than a single file, and it decreases the size of the data. Python inbuilt ZipFile
module could be used to Unzip the Zip files.
This article will discuss the different methods to extracts the compressed or Zip files in Python.
Unzip Files in Python Using the extractall()
Method to the Working Directory
Let’s first import Python inbuilt zipfile
module using the below command.
from zipfile import ZipFile
The complete example code is as follows:
from zipfile import ZipFile
test_file_name = "my_files.zip"
with ZipFile(test_file_name, "r") as zip:
zip.printdir()
zip.extractall()
The r
in the ZipFile
function represents the reading file operation, whereas the extractall()
function will extract the contents of the zip file to the current working directory.
Output:
File Name Modified Size
file1.txt 2020-11-09 23:03:06 0
file2.txt 2020-11-09 23:03:18 0
Unzip Files in Python Using the extractall()
Method to a Different Directory
This method extracts the zip files but to a different directory. The main difference is that we will pass the destination directory as an argument in the extractall()
method.
The complete example code is as follows:
from zipfile import ZipFile
with ZipFile("my_files.zip", "r") as zip:
zip.extractall("temp")
print("File is unzipped in temp folder")
A new directory temp
will be created in the current working directory, and all the zip files are extracted there.
Output:
Files are unzipped in temp folder
Unzip Files in Python Using the extractall()
Method Based on the Condition
This method is handy when you have a large number of files in a zip file, and you need to extract files with a specific extension. For example, we will extract .txt
files from the zip file.
The complete example code is as follows:
from zipfile import ZipFile
with ZipFile("my_files.zip", "r") as obj_zip:
FileNames = obj_zip.namelist()
for fileName in FileNames:
if fileName.endswith(".txt"):
zipObj.extract(fileName, "temp_txt")
The namelist()
function returns the names of all the files in the zip file.
The endswith()
method checks whether the file name ends with .txt
. If so, the extract()
function will extract the .txt
files to the temp_txt
folder in the current working directory.
Unzip Files in Python Using the unpack_archive()
Method
This method uses Python inbuilt shutil
module. It will extract all formats of archived files.
The complete example code is as follows:
import shutil
shutil.unpack_archive("test.zip")
The extracted files will be in the current working directory where you are running this script.