How to Create a Multi Line String in Python
-
Create a Multi-Line String in Python Using
"""
-
Create a Multi-Line String in Python Using
()
-
Create a Multi-Line String in Python Using
\
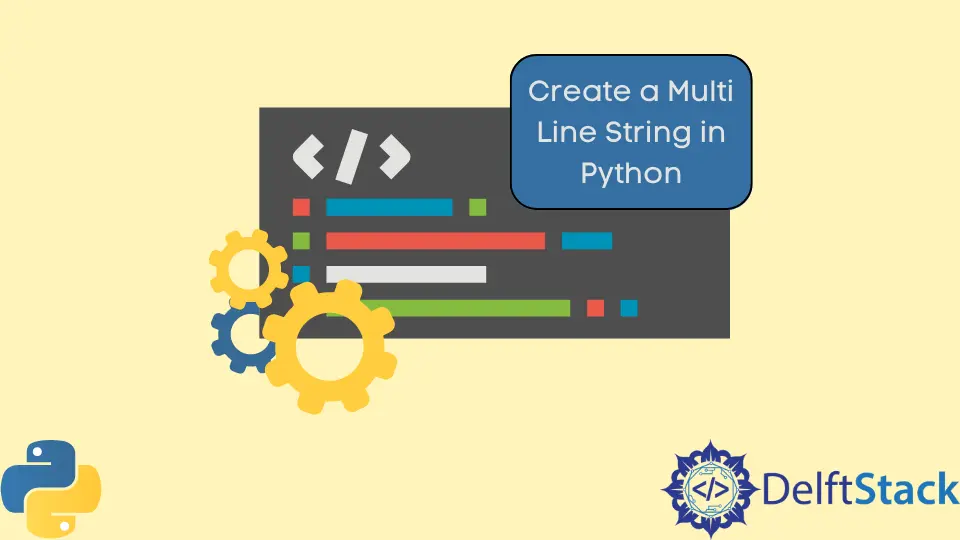
This tutorial will explain multiple ways to create a multi-line string in Python. Multi-line string means a string consisting of multiple lines.
For example:
multi_line_string = "this is line number 1"
"this is line number 2"
"this is line number 3"
"this is line number 4"
All these lines are expected to a string variable - multi_line_string
, but actually, only the first line gets assigned, and the compiler will give an error.
Create a Multi-Line String in Python Using """
One way to create a multi-line string is to use """
at the start and end of the lines. Using triple quotes instead of single or double quotes, we can assign multi-line text to the string. It is the easiest method to copy multiple lines from somewhere and assign them to a string variable without any change.
Example code:
multi_line_string = """this is line number 1
this is line number 2
this is line number 3
this is line number 4"""
print(multi_line_string)
Output:
this is line number 1
this is line number 2
this is line number 3
this is line number 4
Create a Multi-Line String in Python Using ()
In this method, we just put all the text lines in parenthesis ()
to create a multi-line string, while each line is inside the double or single quotes.
It can be useful if we want to create a multi-line string from multiple string variables without concatenating them separately or writing them in a single line and using the +
operator for concatenation.
Example code:
multi_line_string = (
"this is line number 1 "
"this is line number 2 "
"this is line number 3 "
"this is line number 4"
)
print(multi_line_string)
Output:
this is line number 1 this is line number 2 this is line number 3 this is line number 4
Create a Multi-Line String in Python Using \
A multi-line string can also be created by putting backslash \
at the end of each line of multi-line string.
Its function is the same as the parenthesis ()
method. It also just concatenates all the multiple lines and creates a multi-line string.
Example code:
multi_line_string = (
"this is line number 1 "
"this is line number 2 "
"this is line number 3 "
"this is line number 4"
)
print(multi_line_string)
Output:
this is line number 1 this is line number 2 this is line number 3 this is line number 4