Python if-else Shorthand
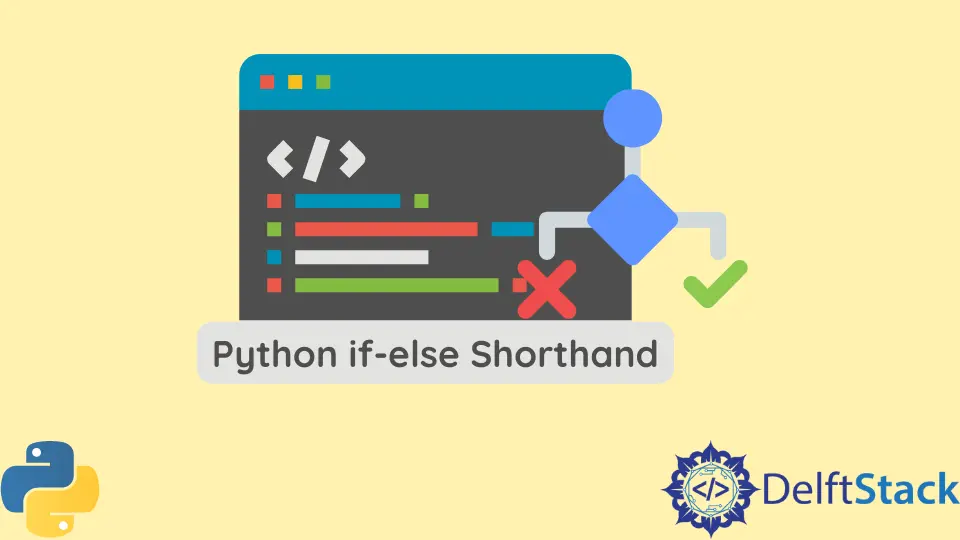
Shorthand notations are often used in programming to make our work easier. Shorthand notations are the methods by which a work can be done more concisely and in less time and energy.
This article will discuss the shorthand notation used in Python as a shortcut for the if-else
statements.
Use the Ternary Operator as if-else
Shorthand in Python
As discussed earlier, a shorthand notation is a way in which a program can be written concisely. There have been many shorthand notations that we have been using so far in Python.
Let us take the example of the assignment operators. The expression a=a+b
becomes a+=b
; similarly, a=a/b
becomes a/=b
, and many more.
Similar to such shorthand notations in Python, we have one more notation known as a ternary operator for the if-else
statement in Python. It was introduced in Python version 2.5 and is continued to exist because of its usefulness.
Since the if-else
statements are used as decision-making statements in any programming language, so are ternary operators. These ternary operators decide whether to execute the first set of statements or the second based on the condition’s truth or falsity.
This ternary operator is used in almost all programming languages, such as Java, C++, etc., in place of the if-else
statement making it easier to use the if
statement. However, Python does not follow the same syntax as the other languages, but the purpose remains the same in all of them.
There are three components in the ternary operator: the condition, the positive value, and the negative value. The condition is the same thing checked in an if
statement; it will decide whether to execute the statements inside if
or else
.
The positive value refers to the statements inside the if
statement, whereas the negative value represents the statements inside the else
statement in Python.
In other languages, the ternary operator is used with the colon and the question mark symbol. However, the if
and the else
keywords are used in Python but with different syntax.
The syntax for the ternary operator is as follows:
(positive value) if (expression/condition) else (negative value)
However, the syntax seems a little confusing, but the processing is as simple as the if/else
statements. Let us understand the ternary operator with the help of the code examples.
print(10) if 3 > 4 else print(15)
print(10) if 5 > 4 else print(15)
Output:
15
10
Therefore, as you can observe in the above code example, the expression 3>4
is checked for validity since it is false. Thus, the statement print(15)
after else
has been executed.
On the other hand, since the expression/condition 5>4
is true. Therefore, the positive statement print(10)
has been executed.
Let us take another example to grasp the ternary operator in Python better.
a = 4
b = 2
c = 2 + a if a == 4 else b
d = 1 + (a if a == 5 else b)
print(c)
print(d)
Output:
6
3
As you can observe in the above code example, in the first statement, the positive value to be evaluated, 2+a
, has been printed since the condition a==4
was true. Had it not been true, the value of b
would have been printed.
Similarly, for the second assignment statement, the condition is evaluated that turns out to be false. Therefore, the value b
will be printed with an addition of 1, and the answer turns out to be 3.
Conclusion
In this article, we’ve learned about the shorthand notation for the if-else
statement in Python, the ternary operator.
The ternary operator comes with a condition/expression, a positive, and a negative value. The condition/expression is checked, and if found true, the positive value is executed; otherwise, the negative statement is executed.