How to Convert String to Double in Python
-
Convert a String to a Double in Python Using the
float()
Function -
Convert a String to a Double in Python Using the
decimal.Decimal()
Method -
Convert a String to a Double in Python Using the
ast.literal_eval()
Method -
Convert a String to a Double in Python Using the
np.fromstring()
Method - Conclusion
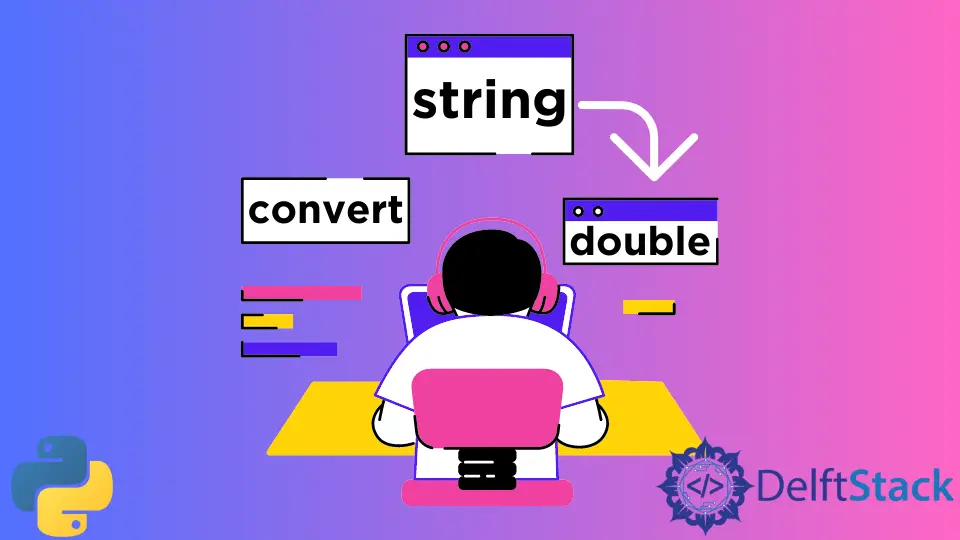
Converting a string to a double in Python is a fundamental operation frequently encountered in various programming tasks. This article will provide a guide to the methods available for converting string data into double-precision floating-point numbers in Python.
Whether you are dealing with numerical input or need to parse and process textual data, this resource will provide you with the information and insights you need to manage this typical activity efficiently.
Convert a String to a Double in Python Using the float()
Function
The float()
function accepts a number or a string as input and returns the floating-point number or a float type as output. To convert a string into a float, we can pass the string to the float()
function.
Syntax:
float(string)
Parameter:
string
(required): This is the string or numeric value that you want to convert to a double (floating-point number).
In the syntax, we provide the string
parameter, and the float()
function returns a floating-point number corresponding to the value contained in the string.
The code example below demonstrates how to use the float()
method to convert a string to a double in Python.
string = '1974.6323'
myfloat = float(string)
print(myfloat)
In this code snippet, we start by initializing a variable named string
with the value 1974.6323
. This value is a string representing a numeric decimal (double) value.
Next, we utilize the float()
function to convert the string
variable, which contains a string representation of a numeric value, into a floating-point (double) number.
The resulting double value is stored in the variable myfloat
. Finally, we use the print()
function to display the converted double value stored in myfloat
.
Output:
1974.6323
The output above is the converted double value. The code took the string 1974.6323
and turned it into a floating-point number (double), preserving its precision.
Convert a String to a Double in Python Using the decimal.Decimal()
Method
The decimal.Decimal()
constructor method of the decimal
module takes a numeric value as input and converts it into a decimal type, which can exactly store and represent all the numeric values and can have as much precision as needed.
Syntax:
from decimal import Decimal
Decimal(string)
Parameter:
string
(required): This is the string or numeric value that you want to convert to a decimal.
In the syntax, we provide the string
parameter, and the Decimal()
method returns a Decimal
object representing the numeric value contained in the string. This method is particularly useful when you need high precision for decimal calculations.
The code example below demonstrates how to use the Decimal()
method to convert a string to a double in Python.
from decimal import Decimal
string = '1974.6323'
myfloat = Decimal(string)
print(myfloat)
In this code snippet, we start by importing the Decimal
class from the decimal
module, which specializes in high-precision decimal floating-point operations, surpassing the capabilities of the standard float type. Next, we initialize a variable named string
with the value 1974.6323
, representing a numeric decimal in string format.
We proceed to use the Decimal()
constructor to convert the string
variable, containing a string representation of a number, into a high-precision decimal number. The result is stored in the variable myfloat
.
Finally, we use the print()
function to display the converted double stored in myfloat
.
Output:
1974.6323
The output above is the converted string to double using the Decimal()
method.
Convert a String to a Double in Python Using the ast.literal_eval()
Method
The ast.literal_eval()
is a function provided by the Python Standard Library’s ast
module. It is typically used for evaluating a string containing a literal Python expression, and it returns the corresponding Python object.
