How to Restart a Loop in Python
-
Using the
continue
Statement -
Using a
while
Loop with a Condition - Using a Function to Encapsulate the Loop
- Conclusion
- FAQ
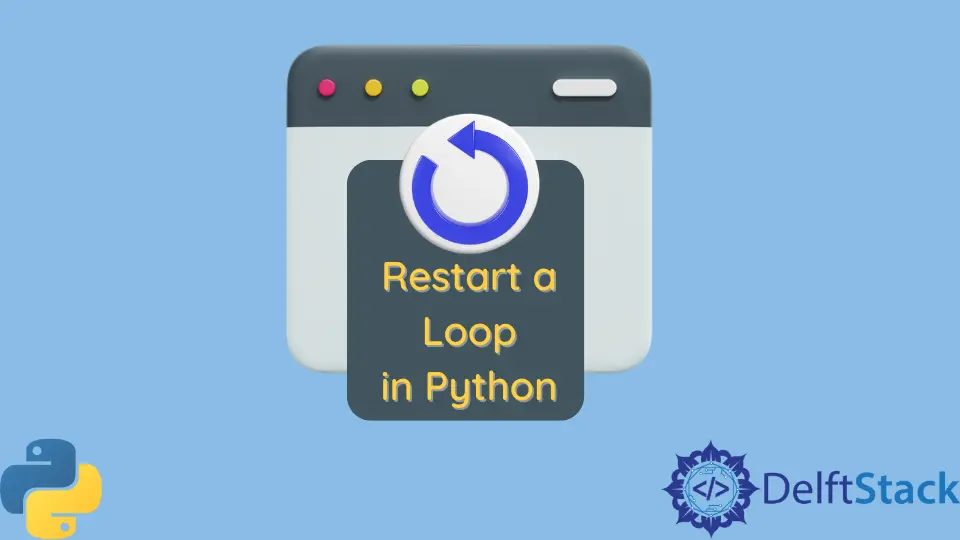
When programming in Python, managing loops effectively is crucial for creating efficient and functional code. Sometimes, you may need to restart a loop based on certain conditions, allowing your program to behave dynamically.
This article will guide you through various methods to restart a loop in Python, ensuring you have the tools to control your program flow effectively. We’ll explore practical examples and clear explanations, making it easy for you to implement these techniques in your own projects. Whether you are a beginner or looking to refine your skills, understanding how to restart a loop can enhance your coding repertoire.
Using the continue
Statement
One of the simplest ways to restart a loop in Python is by using the continue
statement. When Python encounters continue
, it skips the remaining code inside the loop for the current iteration and jumps back to the beginning of the loop. This is especially useful when you want to skip certain conditions and continue processing the rest of the loop.
Here’s a straightforward example:
for i in range(5):
if i == 2:
continue
print(i)
Output:
0
1
3
4
In this example, the loop iterates over the numbers 0 to 4. When i
equals 2, the continue
statement is triggered, skipping the print function for that iteration. As a result, the output omits the number 2, demonstrating how continue
effectively restarts the loop without executing the remaining code for that iteration.
Using continue
is particularly useful in scenarios where you want to avoid executing certain parts of your loop based on specific conditions. This method keeps your code clean and enhances performance by preventing unnecessary computations.
Using a while
Loop with a Condition
Another approach to restarting a loop in Python is to utilize a while
loop with a condition that can be modified during the loop’s execution. This method provides more control over when to restart the loop, allowing for complex logic and conditions.
Here’s how this can be implemented:
i = 0
while i < 5:
if i == 2:
i += 1
continue
print(i)
i += 1
Output:
0
1
3
4
In this example, we initialize i
to 0 and use a while
loop to iterate until i
is less than 5. When i
equals 2, we increment i
and use continue
to skip the print statement. After each print, we also increment i
. The result is the same as the previous example, showing how a while
loop can also effectively restart its execution based on specific conditions.
Using a while
loop gives you the flexibility to control the loop’s behavior more intricately. You can add more complex conditions and logic, making it suitable for scenarios where the loop’s exit criteria may change dynamically.
Using a Function to Encapsulate the Loop
Encapsulating your loop within a function is another effective way to manage loop restarts. By defining a function, you can call it multiple times, allowing you to restart the loop as needed. This method is particularly useful when the loop logic is complex and you want to avoid code duplication.
Here’s an example of how to implement this:
def restartable_loop():
for i in range(5):
if i == 2:
print("Restarting the loop...")
return restartable_loop()
print(i)
restartable_loop()
Output:
0
1
Restarting the loop...
0
1
3
4
In this code, we define a function called restartable_loop
. Inside the function, we iterate over a range of numbers. When i
equals 2, we print a message and call the function again, effectively restarting the loop. The output shows how the loop restarts and continues processing after the interruption.
This method is particularly useful for more complex logic where you need to restart the loop multiple times based on various conditions. By encapsulating the loop in a function, you enhance readability and maintainability, making your code easier to manage and understand.
Conclusion
Restarting a loop in Python is a valuable skill that can streamline your coding process and enhance your program’s functionality. By using techniques such as the continue
statement, while
loops, or encapsulating loops in functions, you can effectively manage the flow of your code. Each method has its advantages, and understanding when to use each can significantly improve your programming efficiency. Whether you are a novice or an experienced developer, mastering these techniques will empower you to write cleaner and more dynamic Python code.
FAQ
-
How do I restart a loop without using a function?
You can use thecontinue
statement to skip the current iteration and continue with the next one. -
Can I restart a loop conditionally?
Yes, by using awhile
loop and modifying the loop variable based on certain conditions, you can control when to restart the loop. -
What is the difference between
for
andwhile
loops in restarting?
Afor
loop iterates over a sequence, while awhile
loop continues until a condition is false, allowing for more dynamic control over the loop’s execution. -
Is using a function to restart a loop efficient?
Yes, encapsulating the loop in a function can improve code readability and maintainability, especially for complex logic. -
Can I restart nested loops in Python?
Yes, you can use similar techniques, but you may need to manage the flow carefully to ensure the right loop restarts as needed.