Python Multiple Constructors
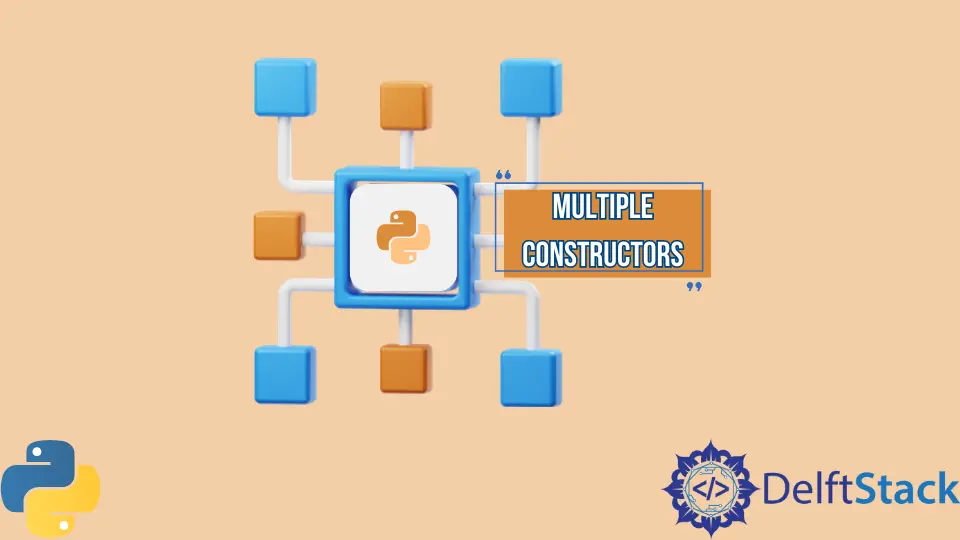
This tutorial will discuss constructors and the method to deal with the need for multiple constructors in Python.
Constructors in Python
In programming languages like Java, C++, and C# programming languages, we have the concept of a constructor function that only runs when an object of the class is initialized. The constructor function is used to initialize all the class attributes. The name of this constructor function is the same as the name of the class. The concept of the constructor function is the same in Python but, the name of the constructor function is __init__()
for all the classes. The constructor in Python always takes one parameter, that is, a pointer to the calling object. The following code snippet shows how we can create a non-parameterized constructor in Python.
class Person:
def __init__(self):
print("Constructor Function")
if __name__ == "__main__":
p1 = Person()
Output:
Constructor Function
We created a non-parameterized constructor for the Person
class and created a Person
class object in the main function. The constructor was called when we created the object with p1 = Person()
.
Multiple Constructors in Python
In Java, C#, and C++ programming languages, there is a concept called multiple constructors where we can create as many constructors for a single class as we desire. Unfortunately, we cannot define multiple constructors for a single class in Python. A general method of getting around this limitation is to use a default parameter constructor. A default parameter constructor is the one that automatically assigns a value to its class attributes if no parameter is passed while creating the class object. The default parameter constructor assigns the specified value to the class attribute if some value is specified during the object creation. The following code snippet shows how to eliminate the need for multiple constructors with the default parameter constructor in Python.
class Person:
def __init__(self, name="Tom"):
self.name = name
def show(self):
print("Name = ", self.name)
if __name__ == "__main__":
p1 = Person()
p2 = Person("Mark")
p1.show()
p2.show()
Output:
Name = Tom
Name = Mark
We created a default parameter constructor in the above code by specifying the default value during the function definition. The constructor of the Person
class takes a parameter name and assigns it to the class parameter name
. The default value of the parameter name is Tom
. We created two objects p1
and p2
of the Person
class in the main function. The object p1
does not specify any value while initialization; the object p2
passes Mark
as a constructor parameter. We then called the show()
function with p1
and p2
. The show()
function displays the value of the name
attribute. When p1
calls the show()
function, the output is the default value Name = Tom
, but when p2
calls the show()
function, the output is Name = Mark
because we specified Mark
while creating the object p2
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn