How to Split Strings by Tab in Python
- Use Regex to Divide Given String by Tab in Python
-
Split Strings by Tab in Python Using the
str.split()
Method - Conclusion
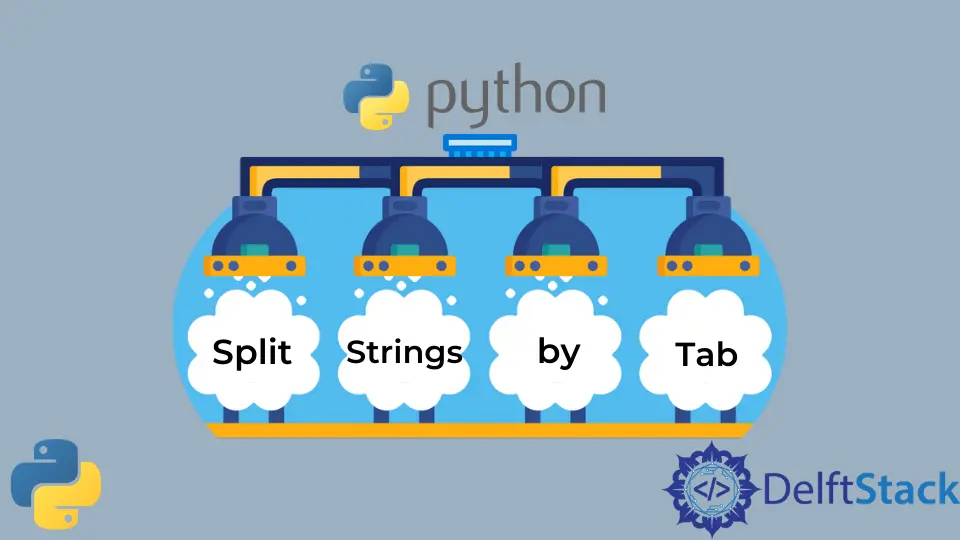
Understanding how to split strings effectively in Python is essential for data manipulation and text processing.
This tutorial focuses on various techniques for splitting strings, specifically by tab.
Use Regex to Divide Given String by Tab in Python
Regular expressions, often referred to as regex or regexp, are a powerful tool for pattern matching and text manipulation. They provide a concise and flexible way to define patterns within strings.
When dealing with structured data like tab-separated values, regular expressions can help you precisely locate and extract the desired information.
Using the re.split()
Function
The re
module in Python provides a split()
function that can be used to split strings using regular expressions. To split a string by tabs, you can define the tab character \t
as the delimiter.
import re
text = "abc\tdef\tghi"
parts = re.split(r"\t", text)
print(parts)
Output:
['abc', 'def', 'ghi']
In this example, we use re.split(r'\t', text)
to split the string text
using the regular expression \t
, which matches the tab character. As seen in the output, the program successfully divided the given string by the tab character.
Using Regex flags
Regular expressions can become more powerful when you utilize flags
to control the behavior of the regex pattern. For instance, you can use the re.MULTILINE
flag to split a multiline string by tabs.
import re
text = "Line1\tTab1\tTab2\nLine2\tTab1\tTab2"
parts = re.split(r"\t", text, flags=re.MULTILINE)
print(parts)
Output:
['Line1', 'Tab1', 'Tab2\nLine2', 'Tab1', 'Tab2']
The code example above shows how the regex flags
splits the string into individual components, considering each line separately. As seen in the output, the program separated the given string by the tab character.
Splitting by Multiple Tabs
If your data contains multiple consecutive tabs and you want to treat them as a single delimiter, you can use the +
quantifier to match one or more tabs.
import re
text = "abc\t\tdef\tghi"
parts = re.split(r"\t+", text)
print(parts)
Output:
['abc', 'def', 'ghi']
In this example, r'\t+'
matches one or more consecutive tabs as a single delimiter. Thus, the program outputs ['abc', 'def', 'ghi']
from the given string: "abc\t\tdef\tghi"
.