Syntax:
import ast
ast.literal_eval(string)
Parameter:
string
(required): This is the string containing a valid literal Python expression (e.g., a number) that you want to evaluate and convert to a Python object.
In the syntax, we provide the string
parameter, and the ast.literal_eval()
method returns the corresponding Python object based on the expression within the string. It’s a safer alternative to the built-in eval()
function because it only evaluates literal expressions and does not execute arbitrary code.
The code example below demonstrates how to use the ast.literal_eval()
method to convert a string to a double in Python.
import ast
string = '1974.6323'
myfloat = ast.literal_eval(string)
print(myfloat)
In this code snippet, we begin by importing the ast
module, a part of the Python Standard Library that offers tools for manipulating abstract syntax trees. The module includes a valuable function, literal_eval()
, which allows for the safe evaluation of literal expressions within strings.
Then, we initialize a variable called string
and assign it the value 1974.6323
, which represents a numeric decimal value. Our objective is to convert this string into a double-precision floating-point number.
To achieve this, we utilize the ast.literal_eval()
method, passing the string
as its argument. This method then evaluates the string as a literal Python expression, in this case, interpreting it as a numeric value and returning it as a double.
Lastly, we utilize the print()
function to display the result of the conversion, which is stored in the myfloat
variable.
Output:
1974.6323
The output of the code is the conversion of the string 1974.6323
into a high-precision double.
We use the ast.literal_eval()
to safely evaluate the string 1974.6323
as a numeric value. The resulting output is the double value 1974.6323
, which accurately represents the original numeric value contained in the string.
Convert a String to a Double in Python Using the np.fromstring()
Method
The np.fromstring()
method is not typically used to convert a single number in a string like the Decimal()
constructor. The np.fromstring()
is usually used for converting a string that represents an array of numbers.
We can still use the np.fromstring()
method from the NumPy
library to convert a string to a double in Python. However, you need to make sure that the string is properly formatted to represent a sequence of numbers.
This method is useful when dealing with strings that represent numbers.
Syntax:
numpy.fromstring(string, dtype=float, count=-1, sep='')
Parameters:
string
(required): This is the input string you want to convert to aNumPy
array. It can be a binary or text string.dtype
(optional): This parameter specifies the data type of the resultingNumPy
array elements. In this case, we specifydtype=float
to convert the string into aNumPy
array of floating-point numbers.count
(optional): This parameter specifies the number of elements to read from the input string. If not specified, or if set to-1
, it reads all available elements.sep
(optional): This parameter specifies the character used to separate the elements within the input string. The default separator is an empty string (''
).
In the syntax, We use numpy.fromstring()
to convert input strings into NumPy
arrays, with options for data type, element count, and separator. The primary parameters include string
(the input string), dtype
(specifying the array’s data type), count
(for element count), and sep
(used to separate elements).
The default separator is an empty string, and -1
for count
reads all available elements. This function proves versatile for handling various data formats.
The code example below demonstrates how to use the np.fromstring()
method to convert a string to a double in Python.
import numpy as np
string = '1974.6323'
double_array = np.fromstring(string, dtype=float, sep=' ')
myfloat = double_array[0] if len(double_array) > 0 else None
if myfloat is not None:
print(f"Converted Double: {myfloat}")
else:
print("Could not convert the string to a double.")
In this code snippet, we first import the NumPy
library as np
. Next, we initialize the string
variable with the value 1974.6323
, representing the numeric value to convert it into a double.
Then, we use the np.fromstring()
method to perform the conversion, specifying the data type as float
and the separator as a space (' '
). The result is stored in double_array
.
To ensure accuracy, we check if double_array
is not empty. If successful, myfloat
captures the converted double; otherwise, it’s set to None
.
Conditional statements are employed to display the converted double or a message if the conversion fails.
The output confirms the conversion’s success, displaying Converted Double: 1974.6323
. This method is handy for handling strings representing numerical values and includes error handling for possible conversion failures.
Output:
Converted Double: 1974.6323
The output displays the successful conversion of the string 1974.6323
into a double using the np.fromstring()
method. The code correctly parses the string as a single number.
Please keep in mind that this approach is intended to work with strings that represent sequences of numbers, and the string should be properly formatted. If the string format doesn’t match, you may encounter errors or incorrect results.
Conclusion
In this article, we discussed several Python methods for converting a string to a double. We began with the float()
function, which is a straightforward and widely used approach for this conversion.
We then delved into the decimal.Decimal()
method, which offers high precision and is useful for scenarios where accuracy is paramount. Furthermore, we discussed the ast.literal_eval()
method, a safe alternative to the eval()
function for evaluating literal Python expressions within a string.
Finally, we explored the np.fromstring()
method from the NumPy
library, which is primarily designed for converting arrays of numbers within properly formatted strings. With these strategies, you now have the knowledge and tools to do string-to-double conversions in Python efficiently.