How to Get a Subarray of an Array in Python
- Use Slicing to Get a Subarray of an Array in Python
-
Use the
numpy
Library to Get Python Subarray - Use List Comprehension to Get a Subarray of an Array in Python
-
Use the
filter
Function to Get a Subarray of an Array in Python -
Use NumPy’s
arange
Function to Get a Subarray of an Array in Python
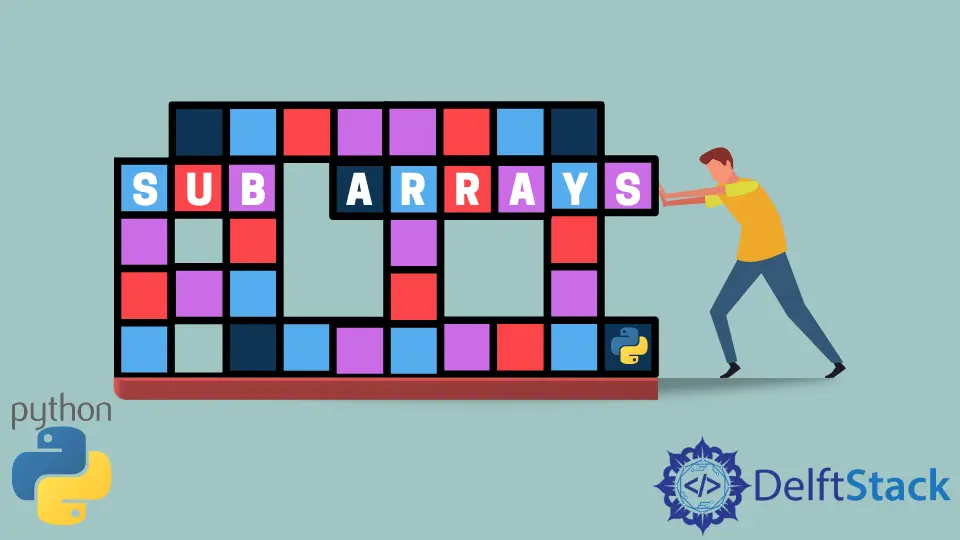
A contiguous part of an array is a subarray, and a contiguous part of a string is a substring. The order of elements remains the same relative to the original list or string for both of them.
Working with arrays is an essential aspect of programming, and at times, you may have to extract a subset of elements from an array or [add a subarray to an existing array]({{relref “/HowTo/Python/append 2d array python.en.md”}}). In Python, this process is commonly referred to as obtaining a subarray.
Python makes obtaining a subarray or a substring very straightforward compared to most of the other programming languages. In this article, we will learn how to obtain a subarray of an array in Python.
Use Slicing to Get a Subarray of an Array in Python
Slicing is a powerful and concise way to obtain subarrays in Python. It involves specifying the start and end indices within square brackets. For a more in-depth guide on slicing techniques, visit [Reverse Order Using Slicing in Python]({{relref ‘/HowTo/Python/reverse order using slicing in python.en.md’}}).
In Python, we can get a subarray of an array using slicing. Extending indexing, which is an easy and convenient notation, can be used to slice an array or a string.
It has the following syntax:
object[start:end:step]
The following is the explanation of each component:
object
: A list or a string.start
: The starting index for slicing. The default value is0
.end
: The ending index for slicing. Note that the value of this index is not a part of the result. The default value is the iterable object’s length.step
: The number of increments between each index in the result. By default, it is1
.
Let us understand how to use this Python feature to get a subarray of an array with the help of some examples.
Example 1:
array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
a = array[1:4]
b = array[0:8]
c = array[6:]
d = array[:5]
print(a)
print(b)
print(c)
print(d)
Output:
As we can see, the output contains all the indexes between the start
and the end
index (start
inclusive and end
exclusive). Moreover, when no value is set for the start
, the first index is considered by default, and when no value is set for the end
, the last value is considered by default.
We can extend this even further and apply slicing to each value of a list. We will make a list of strings and a list of lists and find a substring and a subarray for each string and list using list comprehension and slicing.
List comprehension is an inline syntax for iterating over a list or lists and creating a new list.
Example 2:
a = ["Hello", "World", "Computer", "Science", "GitHub", "StakeOverflow"]
b = [
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
[1.1, 2.2, 3.3, 4.4, 5.5],
[0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9],
["Q", "W", "E", "R", "T", "Y"],
[True, False, None, None, False, True],
]
x = [element[1:5] for element in a]
y = [element[1:3] for element in b]
print(x)
print(y)
Output:
For each string in the list of strings, the above code concatenates characters present at indexes 1
, 2
, 3
, and 4
into a new string and creates a new list of strings.
For the list of lists, it clubs together all the values at indexes 1
and 2
and creates a new list of lists. Furthermore, we store both the new lists in two new variables and print them.
Use the numpy
Library to Get Python Subarray
If you are working with multidimensional arrays, the numpy
library provides a robust solution. The numpy
array allows for more advanced indexing, including the use of arrays as indices.
Example:
import numpy as np
original_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
subarray = original_array[1:, :2]
print(subarray)
Output:
The original_array[1:, :2]
selects rows starting from index 1 and columns up to index 2 (exclusive). The resulting subarray
will be array([[4, 5], [7, 8]])
.
Use List Comprehension to Get a Subarray of an Array in Python
List comprehension provides a straightforward way to create lists, making it an efficient method to obtain subarrays based on a condition. Learn more about list comprehensions in our article on [Nested List Comprehension in Python]({{relref ‘/HowTo/Python/nested list comprehension python.en.md’}}).
Example:
original_array = [1, 2, 3, 4, 5]
subarray = [x for x in original_array if x % 2 == 0]
print(subarray)
Output:
The [x for x in original_array if x % 2 == 0]
creates a subarray containing only even numbers. The resulting subarray
will be [2, 4]
.
Use the filter
Function to Get a Subarray of an Array in Python
The filter
function can be employed to create a subarray based on a specified condition. It takes a function and an iterable as arguments. To explore more ways of usingfilter()
effectively, check out [Filter Lambda Python]({{relref ‘/HowTo/Python/filter lambda python.en.md’}}).
Example:
original_array = [1, 2, 3, 4, 5]
subarray = list(filter(lambda x: x % 2 == 0, original_array))
print(subarray)
Output:
The filter(lambda x: x % 2 == 0, original_array)
creates a subarray containing only even numbers. The resulting subarray
will be [2, 4]
.
Use NumPy’s arange
Function to Get a Subarray of an Array in Python
NumPy provides the arange
function to generate sequences of numbers, which can be used to index elements and obtain subarrays. To dive deeper into NumPy array operations, check out [NumPy Array to List]({{relref ‘/HowTo/Python/numpy array to list.en.md’}})
Example:
import numpy as np
original_array = np.array([1, 2, 3, 4, 5])
indices = np.arange(1, 4)
subarray = original_array[indices]
print(subarray)
Output:
The np.arange(1, 4)
generates an array [1, 2, 3]
, which is then used to index original_array
. The resulting subarray
will be [2, 3, 4]
.