How to Fix TypeError: Not Enough Arguments for Format String Error in Python
- String Formatting in Python
-
Python
TypeError: Not Enough Arguments for Format String
-
Fix the
TypeError: Not Enough Arguments for Format String
- Conclusion
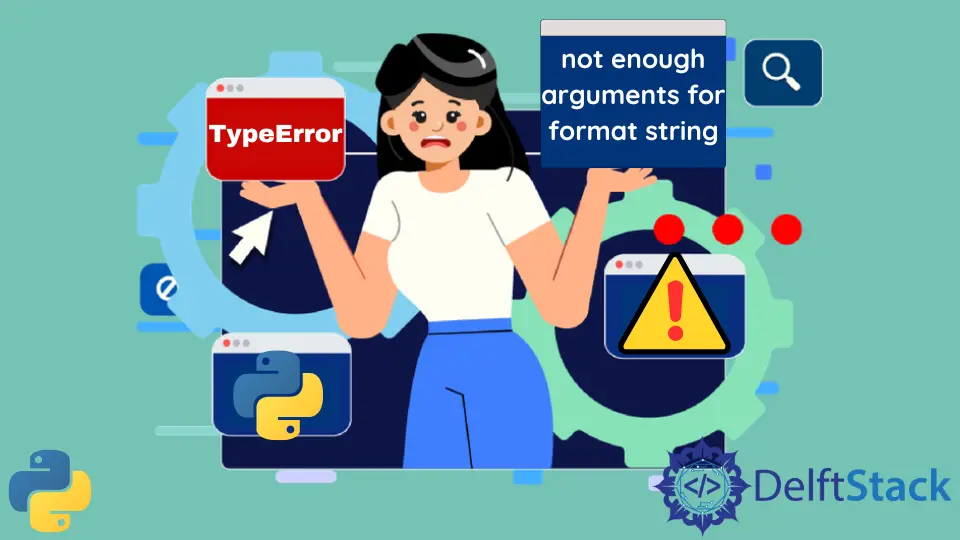
In Python, string formatting is a powerful feature that allows developers to control the appearance of their output. However, it’s not uncommon to encounter errors, and one that often perplexes newcomers is the not enough arguments for format string
error.
This error is indicative of a mismatch between the placeholders in the string and the values provided for substitution.
String Formatting in Python
In Python, string formatting is commonly achieved using the %
operator. This operator, when used with strings, allows the incorporation of placeholders.
These placeholders are denoted by %s
, %d
, %f
, or other format specifiers, depending on the type of data to be inserted.
Consider the following example:
name = "Jake"
age = 32
sentence = "My name is %s, and I am %d years old." % (name, age)
print(sentence)
Output:
My name is Jake, and I am 30 years old.
Here, name
is a string variable holding the value Jake, and age
is an integer variable with the value 30
. The sentence
variable contains a string with two placeholders: %s
for a string and %d
for an integer.
The values (name, age)
are provided in a tuple to replace the respective placeholders, ensuring a match between the number of placeholders and values.
Python TypeError: Not Enough Arguments for Format String
The not enough arguments for format string
error occurs when the total number of placeholders in the string does not match the total number of values provided for substitution.
Let’s look at a common mistake:
name = "Jake"
age = 30
sentence = "My name is %s, and I am %d years old." % name
print(sentence)
Output:
TypeError: not enough arguments for format string
In this case, there are two placeholders (%s
and %d
) in the string, but only one value (name
) is provided. This mismatch results in a TypeError
.
A TypeError
is a built-in Python exception that occurs when an operation or function is applied to an object of an inappropriate type.
In the context of the not enough arguments for format string
error, the TypeError
arises because the %
operator expects a tuple as its right operand when used for string formatting.
However, providing a single value instead of tuple results in a type mismatch, triggering the TypeError
.
Fix the TypeError: Not Enough Arguments for Format String
When encountering the not enough arguments for format string
error in Python, it’s essential to understand the various approaches for resolution. Let’s explore in more detail how to fix this error and discuss additional relevant details for each method.
Use Tuples for Multiple Values
To avoid the error, ensure that the values for substitution are provided in the correct format, typically as a tuple.
name = "Jake"
age = 30
sentence = "My name is %s, and I am %d years old." % (name, age)
print(sentence)
Output:
My name is Jake, and I am 30 years old.
Here, the values (name, age)
are enclosed in parentheses, forming a tuple. This tuple is then used to replace the corresponding placeholders %s
and %d
in the string.
Using tuples not only resolves the error but also provides a clear and concise way to organize multiple values for string formatting.
Switch to the format()
Method
An alternative to the %
operator is the format()
method. This method allows for more flexibility in formatting and avoids the pitfalls of the not enough arguments for format string
error.
Consider the following example:
name = "Jake"
age = 30
sentence = "My first name is {}, and I am {} years old.".format(name, age)
print(sentence)
Output:
My first name is Jake, and I am 30 years old.
In this example, the format()
method is used, and the values (name, age)
are passed as arguments. The {}
placeholders are automatically replaced in the order they appear in the string.
This method not only resolves the error but also allows for more intricate formatting options.
Use F-Strings (Python 3.6 and above)
F-strings provide a concise and readable way to format strings in Python 3.6 and later versions.
Consider the following example:
name = "Jake"
age = 30
sentence = f"My first name is {name}, and I am {age} years old."
print(sentence)
Output:
My first name is Jake, and I am 30 years old.
F-strings are used, and values are directly inserted within curly braces {}
. This method enhances readability and is available in Python 3.6 and later versions.
Conclusion
Understanding the not enough arguments for format string
error is crucial for Python developers working with string formatting. By ensuring a proper match between placeholders and values and exploring alternative formatting methods like format()
and f-strings, developers can enhance the robustness and clarity of their code.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn