How to Remove Leading Zeros in Python String
- Use Iteration Statements to Remove Leading Zeros in a String in Python
-
Use the
lstrip()
Function Along With List Comprehension to Remove Leading Zeros in a String in Python -
Use the
int()
andstr()
Functions to Remove Leading Zeros in a String in Python - Use the Regular Expressions to Remove Leading Zeros in a String in Python
- Conclusion
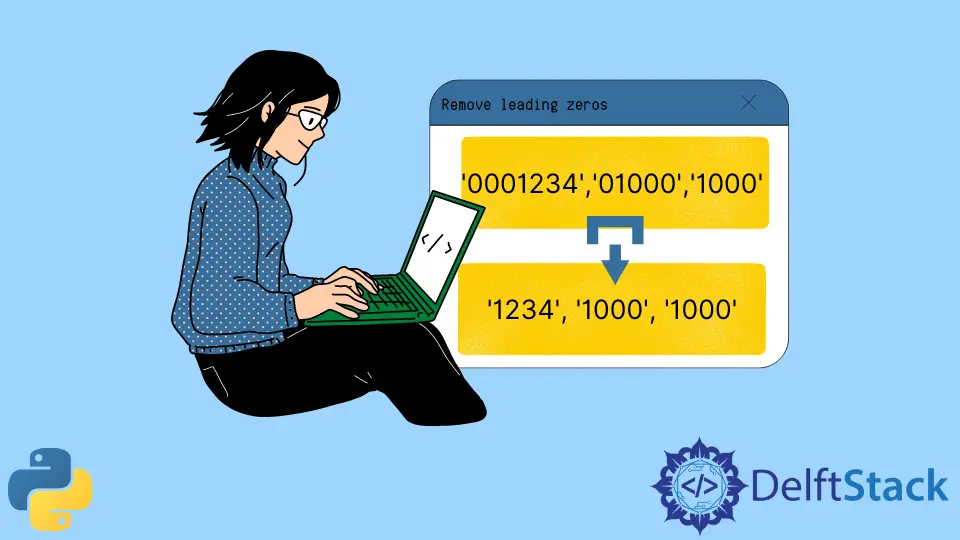
The removal of leading or trailing zeros is essential when data processing is performed and the data has to be passed forward. A stray 0
might get attached to the string while it is transferred from one place to another, and it is recommended to remove it as it is unnecessary and inconvenient.
This tutorial demonstrates the several ways available to remove leading zeros in a string in Python.
Use Iteration Statements to Remove Leading Zeros in a String in Python
The simplest and most basic way to remove leading zeros in a string in Python is to remove them using iteration statements manually. Here, we manually create a code using a for
loop along with the while
loop to make a program that removes leading zeros in a string in Python.
Example Code:
A = [
"0001234",
"01000",
"1000",
]
removeleading = []
for x in A:
while x[0] == "0":
x = x[1:]
removeleading.append(x)
print(removeleading)
Output:
['1234', '1000', '1000']
In the code, we have a list A
containing three strings. We are iterating through each string in the list using a for
loop.
Within the loop, we use a while
loop to continuously remove leading zeros from the current string(x
) as long as its first character is '0'
. This is achieved by updating the string to exclude its first character in each iteration.
Then, the modified strings, without leading zeros, are then appended to the removeleading
list. Finally, the resulting list is printed, and the output will be a list containing the strings ['1234', '1000', '1000']
, each with leading zeros removed.
Use the lstrip()
Function Along With List Comprehension to Remove Leading Zeros in a String in Python
The lstrip()
can be utilized to remove the leading characters of the string if they exist. By default, a space
is the leading character to remove in the string.
List comprehension is a relatively shorter and very graceful way to create lists that are to be formed based on given values of an already existing list. We can combine these two things and use them in our favor.
Example Code:
A = [
"0001234",
"01000",
"1000",
]
res = [ele.lstrip("0") for ele in A]
print(str(res))
Output:
['1234', '1000', '1000']
In this code, we have a list A
containing three strings. We use a list comprehension to iterate through each string in A
and apply the lstrip("0")
method to remove leading zeros.
Then, the resulting modified strings are stored in the res
list. Finally, we convert the list to a string using str(res)
and print the result, and the output will be a list containing the strings ['1234', '1000', '1000']
, each with leading zeros removed.
Use the int()
and str()
Functions to Remove Leading Zeros in a String in Python
The int()
function is used to convert a specified value (usually a string or a number) to an integer. The str()
function is used to convert a specified value to a string.
Now, combining these functions, you can use int()
to convert a string containing leading zeros into an integer and then use str()
to convert it back to a string without leading zeros, effectively removing them.
Example Code:
A = ["0001234", "01000", "1000"]
result_int_str = [str(int(s)) for s in A]
print(result_int_str)
Output:
['1234', '1000', '1000']
In this code, we have a list A
with three strings representing numeric values, some of which have leading zeros. We use a list comprehension to iterate through each string in A
.
Within the comprehension, we convert each string to an integer using int(s)
, effectively removing leading zeros, and then immediately convert it back to a string using str()
. The resulting list, named result_int_str
, contains the modified strings with leading zeros removed.
Finally, we print the list, and the output of this code will be a list containing the strings ['1234', '1000', '1000']
, each with leading zeros removed.
Use the Regular Expressions to Remove Leading Zeros in a String in Python
A regular expression, often abbreviated as regex
or regexp
, is a series of characters that creates a pattern for searching. It’s a powerful tool used in string manipulation and pattern matching within text.
We can use regular expressions (re
module) to eliminate leading zeros from a string in Python. The regular expression r'^0+'
is specifically designed to find and match one or more leading zeros at the beginning of a string.
Example Code:
import re
A = ["0001234", "01000", "1000"]
result_regex = [re.sub(r"^0+", "", s) for s in A]
print(result_regex)
Output:
['1234', '1000', '1000']
In this code, we utilize the re
module for regular expressions in Python. We have a list A
containing three strings, each representing a numeric value with potential leading zeros.
Using list comprehension, we apply the re.sub(r'^0+', '', s)
function to each string in A
. This regular expression (r'^0+'
) targets and replaces one or more leading zeros with an empty string.
The resulting list, named result_regex
, consists of strings with leading zeros removed. Finally, we print this modified list, and the output will be a list containing the strings ['1234', '1000', '1000']
, each with leading zeros removed.
Conclusion
The article highlights the significance of removing leading or trailing zeros during data processing to ensure data integrity and convenience. Stray zeros in strings can be inconvenient and unnecessary.
The tutorial explores various methods to remove leading zeros in Python, presenting iterative approaches using loops, the lstrip()
function combined with list comprehension, and leveraging the int()
and str()
functions for conversion. Additionally, regular expressions (re
module) are introduced as a powerful tool for pattern matching, demonstrated through the use of the regular expression r'^0+'
to efficiently remove leading zeros from strings.
The presented examples showcase concise and effective solutions for handling leading zeros in Python strings.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn