How to Create Text Menu With Infinite Loop in Python
-
Create a Text Menu With Infinite Loop Using a
while
Loop in Python -
Terminate a Text Menu With Infinite Loop Using the
break
Statement in Python -
Terminate a Text Menu With Infinite Loop Using the
Flag
Variable in Python - Create a Text Menu With Infinite Loop Using a Dictionary of Functions in Python
- Conclusion
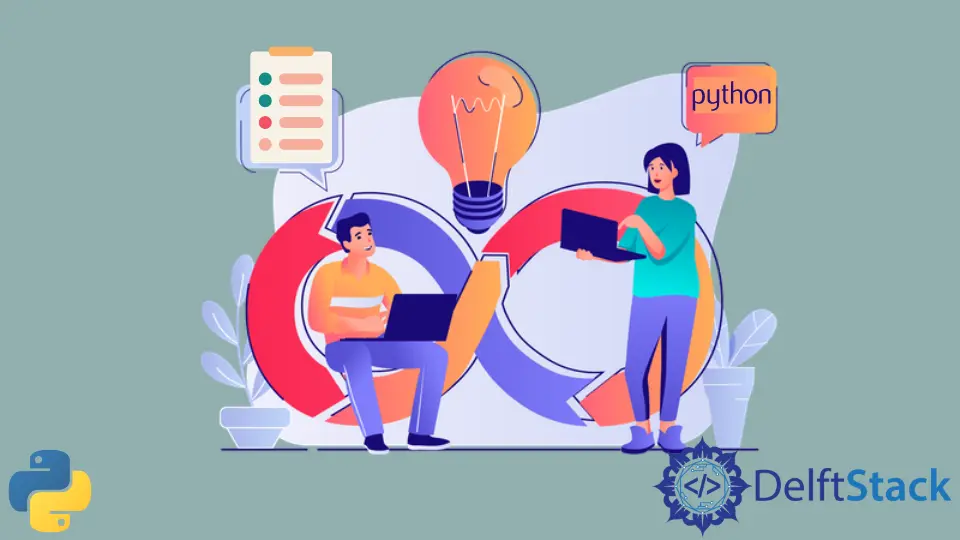
The combination of the while
loop and if-else
statements stands out as a fundamental building block for creating interactive and user-friendly programs.
In this article, we’ll explore how we can create a text menu with an infinite loop using the while
loop and if-else
statements. We will guide you through the process of crafting a text menu with an infinite loop, where users are presented with a set of options, input their choices, and see the corresponding actions executed by providing examples.
Create a Text Menu With Infinite Loop Using a while
Loop in Python
To create a text menu with an infinite loop, we’ll employ a while
loop combined with conditional statements. Inside the loop, we’ll present the user with several options, take their input, and then respond accordingly.
This process will continue until the user manually terminates the program. Let’s look into a practical example to understand this concept.
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
while True:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
Output:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
2
Hello
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
^D
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 11, in <module>
option = int(input())
EOFError: EOF when reading a line
In the Python code, we have a simple program that creates a text menu with an infinite loop. The options()
function displays a menu with five options, each associated with a number from 1
to 5
, and inside the infinite while
loop, the options()
function is called to show the menu to the user.
The program then waits for user input using the input()
function and stores the input as an integer in the option
variable. Based on the value of option
, the program prints a corresponding message, and if the user enters 1
, it prints "Hi"
, and if they enter 2
, it prints "Hello"
, and so on.
This process continues indefinitely until the user decides to manually terminate the program. When the user presses Ctrl+D
(EOF), it raises an error, indicating the end of input, and the program terminates.
As discussed in the following sections, we can use several ways to terminate the while
loop to advance into the program.
Terminate a Text Menu With Infinite Loop Using the break
Statement in Python
We can instruct the user to enter any number outside the specified options to exit the while
loop. We include an else
block in the conditional statements to check for this condition.
If the user enters any number not in the range of 1
to 5
, we print "Bye"
and use the break
statement to exit the loop.
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
print("Enter any other number to terminate.")
while True:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
else:
print("Bye")
break
Output:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
3
Namaste
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
12
Bye
In this Python code, we’ve created a text menu with an infinite loop. The options()
function displays a menu to the user, listing five choices from 1
to 5
, and provides instructions for termination by entering any other number and within a while
loop that runs indefinitely, we call the options()
function to display the menu.
We then await user input, which we convert to an integer using int(input())
. Based on the value of the option
variable, we print corresponding messages, and if the user enters 1
, we display "Hi"
; for 2
, it’s "Hello"
, and so on.
If the user enters any other number, we print "Bye"
and use the break
statement to exit the loop, terminating the program. The code demonstrates a user-friendly menu-driven interface that operates until the user decides to end it.
Terminate a Text Menu With Infinite Loop Using the Flag
Variable in Python
Instead of relying on the break
statement, we can use a flag
variable to control the loop’s execution. Initialize the flag
variable as True
before entering the while
loop.
Inside the loop, if the user enters any number outside the specified options, we print "Bye"
and set the flag
variable to False
. Once the flag
variable becomes False
, the while
loop automatically terminates.
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
print("Enter any other number to terminate.")
flag = True
while flag:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
else:
print("Bye")
flag = False
Output:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
3
Namaste
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
12
Bye
In this Python code, we’ve created a text menu with an infinite loop controlled by a flag
variable. The options()
function displays a menu with five choices from 1
to 5
, providing clear instructions for termination by entering any other number, and we initialize the flag
variable as True
to enter the loop.
Inside the loop, we call the options()
function to display the menu to the user, and then we await their input, converting it to an integer with int(input())
. Depending on the value of the option
variable, we print the corresponding message, such as "Hi"
for 1
, "Hello"
for 2
, and so on.
If the user enters any other number, we print "Bye"
and set the flag
variable to False
, thereby exiting the loop and concluding the program. This code presents a straightforward, menu-driven interface that continues until the user chooses to exit.
Create a Text Menu With Infinite Loop Using a Dictionary of Functions in Python
A more structured approach is to create a dictionary of functions, associating each option with a specific function. This simplifies the code and makes it more extensible.
Creating a text menu with an infinite loop using a dictionary of functions in Python involves defining a menu where each option is associated with a specific function. Instead of using a series of if-elif
statements, you create a dictionary where the keys represent the menu options, and the values are functions to be executed when the corresponding option is selected.
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
print("Enter any other number to terminate.")
def print_hi():
print("Hi")
def print_hello():
print("Hello")
def print_namaste():
print("Namaste")
def print_bonjour():
print("Bonjour")
def print_hola():
print("Hola")
def exit_menu():
print("Bye")
exit()
menu = {
1: print_hi,
2: print_hello,
3: print_namaste,
4: print_bonjour,
5: print_hola,
6: exit_menu,
}
while True:
print("Enter your choice:")
options()
option = int(input())
if option in menu:
menu[option]()
else:
print("Invalid choice. Please try again.")
Output:
Enter your choice:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
1
Hi
Enter your choice:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
4
Bonjour
Enter your choice:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
9
Invalid choice. Please try again.
In this Python code, we have a set of functions: print_hi()
, print_hello()
, print_namaste()
, print_bonjour()
, print_hola()
, and exit_menu()
. Each of these functions is associated with a specific menu option and is responsible for displaying a corresponding message or performing an action.
We also have an options()
function that presents the available menu options to the user, including instructions on how to terminate the program by entering any other number. Next, we define a dictionary named menu
, where each key corresponds to a menu option (1 to 6
), and the values are references to the corresponding functions.
Within an infinite while
loop, we continuously display the menu and prompt the user to enter their choice.
After the user’s input, we check if the entered option is in the menu
dictionary. If it is, we execute the associated function by calling menu[option]()
, and if the option is not found in the dictionary, we display "Invalid choice. Please try again"
.
The program keeps running until the user manually decides to exit by selecting option 6
, which calls the exit_menu()
function and gracefully terminates the program. This code creates an interactive menu-driven interface, allowing users to choose different actions or exit at their discretion.
Conclusion
We’ve discussed various methods for creating a text menu with an infinite loop in Python. This simple approach combines the while
loop with conditional statements to present users with a menu of options, take their input, and execute corresponding actions.
We’ve also explored different ways to exit the infinite loop using the break
statement and a flag
variable, ensuring that users have the flexibility to continue interacting with the program or terminate it at their convenience. Whether you’re building command-line tools, interactive applications, or simple menus, this technique provides a user-friendly and interactive way to control the flow of your Python programs.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub