How to Overload a Constructor in Python
- Using Multiple Arguments to Overload Constructors in Python
-
Use the
@classmethod
Decorators to Overload a Constructor in Python - Using Default Arguments to Overload a Constructor in Python
- Conclusion
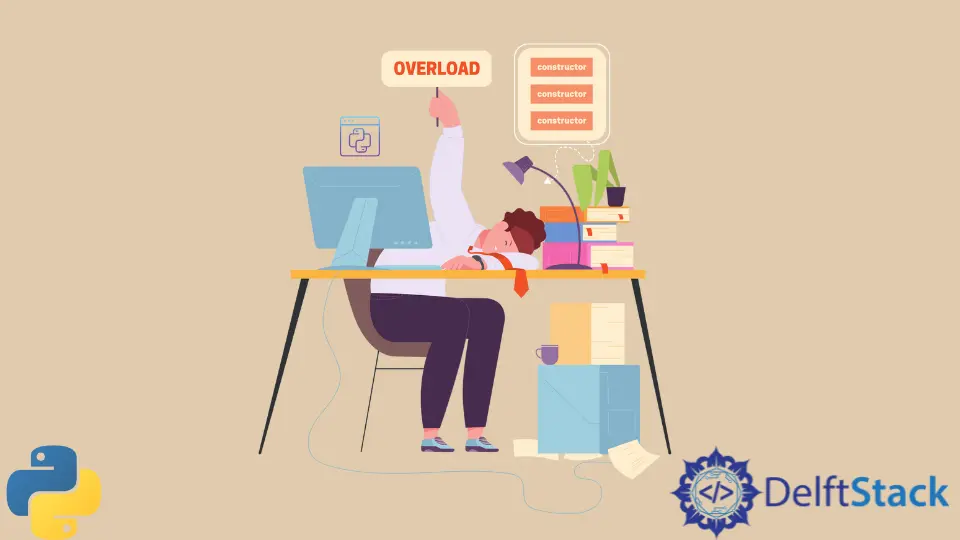
Multiple constructors help in customizing our custom class accordingly to its parameters. While using different parameters, we can trigger different constructors.
Multiple constructors are not directly supported in Python. When multiple constructors are provided in the class, the latest one overrides the previous one. However, there are some alternative ways to overload a constructor in Python.
We will discuss these methods in this article.
Using Multiple Arguments to Overload Constructors in Python
Function overloading refers to having different functions with the same name with different types of parameters. We can overload a constructor by declaring multiple conditions, with every condition based on a different set of arguments.
Example:
class delftstack:
def __init__(self, *args):
if isinstance(args[0], int):
self.ans = args[0]
elif isinstance(args[0], str):
self.ans = "Hello from " + args[0]
s1 = delftstack(1)
print(s1.ans)
s2 = delftstack("Delft")
print(s2.ans)
In this example, we define a class delftstack
with a constructor method that accepts variable arguments *args
. Inside the constructor, we check the type of the first argument passed (args[0]
).
If it’s an integer, we assign it directly to the ans
attribute of the object. If it’s a string, we concatenate it with the string "Hello from "
before assigning it to ans
.
This allows us to create instances of delftstack
with either an integer or a string as the first argument, resulting in different initialization behavior.
Output:
We can also overload a constructor based on the number of arguments provided. This method is similar to the previous example.
Example:
class delftstack:
def __init__(self, *args):
if len(args) > 3:
self.ans = "More than three"
elif len(args) <= 3:
self.ans = "Less than three"
s1 = delftstack(1, 2, 3, 4)
print(s1.ans)
s2 = delftstack(1, 2)
print(s2.ans)
In this example, the class delftstack
defines a constructor method that accepts variable arguments *args
. Inside the constructor, we evaluate the length of the args
tuple.
If the length is greater than 3, we assign the string "More than three"
to the ans
attribute of the object. Otherwise, if the length is less than or equal to 3, we assign the string "Less than three"
.
Consequently, the behavior of object initialization varies based on the number of arguments passed.
Output:
Use the @classmethod
Decorators to Overload a Constructor in Python
The @classmethod
decorator allows the function to be accessible without instantiating a class. Such methods can be accessed by the class itself and via its instances.
When used in overloading, such functions are called factory methods. We can use them to implement the concept of constructor overloading in Python.
Example:
class delftstack(object):
def __init__(self, a):
self.ans = "a"
@classmethod
def first(cls):
return "first"
@classmethod
def second(cls):
return "second"
s1 = delftstack.first()
print(s1)
s2 = delftstack.second()
print(s2)
In this example, the class delftstack
defines a primary constructor __init__
and two class methods, first()
and second()
, decorated with @classmethod
. These class methods serve as alternative constructors.
When delftstack.first()
is called, it returns "first"
, and when delftstack.second()
is called, it returns "second"
. These methods can be used to create instances of the class with specific initialization patterns, providing flexibility in object creation.
Output:
This method is the most pythonic way of overloading a constructor. In the above example, the cls
argument of the factory method refers to the class itself.
Using Default Arguments to Overload a Constructor in Python
Using default parameter values in Python allows us to create constructors that appear to accept different numbers of arguments, effectively achieving constructor overloading. By specifying default values for parameters in the constructor method, we can define multiple initialization patterns within a single constructor.
Example:
class MyClass:
def __init__(self, arg1=0, arg2=0):
self.data = arg1 + arg2
obj1 = MyClass()
obj2 = MyClass(3)
obj3 = MyClass(2, 5)
print(obj1.data)
print(obj2.data)
print(obj3.data)
In the provided code, we define a class MyClass
with a constructor method __init__
that takes two parameters, arg1
and arg2
, with default values of 0. This constructor initializes an attribute data
with the sum of arg1
and arg2
.
We then create three instances of MyClass
- obj1
, obj2
, and obj3
- each with different argument combinations. obj1
is initialized with no arguments, resulting in data
being 0. obj2
is initialized with one argument (3), resulting in data
being 3.
Finally, obj3
is initialized with two arguments (2 and 5), resulting in their sum, 7, being assigned to data
. We then print the values of data
for each object, demonstrating how default parameter values enable constructor overloading and flexible object initialization in Python.
Output:
Conclusion
Constructors are pivotal in object-oriented programming, and Python provides various ways to achieve constructor overloading to customize class initialization. Through this article, we explored multiple methods to overload constructors in Python, including using multiple arguments, @classmethod
decorators, and default parameter values.
Each method offers its unique advantages and can be selected based on specific requirements. While using multiple arguments allows for flexible initialization based on argument types or counts, @classmethod
decorators facilitate the creation of alternative constructors within a class.
Additionally, default parameter values provide a concise way to define multiple initialization patterns within a single constructor.