Splitting by Whitespace (Tabs and Spaces)
Sometimes, your data may contain both tabs and spaces as delimiters. To handle this, you can use the \s
pattern to match any whitespace character (including tabs and spaces).
import re
text = "abc\t def ghi"
parts = re.split(r"\s+", text)
print(parts)
Output:
['abc', 'def', 'ghi']
In this code example, the parts = re.split(r"\s+", text)
line uses the re.split()
function to split the string text into a list of substrings based on the regular expression r"\s+"
. This operation also breaks the string into parts whenever it encounters one or more whitespace characters.
Thus, the final output of the code is a list containing three elements: 'abc'
, 'def'
, and 'ghi'
, which are the parts of the original string separated by whitespace characters.
Using the str.rstrip()
Function and Regex
In case you have a string with a trailing tab. Our objective is to split the string based on tab characters, making sure that any trailing tab is eliminated.
This approach helps us avoid having an empty string element at the end of the resulting list, which can occur when trailing characters are not removed.
To achieve this, we utilize the str.rstrip()
function, which efficiently removes trailing characters from a string. In this scenario, we apply a regular expression to identify and remove any trailing tab characters.
import re
text = "abc\tdef\tghi\t"
trimmed_text = text.rstrip("\t")
split_text = re.split(r"\t", trimmed_text)
print(split_text)
In this code snippet, text
represents the original string abc\tdef\tghi\t
with a trailing tab character. The rstrip('\t')
function removes the trailing tab, resulting in trimmed_text = "abc\tdef\tghi"
.
After trimming the string, we use re.split()
to split the trimmed text by tab characters using the regular expression r'\t'
. The outcome is a list with elements that were separated by tabs.
Output:
['abc', 'def', 'ghi']
As seen in the output, the trailing tab character was removed, and the original string got split by the tab character, resulting in a list containing three elements: 'abc'
, 'def'
, and 'ghi'
.
Split Strings by Tab in Python Using the str.split()
Method
When it comes to tab-separated data, Python’s str.split()
method is also a versatile and straightforward way to achieve this. We’ll cover various techniques, including using different parameters to enhance your string-splitting capabilities.
Using the str.split()
Method With
as the Separator
The str.split()
method allows you to split a string into a list of substrings by using a specified delimiter. To split a string by tab characters (\t
), you can pass \t
as the separator.
text = "This\tis\tan\texample\tstring"
parts = text.split("\t")
print(parts)
In this example, text.split('\t')
will split the text
string into a list, using tab characters as the delimiter. The resulting list, parts
, will contain each component separated by tabs.
Output:
['This', 'is', 'an', 'example', 'string']
The final output of the code is a list containing five elements: 'This'
, 'is'
, 'an'
, 'example'
, and 'string'
. These elements are the parts of the original string separated by the tab characters.
Using the str.split()
Method With the sep
Parameter (Python 3.9 and Later)
Starting from Python 3.9, the str.split()
method introduced the sep
parameter, which allows you to specify the separator directly.
text = "This\tis\tan\texample\tstring"
parts = text.split(sep="\t")
print(parts)
Output:
['This', 'is', 'an', 'example', 'string']
In this version of the str.split()
method, you can pass \t
as the sep
parameter to achieve the same result as in the previous method. This offers a more explicit and Pythonic way to specify the separator.
Using the str.split()
Method With the maxsplit
Parameter
The str.split()
method also allows you to split a string a certain number of times by using the maxsplit
parameter.
text = "This\tis\tan\texample\tstring"
parts = text.split("\t", 4)
print(parts)
Output:
['This', 'is', 'an', 'example', 'string']
The split("\t", 4)
operation breaks the string into parts whenever it encounters a tab character, but it stops after the fourth occurrence of the tab. The resulting list will have at most 5
elements, as it includes the segments before and after the first four tabs.
The final output of the code is a list containing five elements: 'This'
, 'is'
, 'an'
, 'example'
, and 'string'
. These elements are the parts of the original string separated by the tab characters, but the splitting stops after the fourth tab.
This behavior is controlled by the second argument (4
) in the split()
method.
Conclusion
In data analysis and text processing, accurate string splitting is essential. This article has explored various methods in Python for splitting strings using tabs, making it simpler to handle tab-separated data, multiline content, and trailing characters.
Whether you prefer the flexibility of regular expressions or the simplicity of Python’s str.split()
method, you now have the tools to navigate string manipulation intricacies in Python. With this knowledge, you can confidently approach data parsing tasks, enhancing the versatility and power of your Python code.