How to Get the String Length and Size in Python
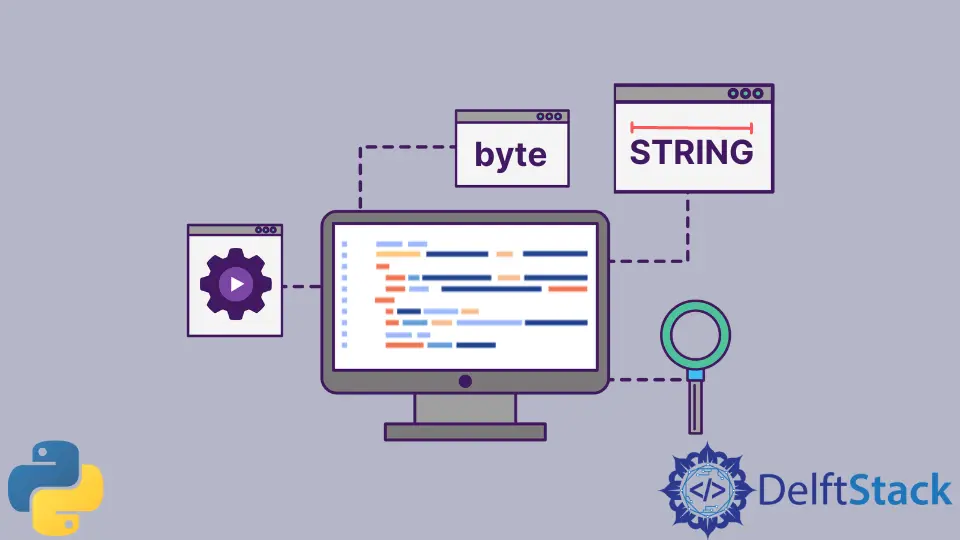
Understanding how to measure the length of a string is fundamental in Python programming. Whether you’re manipulating text, validating user input, or working with data, knowing the size of your strings is essential.
In this tutorial, we will explore various methods to determine the length of a string in Python. From the built-in functions to unique approaches, we’ll ensure you have a solid grasp of how to get string lengths efficiently. So, if you’re ready to dive into the world of Python string manipulation, let’s get started!
Using the Built-in len()
Function
The simplest and most common way to find the length of a string in Python is by using the built-in len()
function. This function takes a string as an argument and returns the number of characters in it. It counts all characters, including letters, numbers, spaces, and punctuation.
Here’s how you can use it:
my_string = "Hello, World!"
length_of_string = len(my_string)
print(length_of_string)
Output:
13
In this example, we first define a string called my_string
with the value “Hello, World!”. We then pass this string to the len()
function, which calculates its length. The result is stored in the variable length_of_string
, which is then printed. The output shows that the string contains 13 characters in total.
Using len()
is straightforward and efficient, making it the go-to method for measuring string lengths in Python. This function works with any string, regardless of its content, and is widely used in various applications, from data processing to web development.
Using String Methods
Apart from the len()
function, Python strings come with a variety of methods that can be useful for string manipulation. While these methods don’t directly return the length, they can help you manipulate the string before measuring its length.
For instance, you might want to count the length of a string after stripping whitespace or converting it to lowercase. Here’s how you can do that:
my_string = " Hello, World! "
cleaned_string = my_string.strip()
length_of_cleaned_string = len(cleaned_string)
print(length_of_cleaned_string)
Output:
13
In this example, we start with a string that has leading and trailing spaces. By using the strip()
method, we remove those spaces. We then apply the len()
function to the cleaned string. The final output shows that the length remains 13, but now we have a string without unnecessary whitespace.
Using string methods like strip()
or lower()
before measuring length can be particularly useful when validating user input or preparing data for analysis. It ensures that you’re counting only the characters you care about, providing a more accurate measurement.
Using List Comprehensions
Another interesting approach to get the length of a string is by using list comprehensions. This method might seem a bit unconventional compared to the straightforward len()
function, but it can be useful in specific scenarios, especially when you want to perform additional operations while counting.
Here’s an example of how to use a list comprehension to count the length of a string:
my_string = "Hello, World!"
length_of_string = sum(1 for _ in my_string)
print(length_of_string)
Output:
13
In this code, we utilize a generator expression within the sum()
function. The expression 1 for _ in my_string
iterates over each character in my_string
, yielding 1
for each character. The sum()
function then adds these values together to give the total count.
While this method is more complex than simply using len()
, it demonstrates the flexibility of Python and can be adapted for more advanced string processing tasks, such as filtering characters based on certain conditions while counting.
Conclusion
In summary, measuring the length of a string in Python is a fundamental skill that every programmer should master. Whether you choose the straightforward len()
function, utilize string methods for data cleaning, or explore list comprehensions for more complex scenarios, you now have multiple tools at your disposal. Understanding these methods will enhance your ability to manipulate and validate strings effectively, paving the way for more advanced Python programming. Happy coding!
FAQ
-
How does the
len()
function work in Python?
Thelen()
function returns the number of characters in a string, including spaces and punctuation. -
Can I use
len()
on other data types in Python?
Yes, thelen()
function can be used on other data types like lists and tuples, returning the number of elements in them.
-
What happens if I pass an empty string to
len()
?
If you pass an empty string tolen()
, it will return 0, indicating there are no characters. -
Are there any performance differences between using
len()
and other methods?
Yes,len()
is the most efficient method for measuring string length, while other methods may involve additional processing. -
Can I use
len()
to count specific characters in a string?
No,len()
counts all characters. To count specific characters, you would need to use a loop or list comprehension.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn