The NetworkX Package in Python
- Install NetworkX Package in Python
- Add Nodes to a Graph Using NetworkX Package in Python
- Add Edges to a Graph Using NetworkX Package in Python
- Remove Nodes from a Graph Using NetworkX Package in Python
- Remove Edges from a Graph Using NetworkX Package in Python
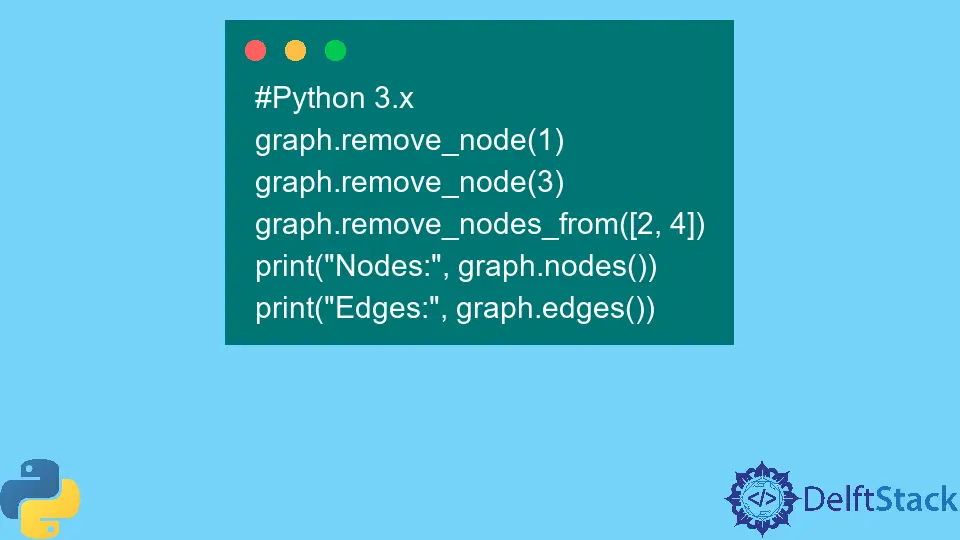
This tutorial will introduce the uses of the Python NetworkX package to study complex networks.
NetworkX package includes built-in methods by which we can create, manipulate, and analyze complex networks represented as a graph.
This library provides many features such as graph data structure, creating and deleting nodes, adding links between nodes, and many other algorithms for graphs.
Install NetworkX Package in Python
To use the NetworkX package in the Python program, we should install it first using the following command.
#Python 3.x
pip install networkx
Add Nodes to a Graph Using NetworkX Package in Python
To add nodes to a graph, first, we must import the NetworkX library and create an empty graph by calling the Graph()
method. When adding nodes to the graph, we can add them either one by one or collectively.
We will use the add_node()
method to add nodes one by one. To add a list of nodes, we will use the add_nodes_from()
method that takes an iterable object.
A node can be immutable objects such as int, float, String, boolean, tuple, etc. If we add duplicate nodes in a graph, they will be ignored.
We can print nodes to a graph using the code below.
# Python 3.x
import networkx as n
graph = n.Graph()
graph.add_node(1)
graph.add_node(2)
graph.add_node(3)
graph.add_node(4)
graph.add_nodes_from([5, 6, 7])
print("Nodes:", graph.nodes())
Output:
#Python 3.x
Nodes: [1, 2, 3, 4, 5, 6, 7]
Add Edges to a Graph Using NetworkX Package in Python
By joining two or more nodes, we can add an edge to a graph. We can either add one edge or multiple edges at a time.
We will use the add_edge()
method to add a single edge. And to add multiple edges at a time, we will use the add_edges_from()
method.
The following code is a continuation of the above code.
# Python 3.x
graph.add_edge(1, 2)
graph.add_edge(3, 4)
graph.add_edges_from([(5, 6), (6, 7)])
print("Nodes:", graph.nodes())
print("Edges:", graph.edges())
Output:
#Python 3.x
Nodes: [1, 2, 3, 4, 5, 6, 7]
Edges: [(1, 2), (3, 4), (5, 6), (6, 7)]
In the code above, we added an edge between nodes 1 and 2 and nodes 3 and 4 using add_ edge()
. And we simultaneously added edges between nodes 5 and 6 and nodes 6 and 7 using add_edges_from()
.
Finally, we printed the nodes and edges of the graph.
Remove Nodes from a Graph Using NetworkX Package in Python
We can also remove nodes in a graph in Python. If we delete a node, its associated edge will also be deleted.
To remove a single node from the graph, we will use remove_node()
. And to remove multiple nodes at the same time, we will use remove_nodes_from()
.
We’ll pass some iterable containers to these methods consisting of a list of nodes to delete. When we use these methods, and the respective node doesn’t exist, NetworkX error will be returned.
# Python 3.x
graph.remove_node(1)
graph.remove_node(3)
graph.remove_nodes_from([2, 4])
print("Nodes:", graph.nodes())
print("Edges:", graph.edges())
Output:
#Python 3.x
Nodes: [5, 6, 7]
Edges: [(5, 6), (6, 7)]
In the above code, we deleted nodes 1 and 3 using remove_node()
. And we deleted nodes 2 and 4 using remove_nodes_from()
at the same time.
Thus the edges associated with them are also deleted.
Remove Edges from a Graph Using NetworkX Package in Python
To remove edges of a graph, we will use the remove_edge()
and remove_edges_from()
methods.
If we want to remove a single edge, we must go with remove_edge()
, and if we’re going to remove multiple edges simultaneously, we must use remove_edges_from()
.
# Python 3.x
graph.remove_edge(5, 6)
graph.remove_edges_from([(6, 7), (4, 5)])
print("Nodes:", graph.nodes())
print("Edges:", graph.edges())
Output:
#Python 3.x
Nodes: [5, 6, 7]
Edges: []
The edge between nodes 5 and 6 was first deleted using the remove_edge()
method.
We also want to remove the edges between nodes 6 and 7 and nodes 4 and 5 using the remove_edges_from()
method; however, nodes 5 and 6 were already removed. Thus, the method ignored this one.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn