How to Pad String With Spaces in Python
-
Use the
ljust()
Function to Pad the Right End of a String With Spaces in Python -
Use the
rjust()
Function to Pad the Left End of a String With Spaces in Python -
Use the
center()
Function to Pad Both Ends of a String With Spaces in Python -
Use String Formatting With the Modulus
(%)
Operator to Pad a String With Spaces in Python -
Use String Formatting With the
str.format()
Function to Pad a String With Spaces in Python -
Use String Formatting With
f-strings
to Pad a String With Spaces in Python - Conclusion
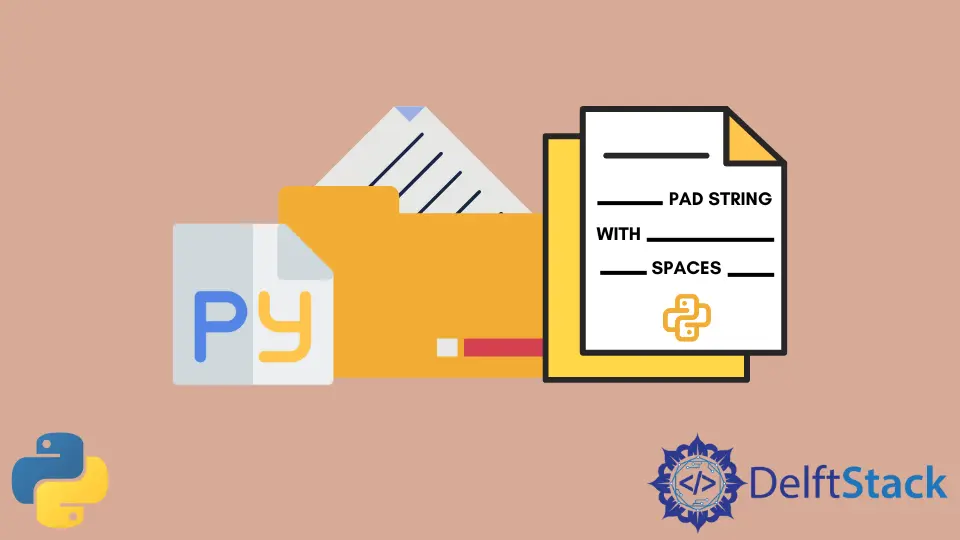
String padding is a valuable tool for string formatting and aligning text when printing. It can be applied to one side of a string, both sides equally, or even using different characters to meet specific formatting requirements.
This article will discuss the different string padding methods, including the ljust()
, rjust()
, center()
functions, the modulus operator %
, str.format()
, and modern f-strings
. Let’s look into each method to understand how string padding works in Python.
Use the ljust()
Function to Pad the Right End of a String With Spaces in Python
The ljust()
function in Python offers a straightforward way to add right-padding to a string. It ensures that the string occupies a specified width by adding spaces (or any specified character) to its right side.
Syntax:
string.ljust(width, fillchar)
Parameters:
string
: The original string that you want to pad.width
: The total width that the padded string should occupy.fillchar
(optional): The character used for padding. The default is a space character.
The following code uses the ljust()
function to pad the right end of a string with spaces in Python.
a = "I am legend"
print(a.ljust(15))
In this code, we have a string variable named a
with the value "I am legend"
. We use the ljust()
function to modify this string and the ljust()
function is applied to the string a
and takes two parameters.
The first parameter, 15
, specifies the total width the string should occupy after padding. The second parameter, which is optional, indicates the character to be used for padding.
In this case, since we haven’t provided the second parameter, the default character used for padding is a space. So, when we run this code, it pads the string a
with spaces on the right side to make it a total of 15 characters wide.
Output:
I am legend
As a result, the output of this code will be I am legend
followed by enough spaces to fill the remaining characters to the right, making the total length of the output string equal to 15 characters.
Use the rjust()
Function to Pad the Left End of a String With Spaces in Python
The rjust()
function is similar to ljust()
but adds left padding to a string, ensuring that it occupies the specified width. This function is particularly helpful for intuitive sorting, such as naming files that start with numbers.
Syntax:
string.rjust(width, fillchar)
Parameters:
string
: The original string to be padded.width
: The desired total width for the padded string.fillchar
(optional): The character used for padding. The default is a space.
The following code uses the rjust()
function to pad the left end of a string with spaces in Python.
a = "I am legend"
print(a.rjust(15, " "))
In this code, we start with a string variable a
containing the text "I am legend"
. We use the rjust()
function to manipulate this string, and we provide two parameters.
The first parameter, 15
in this case, specifies the total width that we want the string to occupy after padding. The second parameter, " "
(a space), is optional and denotes the character we want to use for padding.
Since we’ve explicitly provided " "
as the second parameter, it means we want to pad the string with spaces. Consequently, when we run this code, it pads the string a
with spaces on the left side, ensuring that the total length of the output string is 15 characters.
Output:
I am legend
The output prints the string I am legend
with spaces added to its left, making it a total of 15 characters wide.
Use the center()
Function to Pad Both Ends of a String With Spaces in Python
The center()
function allows us to pad a string with equal amounts of characters on both ends, centering the text within a specified width.
Syntax:
string.center(width, fillchar)
Parameters:
string
: The original string to be padded.width
: The total width of the padded string.fillchar
(optional): The padding character (default is a space).
The following code uses the center()
function to pad both ends of a string with spaces in Python.
a = "I am legend"
print(a.center(20))
In this code, we start with a string variable a
containing the text "I am legend"
. We used the center()
function to modify this string and provided two parameters.
The first parameter, 20
in this instance, specifies the total width that we want the string to occupy after padding. The second parameter is optional and typically indicates the character to use for padding, but in our code, we haven’t provided it, so it defaults to using a space for padding.
Consequently, when we execute this code, it centers the string a
within a total width of 20 characters. To achieve this, it adds an equal number of spaces on both sides of the string "I am legend"
.
Output:
I am legend
The output of this code will be the string I am legend
surrounded by spaces on both sides, making it a total of 20 characters wide and perfectly centered.
Use String Formatting With the Modulus (%)
Operator to Pad a String With Spaces in Python
The modulus operator(%
) is one of the oldest methods for string formatting in Python. It enables you to create placeholders and replace them with the values you need.
The %
sign and a letter representing the conversion type are marked as a placeholder for the variable. Being the oldest of the available ways to implement string formatting, this operator works on almost all versions of Python.
Syntax:
"%widths" % (value)
Parameters:
width
: The total width for the output string.value
: The value to be inserted into the placeholder.
Code Example - Left Padding:
The following code uses string formatting with the modulus (%
) operator to pad a string with spaces in Python.
print("%15s" % ("I am legend"))
In this code, we utilized Python’s string formatting technique. We define a format string "%15s"
, which acts as a template for the string’s formatting.
The %
signifies a placeholder for the string, and 15
sets the desired total width. By using the %
operator, we insert the string "I am legend"
into the placeholder.
Output:
I am legend
The output of this code will be I am legend
with enough spaces added to its left to make it a total of 15 characters wide. This string will be left-aligned within the 15-character width.
Code Example - Right Padding:
In the program above, we do the left padding to the string. We can similarly do the right padding with the following code.
print("%-15s" % ("I am legend"))
Output:
I am legend
Here, the only drawback is that it can only implement padding on the left end or the right end of a string, but not both ends at once. Moreover, using this method affects the readability of the program.
Use String Formatting With the str.format()
Function to Pad a String With Spaces in Python
Another way to implement string formatting in Python is by using the str.format()
function. This process makes the curly {}
brackets mark the places in the print
statement where the variables need to be substituted.
The str.format()
was first introduced in Python 2.6 and can be used from Python 2.6 to Python 3.5. It uses placeholders marked by curly braces {}
for variable substitution.
Syntax:
"{}{:width}".format(value, value)
Parameters:
width
: The width for the output string.value
: The value to be inserted into the placeholder.
Code Example - Right Padding:
The following code uses string formatting with the str.format()
function to pad a string with spaces in Python.
print("{:15}".format("I am legend"))
In this code, we use Python’s string formatting method. We create a format string "{:15}"
where the :
indicates a placeholder for the string, and 15
specifies the desired width.
We apply the format method to insert "I am legend"
into this placeholder. When executed, the code pads the string with spaces on the right side, ensuring the output is 15 characters wide.
Output:
I am legend
The result is I am legend
followed by spaces to reach the specified width.
Code Example - Left Padding:
The code above adds the right padding to the given string. To add the left padding to the given string, we can make minor tweaks to the program above and use the following program.
print("{:>15}".format("I am legend"))
Output:
I am legend
The str.format()
function can also pad the string on both sides at once. Then, all you have to do is add the ^
sign to the placeholder after the colon sign.
The following code uses the str.format()
function to pad both ends of a string with spaces in Python.
print("{:^15}".format("I am legend"))
Output:
I am legend
Use String Formatting With f-strings
to Pad a String With Spaces in Python
Introduced in Python 3.6, f-strings
are a modern and efficient method for string formatting. They offer concise and readable string padding options.
It is more efficient than its other two peers, the %
sign and str.format()
, as it is faster and easier to understand. It also helps in implementing string formatting in Python at a faster rate than the other two methods.
Syntax:
f'{"text" :{padding}^{width}}'
Parameters:
width
: The width of the output string.padding
: The padding character.^
(optional): For center alignment.
Code Example - Right Padding:
The following code uses string formatting with f-strings
to pad the right end of a string with spaces in Python.
width = 15
padding = " "
print(f'{"I am legend" :{padding}<{width}}')
In this code, we set the width
to 15
for the desired output width and specify a padding
character as a space (" "
). We use an f-string
with formatting, enclosing "I am legend"
in curly braces.
After a colon (:
), we specify the padding character, {padding}<
for left alignment, and the {width}
. When executed, the code pads I am legend
with spaces on the right to achieve a 15-character width.
Output:
I am legend
The output will display I am legend
followed by the necessary spaces to meet the specified width, ensuring the left alignment as per our formatting instructions.
Code Example - Left Padding:
To add the left padding to the given string, we can make small tweaks to the code above and use the following program. We changed the padding character <{width}
to >{width}
.
width = 15
padding = " "
print(f'{"I am legend" :{padding}>{width}}')
Output:
I am legend
Code Example - Center Alignment:
Similar to the case of the str.format()
function, we can use the ^
sign between the padding and the width specified in the placeholder to pad both ends of a string with spaces in Python.
The ^
character signifies center alignment.
width = 15
padding = " "
print(f'{"I am legend" :{padding}^{width}}')
Output:
I am legend
When it comes to using string formatting for achieving the purpose of padding the string, the use of f-strings
is recommended the most among the ones we mentioned as it is more concise, readable, and, hence, less prone to errors.
Conclusion
In conclusion, string padding is a crucial aspect of string formatting and alignment in Python. This comprehensive guide explored various methods to pad strings with spaces, including ljust()
, rjust()
, center()
, the modulus operator %
, str.format()
, and modern f-strings
and among these methods, f-strings
stand out as a concise and readable choice for efficient string padding in Python.
Whether we need to align text in tabular data, format output, or improve the visual presentation of strings, understanding string padding techniques is essential for effective Python programming. By choosing the right method for our specific needs, we can achieve precise string formatting and alignment with ease.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn