Python NULL
-
Check if the Given Variable Is
None
in Python -
Check if the Variable Has a
None
Type -
Assign a
NULL
Value to a Pointer in Python
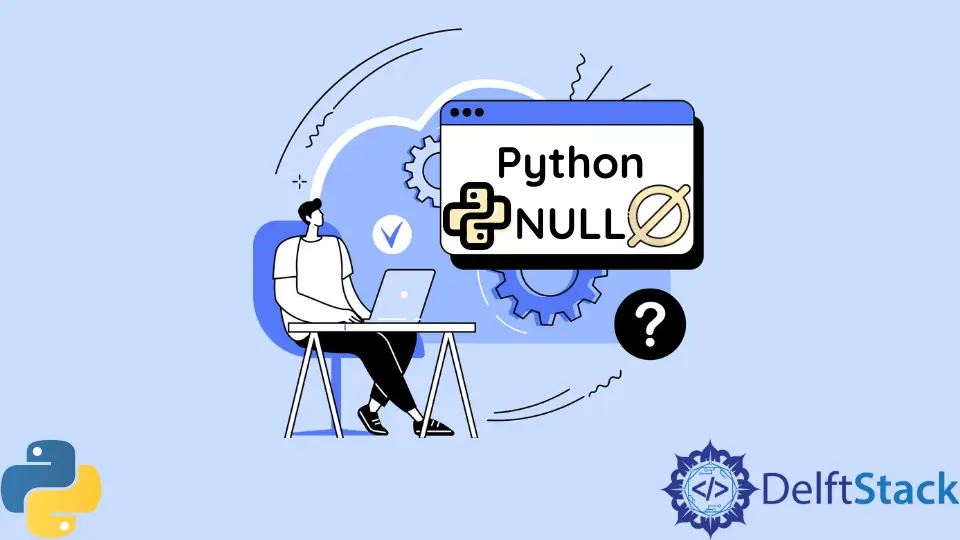
NULL
is a keyword that is used in many programming languages. Java or C being a few examples of these programming languages. If you have ever come across these languages, there is a good chance that you might have heard about the NULL
keyword. In most languages, it represents a pointer that points to nothing, marks parameters that are yet unknown, or denotes that a given particular variable is empty.
This tutorial discusses the NULL
keyword in Python.
When it comes to the NULL
keyword, Python is different from the other programming languages. In most programming languages, the value of NULL
is 0, while it is different in Python.
In Python, the NULL
objects and variables are defined using the None
keyword. The None
keyword does not have a defined value 0
or anything else. The None
keyword is both a NoneType
data type and an object.
Check if the Given Variable Is None
in Python
The keywords is
and ==
can be used to check the None
keyword.
The following code uses the is
keyword to check the NULL
object in Python:
X = None
if X is None:
print("X is None")
else:
print("X is not None")
The above code provides the following output:
X is None
Code Explanation:
- In the above code, the variable
X
is initialized with theNone
keyword. - Next, we implement the
if...else
conditional statement. If the variableX
isNone
, it checks whether the given variableX
has a null value. - If the statement turns out to be true, the statement
print('X is None')
is displayed on the screen. If the statement turns out to be false, the statementprint('X is not None')
is then printed.
In the above code, the is
operator is utilized for evaluating the variable. We can also use the ==
operator in place of the is
operator.
Similarly, the following code uses the ==
operator to check NULL
object in Python.
X = None
if X == None:
print("X is None")
else:
print("X is not None")
The above code provides the following output:
X is None
Check if the Variable Has a None
Type
In addition to checking the NULL
object or None
keyword in Python, the data type of the None
keyword can also be checked as follows:
X = None
print(type(X))
The above code provides the following output:
<class 'NoneType'>
Code Explanation:
- First, the
None
value is assigned to the variableX
. - The
type()
, used within theprint()
method, returns the data type of the given variable taken as an argument.
Assign a NULL
Value to a Pointer in Python
In Python, all the objects are implemented with the help of references, which leads to the fact that there is no distinction between pointers to objects and objects.
The following code assigns a NULL
value to a pointer in Python.
class Node1:
def __init__(self):
self.val = 0
self.right = None
self.left = None
The important thing to note here is that unlike how NULL
works in C, Python’s None
keyword does not mean a pointer that points to nothing, but rather it simply is an instance of the NoneType
class.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn