How to Replace Character in String at Index in Python
- Use String Slicing to Replace a Character in a String at a Certain Index in Python
- Use a List to Replace a Character in a String at a Certain Index in Python
- Replace More Than One Character at Different Indices With the Same Character in All the Cases
- Replace More Than One Character at Different Indices With Different Characters in All the Cases
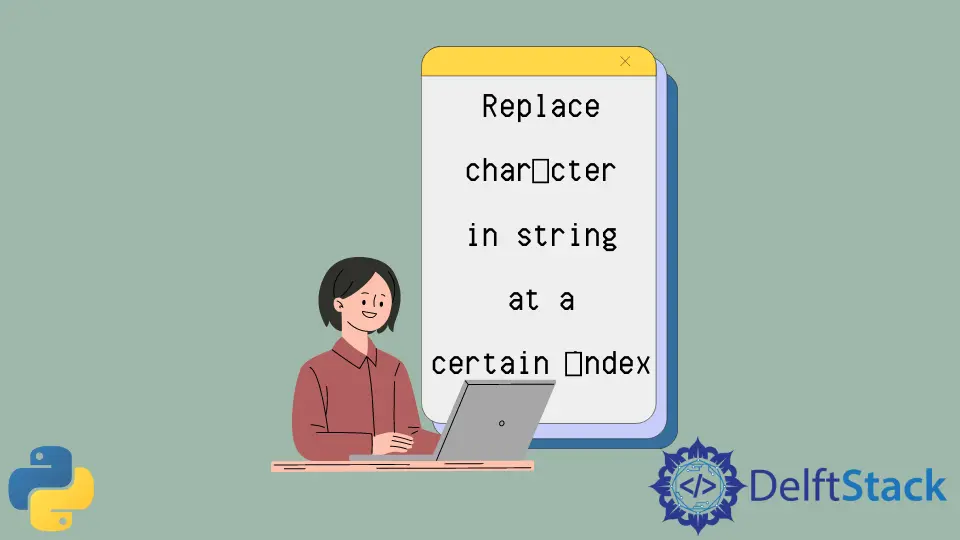
As we all know, strings in Python are immutable, so there is no possible way of directly making changes to the string during the runtime of the Python code. However, there is a need for doing this in some cases, and it can be done indirectly in different ways.
This tutorial demonstrates how to replace a character in a string at a specific index in Python.
Use String Slicing to Replace a Character in a String at a Certain Index in Python
List slicing is an efficient way to solve some of the problems encountered during the coding process. The colon (:
) operator is utilized for the list slicing process. The details or parameters such as start
, finish
, and step
can be specified with the help of the colon operator.
The following code uses string slicing to replace a character in a string at a certain index in Python.
stra = "Meatloaf"
posn = 5
nc = "x"
stra = string[:posn] + nc + string[posn + 1 :]
print(stra)
The above code provides the following output:
Meatlxaf
Explanation:
- To replace a single character at the specified position in the given string, three sections are made by splitting the given string.
- The middle section contains only the character that needs to be replaced. The first and last sections contain the characters before and after the selected character, respectively.
- All three sections are then joined together using the simple
+
operator. However, instead of the middle section containing the selected character, the character that needs to be replaced is joined in place of it.
Use a List to Replace a Character in a String at a Certain Index in Python
A list is one of the four built-in datatypes that Python provides, and it is utilized to stock several items in a single variable. Lists are ordered, changeable, and have a definite count.
In this method, the given string is first converted into a list. After that, the old character is replaced by the new character at the specified index. Finally, the list items are converted to a string using the join()
function.
The following code uses a List to replace a character in a string at a certain index in Python.
stra = "Meatloaf"
posn = 6
nc = "x"
tmp = list(stra)
tmp[posn] = nc
stra = "".join(tmp)
print(stra)
The above code provides the following output:
Meatloxf
These two were the methods that can be utilized to deal with a single character in a string. Moving on, we will now focus on replacing characters at multiple specified indices.
Replace More Than One Character at Different Indices With the Same Character in All the Cases
Here, we will make use of Lists. This method is utilized when there are a few indices, and all the characters at these multiple indices need to be replaced by a single character.
For this to work, all the indices in the given list would need to be iterated, and slicing can be implemented for replacing each found index that requires a character change.
The following code uses list slicing for when we need to replace characters at many indices with a single character.
stra = "Meatloaf"
loi = [2, 4, 6]
nc = "x"
res = ""
for i in loi:
stra = stra[:i] + nc + stra[i + 1 :]
print(stra)
The above code provides the following output:
Mextxoxf
Replace More Than One Character at Different Indices With Different Characters in All the Cases
In this case, the same character does not need to be replaced in all the indices.
For this purpose, we will make use of a dictionary instead of a list. A dictionary is much similar to a hashmap
and stores the entered data in the form of key:value
pairs.
In order to replace all the characters given in the selected indices with their respective replacement, iteration all over the created dictionary’s key:value
pairs.
The following code replaces characters at multiple indices with their respective replacement.
stra = "Meatloaf"
cr = {1: "x", 3: "c", 5: "w"}
res = ""
for index, replacement in cr.items():
stra = stra[:index] + cr[index] + stra[index + 1 :]
print(stra)
The above code provides the following output:
Mextcowf
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn